Which Statement Best Describes The Function Below
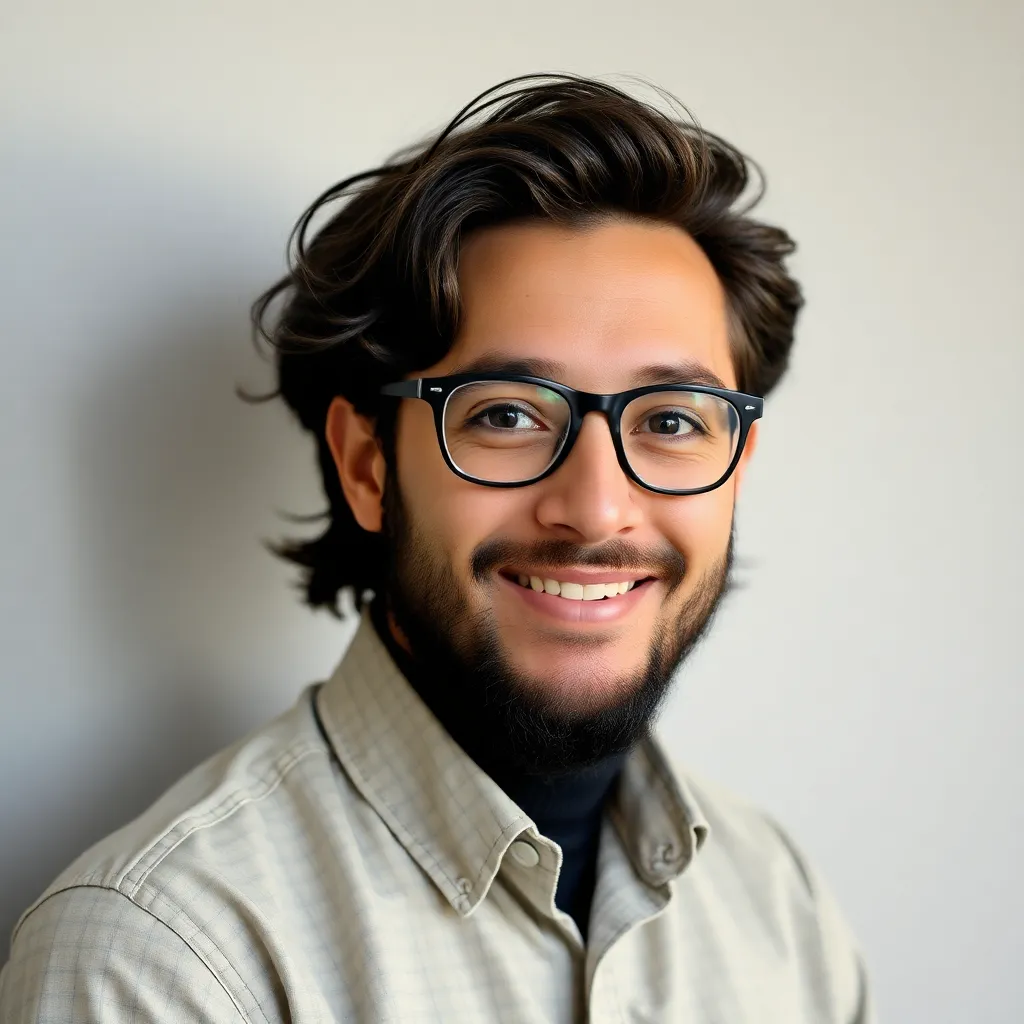
Holbox
Mar 26, 2025 · 5 min read
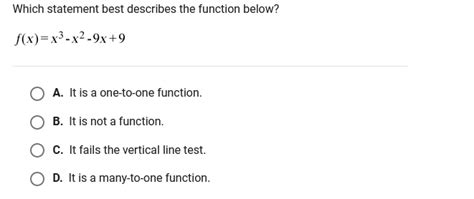
Table of Contents
- Which Statement Best Describes The Function Below
- Table of Contents
- Decoding Function Behavior: A Comprehensive Guide to Understanding and Describing Function Functionality
- What Makes a Statement "Best"?
- Analyzing Function Behavior: A Step-by-Step Approach
- Examples and Analysis
- Beyond Simple Statements: Adding Nuance and Detail
- The Importance of Clear Function Documentation
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
Decoding Function Behavior: A Comprehensive Guide to Understanding and Describing Function Functionality
Understanding the function of a given code snippet is crucial in programming. This isn't simply about identifying the what – what the code does – but also the how – how it achieves its outcome and the underlying logic. This article dives deep into the process of analyzing functions, focusing on choosing the best statement to describe its behavior, encompassing various aspects like input-output relationships, algorithmic complexity, and error handling.
What Makes a Statement "Best"?
Before delving into examples, let's clarify what constitutes the "best" statement describing a function. Several factors contribute:
- Accuracy: The statement must precisely reflect the function's behavior, avoiding oversimplification or misleading generalizations.
- Conciseness: Clarity is key. The statement should be brief and to the point, avoiding unnecessary jargon or technical detail unless absolutely essential for accurate representation.
- Completeness: It should encompass all aspects of the function's behavior, including edge cases, error handling, and any side effects.
