Which Code Example Is An Expression
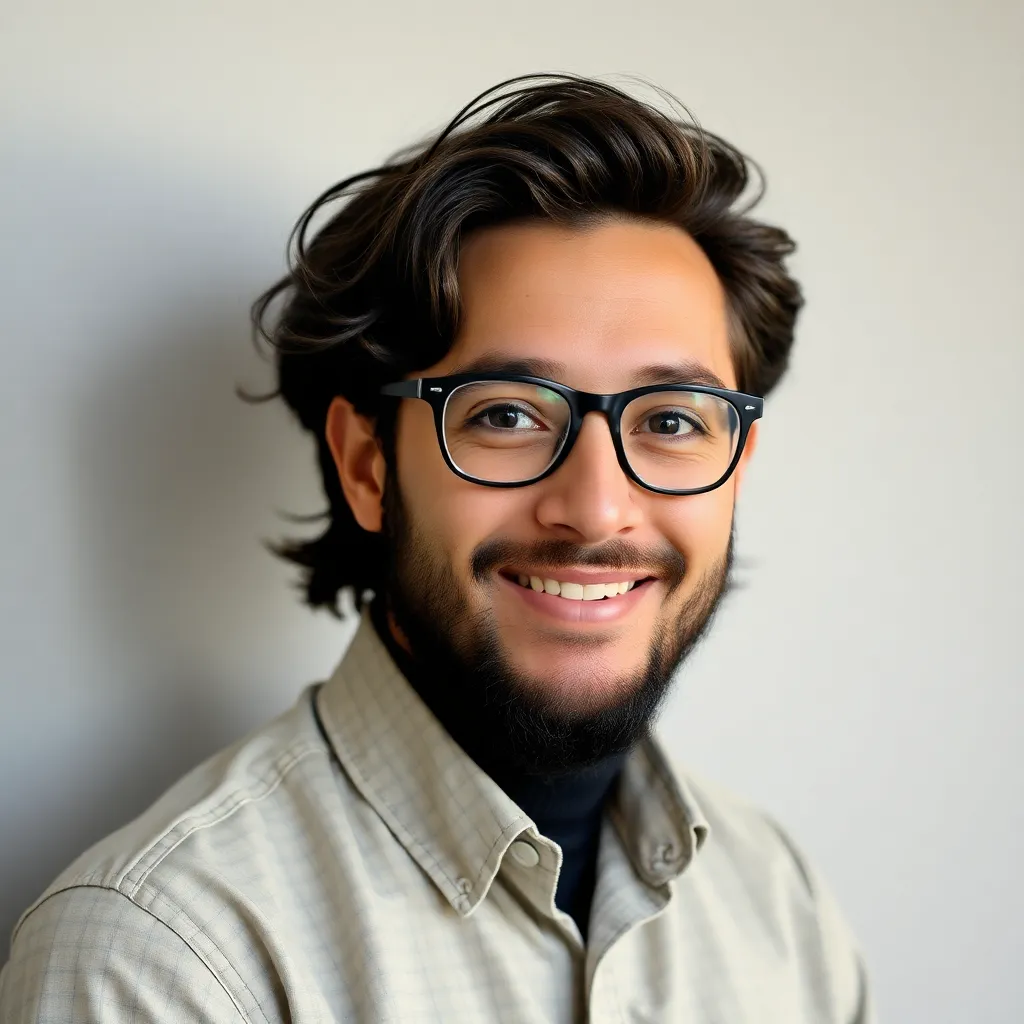
Holbox
Apr 03, 2025 · 5 min read

Table of Contents
- Which Code Example Is An Expression
- Table of Contents
- Which Code Example is an Expression? A Deep Dive into Programming Fundamentals
- What is an Expression?
