8.2.7 Sum Rows In A 2d Array
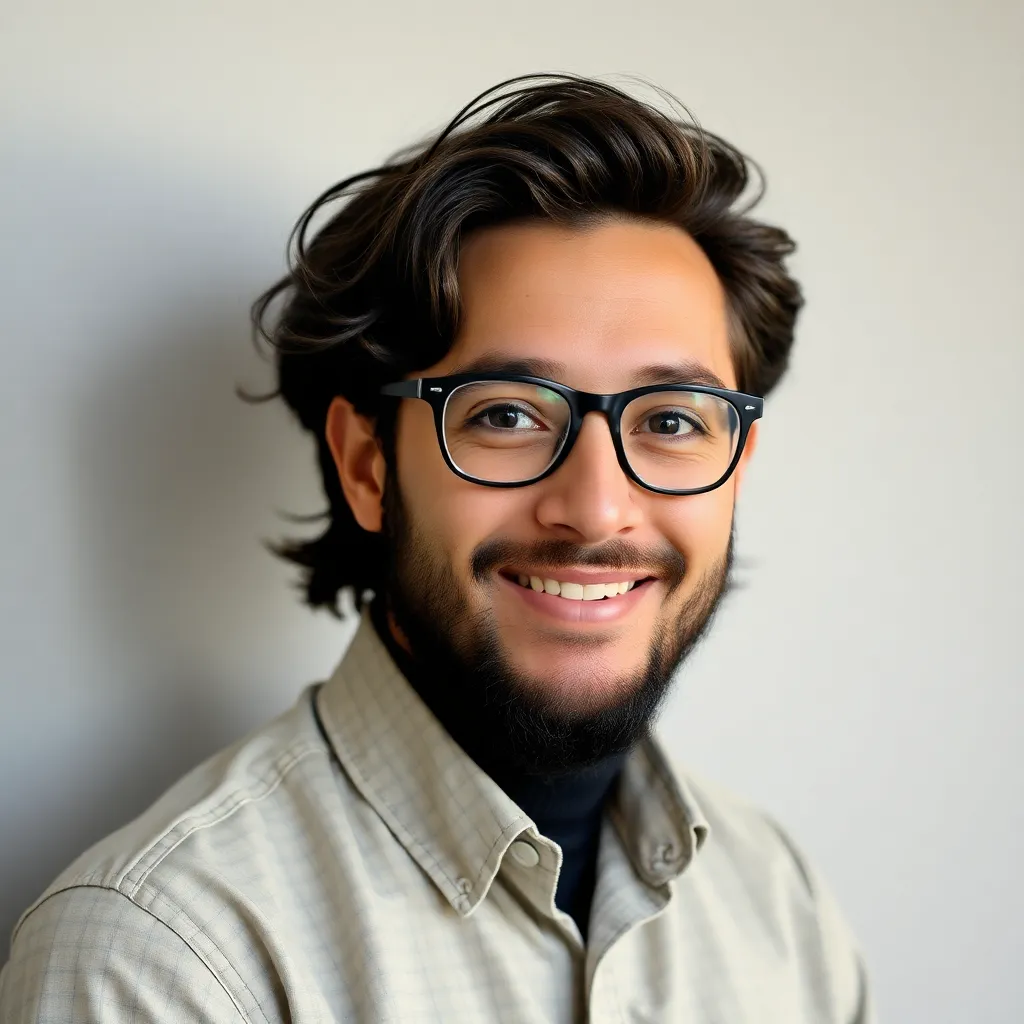
Holbox
Mar 26, 2025 · 6 min read
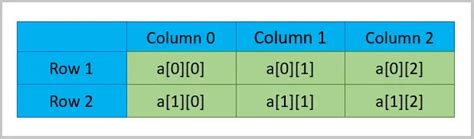
Table of Contents
- 8.2.7 Sum Rows In A 2d Array
- Table of Contents
- 8.2.7 Sum Rows in a 2D Array: A Comprehensive Guide
- Understanding 2D Arrays
- Basic Iterative Approach
- Using NumPy (Python) for Enhanced Performance
- Functional Programming Approach (Python)
- Handling Irregular 2D Arrays
- Choosing the Right Approach
- Advanced Techniques and Considerations
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
8.2.7 Sum Rows in a 2D Array: A Comprehensive Guide
Summing the rows of a two-dimensional (2D) array is a fundamental operation in various programming tasks, ranging from simple data analysis to complex matrix manipulations. This comprehensive guide delves into the intricacies of this operation, exploring multiple approaches, optimizing for efficiency, and providing practical examples in various programming languages. We'll cover everything from basic iterative methods to more advanced techniques, ensuring you gain a solid understanding of how to effectively sum rows in a 2D array.