- Clarity: The statement should be easily understandable by a programmer with a reasonable level of experience. Avoid ambiguity or jargon that only specialists would understand.
Analyzing Function Behavior: A Step-by-Step Approach
Analyzing a function involves a structured approach:
-
Identify Inputs and Outputs: What data does the function accept as input? What data does it return as output? Understanding this fundamental relationship is the first step. Data types, dimensions (for arrays or matrices), and potential ranges are all important considerations.
-
Trace Execution: Mentally (or using a debugger) step through the code's execution, considering different input values. Pay close attention to control flow (loops, conditional statements), variable assignments, and function calls.
-
Identify the Algorithm: What is the underlying algorithm or logic employed by the function? Is it a sorting algorithm, a search algorithm, a recursive algorithm, or something else entirely? Understanding the algorithm sheds light on the function's computational complexity and behavior.
-
Handle Edge Cases: Consider boundary conditions and unusual inputs. How does the function behave when given empty inputs, null values, or inputs outside its expected range? Proper handling of edge cases is crucial for robust code.
-
Analyze Error Handling: Does the function incorporate error handling mechanisms? How does it respond to invalid inputs or exceptional conditions? This aspect is crucial for evaluating its robustness and reliability.
-
Consider Side Effects: Does the function modify any external state? Does it modify global variables, write to files, or interact with external systems? These side effects are often crucial in determining the function's overall impact.
Examples and Analysis
Let's analyze several example functions and determine the best statement to describe their functionality:
Example 1: A Simple Summation Function
def sum_numbers(a, b):
"""This function adds two numbers together."""
return a + b
Best Statement: "The sum_numbers
function returns the sum of its two numerical arguments."
This statement is accurate, concise, and complete. It clearly defines the input and output, and there are no hidden complexities or side effects.
Example 2: A String Reversal Function
def reverse_string(input_string):
"""Reverses a given string."""
return input_string[::-1]
Best Statement: "The reverse_string
function returns a new string that is the reverse of the input string."
This statement accurately describes the function's behavior. It emphasizes that a new string is created, avoiding any confusion about in-place modification.
Example 3: A Function with Error Handling
def divide_numbers(a, b):
"""Divides two numbers, handling division by zero."""
try:
result = a / b
return result
except ZeroDivisionError:
return "Error: Division by zero"
Best Statement: "The divide_numbers
function divides the first argument by the second. It returns the result of the division, or an error message if the second argument is zero."
This statement accurately conveys the function's core functionality and its error-handling mechanism. It's concise and clearly explains the two potential outcomes.
Example 4: A More Complex Function (Finding the Maximum in an Array)
def find_maximum(numbers):
"""Finds the maximum number in a list of numbers. Returns None if the list is empty."""
if not numbers:
return None
max_num = numbers[0]
for number in numbers:
if number > max_num:
max_num = number
return max_num
Best Statement: "The find_maximum
function iterates through a list of numbers and returns the largest number. If the input list is empty, it returns None
."
This statement is accurate, clearly describing the algorithm and edge case handling.
Example 5: A Function with Side Effects
global_variable = 0
def increment_global(amount):
"""Increments a global variable by a given amount."""
global global_variable
global_variable += amount
return global_variable
Best Statement: "The increment_global
function increments the global variable global_variable
by the given amount and returns the updated value of the global variable."
This statement highlights the crucial side effect: modification of a global variable. It's essential to mention this side effect because it affects the program's state beyond the function's immediate scope.
Beyond Simple Statements: Adding Nuance and Detail
For more complex functions, a single sentence might not suffice. In such cases, a more detailed description might be necessary, using bullet points or a paragraph to break down the functionality into smaller, more manageable chunks. For instance, if a function involves multiple steps or uses complex algorithms, a more elaborate explanation, perhaps accompanied by pseudocode or a flowchart, might be needed to fully capture its behavior.
The Importance of Clear Function Documentation
The process of writing a clear and accurate description of a function's behavior is intrinsically linked to the practice of writing good function documentation. Well-written documentation not only aids understanding but also significantly improves code maintainability, collaboration, and overall project success. The principles outlined in this article should inform how you write your function documentation, ensuring it is accurate, complete, and easily understandable by others (including your future self!).
Conclusion
Describing the function of a given code snippet requires a meticulous and systematic approach. By carefully examining the inputs, outputs, algorithm, error handling, and side effects, we can create concise, accurate, and clear statements that accurately represent a function's behavior. This approach is essential for both understanding code and for creating effective documentation that enhances collaboration and maintainability. Remember that the "best" statement is the one that is most accurate, concise, complete, and easily understandable in the context of its intended audience.
Latest Posts
Latest Posts
-
Lagle Corporation Has Provided The Following Information
Mar 29, 2025
-
The Clear Zone Around An Antibiotic Disk
Mar 29, 2025
-
Experiment 25 Ph Measurements Buffers And Their Properties
Mar 29, 2025
-
What Should Managers Do When It Comes To Stress Reduction
Mar 29, 2025
-
Draw The Major Organic Product Of The Following Reaction Sequence
Mar 29, 2025
Related Post
Thank you for visiting our website which covers about Which Statement Best Describes The Function Below . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.