Find As A Function Of If
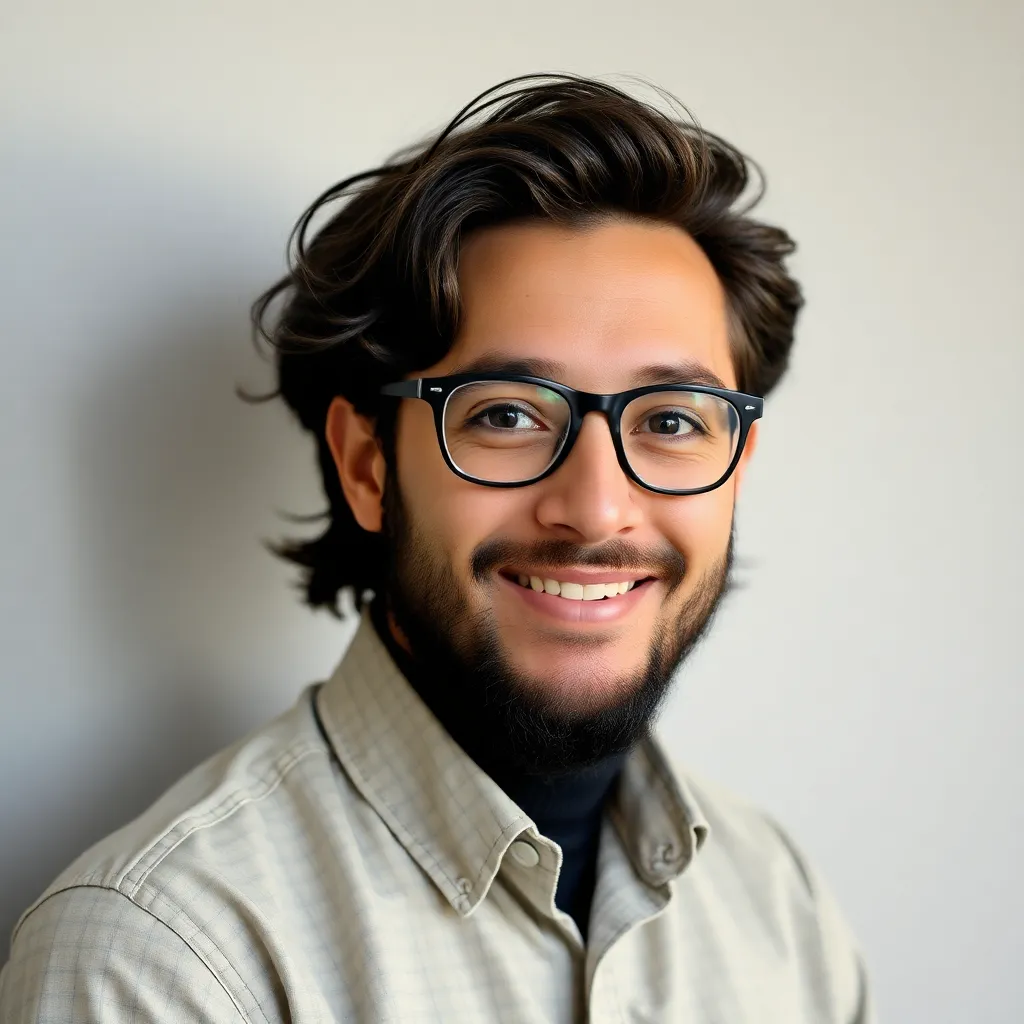
Holbox
Mar 19, 2025 · 5 min read
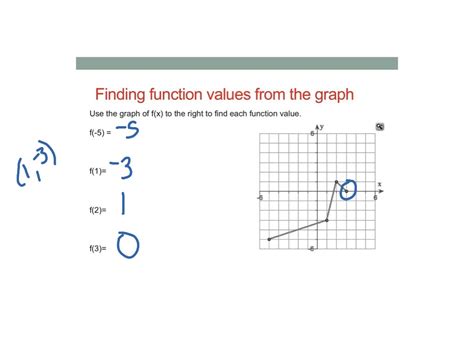
Table of Contents
Find as a Function of IF: Mastering Conditional Logic in Programming
Finding a value based on a condition is a fundamental concept in programming. This core functionality is almost universally implemented using conditional statements, most commonly the if
statement. This comprehensive guide delves into the intricacies of using if
statements to find values, exploring various scenarios, complexities, and best practices to ensure your code is efficient, readable, and robust. We'll cover everything from simple if
statements to nested structures and more advanced techniques for handling conditional logic.
Understanding the Basics: The Simple if
Statement
At its heart, the if
statement allows your program to execute a block of code only when a specific condition is true. The syntax generally follows this pattern:
if (condition) {
// Code to execute if the condition is true
}
Let's illustrate this with a simple example. Suppose we want to find the absolute value of a number. We can use an if
statement to check if the number is negative and, if so, multiply it by -1:
public class FindAbsoluteValue {
public static int findAbsolute(int num) {
if (num < 0) {
return num * -1;
} else {
return num;
}
}
public static void main(String[] args) {
int number = -5;
int absoluteValue = findAbsolute(number);
System.out.println("The absolute value of " + number + " is: " + absoluteValue);
}
}
This Java code demonstrates a basic if-else
structure. The condition num < 0
is evaluated. If it's true, the function returns the negative of the number; otherwise, it returns the number itself.