Understanding 2D Arrays
Before diving into row summation, let's establish a clear understanding of 2D arrays. A 2D array, also known as a matrix, is a data structure that organizes elements in a grid-like format with rows and columns. Each element in the array is accessed using its row and column index. For example, in a 3x4 array (3 rows, 4 columns), the element at the second row and third column would be accessed using indices [1, 2] (assuming zero-based indexing).
The size of a 2D array is defined by the number of rows and columns it contains. Understanding this structure is crucial for efficiently implementing row summation algorithms.
Basic Iterative Approach
The most straightforward method for summing the rows of a 2D array involves using nested loops. The outer loop iterates through each row, while the inner loop iterates through the elements of the current row, summing them up.
Python Example:
def sum_rows_iterative(array_2d):
"""
Sums the rows of a 2D array using nested loops.
Args:
array_2d: A 2D array (list of lists).
Returns:
A list containing the sum of each row. Returns an empty list if the input is invalid.
"""
try:
row_sums = []
for row in array_2d:
row_sum = sum(row) #Utilizing Python's built-in sum function for efficiency
row_sums.append(row_sum)
return row_sums
except TypeError:
return [] #Handle cases where input is not a 2D array
# Example usage
my_array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
row_sums = sum_rows_iterative(my_array)
print(f"Row sums: {row_sums}") # Output: Row sums: [6, 15, 24]
invalid_array = "not an array"
row_sums = sum_rows_iterative(invalid_array)
print(f"Row sums for invalid input: {row_sums}") # Output: Row sums for invalid input: []
This Python example demonstrates a robust function that handles potential TypeError
exceptions, ensuring the code doesn't crash if an invalid input is provided. The use of Python's built-in sum()
function further enhances efficiency.
Java Example:
public class SumRows2DArray {
public static int[] sumRows(int[][] arr) {
int rows = arr.length;
int cols = arr[0].length; // Assumes all rows have the same length
int[] rowSums = new int[rows];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
rowSums[i] += arr[i][j];
}
}
return rowSums;
}
public static void main(String[] args) {
int[][] myArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int[] sums = sumRows(myArray);
System.out.print("Row sums: ");
for (int sum : sums) {
System.out.print(sum + " ");
} // Output: Row sums: 6 15 24
}
}
The Java example showcases a similar iterative approach, ensuring the code is clear and easy to understand. Error handling isn't explicitly included in this example for brevity but should be considered in a production environment.
Using NumPy (Python) for Enhanced Performance
For larger arrays, the iterative approach can become computationally expensive. NumPy, a powerful Python library for numerical computation, offers significantly faster solutions using vectorized operations.
import numpy as np
def sum_rows_numpy(array_2d):
"""
Sums the rows of a 2D array using NumPy.
Args:
array_2d: A 2D array (list of lists or NumPy array).
Returns:
A NumPy array containing the sum of each row. Returns None if input is invalid.
"""
try:
np_array = np.array(array_2d) #Convert to NumPy array if it's a list of lists
row_sums = np.sum(np_array, axis=1)
return row_sums
except (ValueError, TypeError):
return None #Handle cases where input is not a valid 2D array
my_array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
row_sums = sum_rows_numpy(my_array)
print(f"Row sums (NumPy): {row_sums}") # Output: Row sums (NumPy): [ 6 15 24]
invalid_array = "not an array"
row_sums = sum_rows_numpy(invalid_array)
print(f"Row sums for invalid input (NumPy): {row_sums}") #Output: Row sums for invalid input (NumPy): None
NumPy's np.sum()
function with axis=1
efficiently calculates the sum along each row. This approach leverages NumPy's optimized underlying implementation, resulting in a substantial performance improvement for large datasets. The example also includes error handling for robustness.
Functional Programming Approach (Python)
Python's functional programming capabilities offer an elegant alternative. The map()
function combined with sum()
can concisely achieve row summation:
def sum_rows_functional(array_2d):
"""
Sums rows of a 2D array using Python's map and sum functions.
Args:
array_2d: A 2D array (list of lists).
Returns:
A list containing the sum of each row. Returns an empty list if input is invalid.
"""
try:
return list(map(sum, array_2d))
except TypeError:
return []
my_array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
row_sums = sum_rows_functional(my_array)
print(f"Row sums (functional): {row_sums}") # Output: Row sums (functional): [6, 15, 24]
This approach is more concise but might not offer the same performance benefits as NumPy for extremely large arrays.
Handling Irregular 2D Arrays
The previous examples assumed rectangular 2D arrays (all rows have the same number of columns). If you're working with irregular arrays, you'll need to modify the approach to handle rows of varying lengths.
def sum_rows_irregular(array_2d):
"""
Sums the rows of an irregular 2D array.
Args:
array_2d: A 2D array (list of lists) with potentially varying row lengths.
Returns:
A list containing the sum of each row. Returns an empty list if input is invalid.
"""
try:
row_sums = [sum(row) for row in array_2d]
return row_sums
except TypeError:
return []
irregular_array = [[1, 2], [3, 4, 5], [6]]
row_sums = sum_rows_irregular(irregular_array)
print(f"Row sums (irregular): {row_sums}") # Output: Row sums (irregular): [3, 12, 6]
This example uses a list comprehension for a concise and efficient solution for irregular arrays.
Choosing the Right Approach
The optimal method for summing rows in a 2D array depends on several factors:
- Array size: For small arrays, the basic iterative approach is sufficient. For larger arrays, NumPy offers significant performance advantages.
- Programming language: The specific language and its available libraries will influence your choice. NumPy in Python is highly recommended for performance-critical applications.
- Array regularity: If you have an irregular array (rows with different lengths), you'll need an approach that handles this variability.
Advanced Techniques and Considerations
For extremely large datasets, consider using parallel processing techniques to further enhance performance. Libraries like multiprocessing in Python can distribute the row summation task across multiple cores.
Furthermore, always prioritize error handling. Check for invalid input types (e.g., not a list of lists) and handle potential exceptions gracefully. Robust error handling ensures your code's stability and reliability.
Conclusion
Summing rows in a 2D array is a common task with various efficient solutions. Understanding the nuances of different approaches, including iterative methods, NumPy's vectorized operations, and functional programming techniques, empowers you to choose the most appropriate method based on your specific needs and the scale of your data. Remember to prioritize efficient coding practices and comprehensive error handling for robust and reliable results. By mastering these techniques, you can effectively process 2D arrays and build more efficient and sophisticated applications.
Latest Posts
Latest Posts
-
The Sandwich Approach To Delivering Bad News
Mar 29, 2025
-
Within The Relevant Range Of Activity Costs
Mar 29, 2025
-
Exercise 6 12 Bank Reconciliation Lo P3
Mar 29, 2025
-
Joe Decides To Build A Chicken Coop
Mar 29, 2025
-
A High Degree Of Operating Leverage Means
Mar 29, 2025
Related Post
Thank you for visiting our website which covers about 8.2.7 Sum Rows In A 2d Array . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.