Expanding Functionality: if-else if-else
Chains
When dealing with multiple conditions, using a chain of if-else if-else
statements provides a structured way to handle various possibilities. Consider a scenario where you need to assign a letter grade based on a numerical score:
def get_letter_grade(score):
if score >= 90:
return 'A'
elif score >= 80:
return 'B'
elif score >= 70:
return 'C'
elif score >= 60:
return 'D'
else:
return 'F'
print(get_letter_grade(85)) # Output: B
This Python function efficiently assigns a letter grade based on the input score. The conditions are checked sequentially, and the first condition that evaluates to true determines the returned grade. The else
block acts as a catch-all for scores below 60.
Nested if
Statements: Handling Complex Logic
Nested if
statements are used when a condition needs to be further evaluated based on the outcome of a previous condition. This approach is crucial for managing more intricate decision-making processes.
Imagine a program that determines eligibility for a loan based on credit score and income:
function isLoanEligible(creditScore, income) {
if (creditScore >= 700) {
if (income >= 50000) {
return true; // Eligible
} else {
return false; // Not eligible due to income
}
} else {
return false; // Not eligible due to credit score
}
}
console.log(isLoanEligible(750, 60000)); // Output: true
console.log(isLoanEligible(650, 80000)); // Output: false
This JavaScript function demonstrates nested if
statements. Eligibility is determined first by checking the credit score. Only if the credit score meets the threshold is the income checked.
The Ternary Operator: A Concise Alternative
For simple conditional assignments, the ternary operator offers a more compact syntax. It's a shorthand way of expressing an if-else
statement in a single line.
Let's revisit the absolute value example using the ternary operator in C#:
public int FindAbsolute(int num) => num < 0 ? num * -1 : num;
This single line of C# code achieves the same functionality as the longer if-else
block in the earlier Java example. The expression num < 0 ? num * -1 : num
evaluates to num * -1
if num
is negative and num
otherwise.
Switch Statements: Handling Multiple Equality Checks
When dealing with many equality checks against a single variable, switch
statements offer a cleaner and often more efficient approach than long if-else if
chains.
Consider a program that determines the day of the week based on a numerical representation (1 for Monday, 2 for Tuesday, etc.):
#include
#include
std::string getDayOfWeek(int day) {
switch (day) {
case 1: return "Monday";
case 2: return "Tuesday";
case 3: return "Wednesday";
case 4: return "Thursday";
case 5: return "Friday";
case 6: return "Saturday";
case 7: return "Sunday";
default: return "Invalid day";
}
}
int main() {
std::cout << getDayOfWeek(3) << std::endl; // Output: Wednesday
return 0;
}
This C++ code uses a switch
statement to efficiently determine the day of the week. The default
case handles invalid input values.
Advanced Techniques: Handling Null Values and Exceptions
In real-world applications, you'll often encounter scenarios involving null values or potential exceptions. Proper error handling is crucial to prevent unexpected crashes and maintain program stability.
Let's consider a function that finds the length of a string, handling the possibility of a null string:
public int getStringLength(String str) {
if (str == null) {
return 0; // or throw an exception: throw new IllegalArgumentException("String cannot be null");
} else {
return str.length();
}
}
This Java function checks for a null string before attempting to access its length. This prevents a NullPointerException
. Alternatively, throwing an exception could be a more appropriate response, depending on the context of your application.
Optimizing Conditional Logic for Performance
Efficient conditional logic is critical for performance, especially in applications handling large datasets or complex calculations. Avoid unnecessary nesting and redundant checks. Consider using more efficient data structures, such as hash tables or maps, for faster lookups when dealing with many conditions.
Best Practices for Writing Readable and Maintainable Code
-
Keep it Simple: Avoid overly complex nested
if
statements. Break down complex logic into smaller, more manageable functions. -
Use Meaningful Variable Names: Choose descriptive names for variables and functions to improve code readability.
-
Add Comments: Explain the purpose and logic of your conditional statements, particularly those that are complex or non-obvious.
-
Follow Consistent Formatting: Maintain consistent indentation and spacing to enhance code readability.
-
Test Thoroughly: Test your conditional logic with various inputs to ensure it behaves as expected and handles edge cases correctly.
Conclusion: Mastering Conditional Logic for Effective Programming
The ability to find a value as a function of an if
statement is fundamental to programming. Mastering conditional logic, from basic if
statements to nested structures and advanced techniques, is essential for building robust and efficient applications. By adhering to best practices and employing appropriate error handling, you can ensure your code is not only functional but also easily understood, maintained, and optimized for performance. The examples provided across various programming languages illustrate the versatility and importance of understanding and effectively utilizing conditional logic in any programming endeavor. Remember, clear, concise, and well-tested code is crucial for building reliable and maintainable software.
Latest Posts
Latest Posts
-
Umatilla Bank And Trust Is Considering Giving
Mar 19, 2025
-
An Ordinary Annuity Is Best Defined As
Mar 19, 2025
-
A Flexible Budget Performance Report Compares
Mar 19, 2025
-
Standard Heat Of Formation For H2o
Mar 19, 2025
-
Complete The Synthesis Below By Selecting Or Drawing The Reagents
Mar 19, 2025
Related Post
Thank you for visiting our website which covers about Find As A Function Of If . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.