- Key Characteristics of Expressions:
- Contrasting Expressions with Statements
- Examples of Expressions Across Programming Languages
- JavaScript Expressions:
- Python Expressions:
- C++ Expressions:
- Java Expressions:
- Identifying Expressions: A Practical Guide
- Common Pitfalls and Misconceptions
- Conclusion: Mastering Expressions for Better Code
- Latest Posts
- Latest Posts
- Related Post
Which Code Example is an Expression? A Deep Dive into Programming Fundamentals
Understanding the difference between statements and expressions is crucial for any programmer, regardless of their chosen language. While both are fundamental building blocks of code, they serve distinct purposes and have different characteristics. This comprehensive guide delves into the core concept of expressions, providing clear explanations, examples across various programming languages (including JavaScript, Python, C++, and Java), and practical tips to help you confidently identify expressions in your code.
What is an Expression?
In programming, an expression is a combination of values, variables, operators, and function calls that evaluates to a single value. Think of it as a mathematical formula or a concise piece of code that produces a result. Crucially, an expression always results in a value. This value can then be used in other parts of the program, assigned to a variable, or printed to the console.
Key Characteristics of Expressions:
- Evaluation: Expressions are evaluated by the interpreter or compiler to produce a single value.
- Value Production: The primary purpose of an expression is to generate a result.
- Operands and Operators: Expressions typically involve operands (values or variables) and operators (symbols that perform operations on operands).
- Composable: Expressions can be nested within other expressions to create more complex calculations.
Contrasting Expressions with Statements
It's important to distinguish expressions from statements. While expressions produce values, statements perform actions. Statements don't necessarily return a value; their purpose is to execute a specific command or control the flow of the program. Examples of statements include conditional statements (if
, else
), loops (for
, while
), function definitions, and assignments that don't involve an expression on the right-hand side.
Examples of Expressions Across Programming Languages
Let's examine various code examples to illustrate what constitutes an expression.
JavaScript Expressions:
// Arithmetic expressions
let x = 10 + 5; // Addition
let y = 20 / 4; // Division
let z = x * y; // Multiplication
// String expressions
let message = "Hello, " + "world!"; // Concatenation
// Boolean expressions
let isGreater = x > y; // Comparison
let isEqual = x === 15; // Equality
// Function call expressions
let result = Math.sqrt(25); // Calling a Math function
// Object property access
let person = { name: "Alice", age: 30 };
let age = person.age; // Accessing an object property
// Array element access
let numbers = [1, 2, 3, 4, 5];
let thirdNumber = numbers[2]; // Accessing an array element
// Ternary Operator (conditional expression)
let status = age >= 18 ? "Adult" : "Minor";
All the examples above in JavaScript are expressions because they evaluate to a single value. Even the ternary operator, although it has a conditional aspect, ultimately produces a value ("Adult" or "Minor").
Python Expressions:
# Arithmetic expressions
x = 10 + 5
y = 20 // 4 # Integer division
z = x * y
# String expressions
message = "Hello, " + "world!"
# Boolean expressions
is_greater = x > y
is_equal = x == 15
# Function call expressions
result = math.sqrt(25) # Requires importing the 'math' module
# List element access
numbers = [1, 2, 3, 4, 5]
third_number = numbers[2]
# Conditional expression (ternary operator)
status = "Adult" if age >= 18 else "Minor"
Python's syntax differs slightly from JavaScript, but the fundamental principle remains the same. Every example above is an expression because it evaluates to a single value.
C++ Expressions:
#include
#include
int main() {
// Arithmetic expressions
int x = 10 + 5;
int y = 20 / 4;
int z = x * y;
// String expressions (using string concatenation)
std::string message = "Hello, " + "world!";
// Boolean expressions
bool isGreater = x > y;
bool isEqual = x == 15;
// Function call expressions
double result = std::sqrt(25); // Using the cmath library
// Array element access
int numbers[] = {1, 2, 3, 4, 5};
int thirdNumber = numbers[2];
std::cout << message << std::endl; // Output: Hello, world!
return 0;
}
C++ shares similarities with other languages, illustrating that the concept of an expression transcends specific syntax.
Java Expressions:
public class Main {
public static void main(String[] args) {
// Arithmetic expressions
int x = 10 + 5;
int y = 20 / 4;
int z = x * y;
// String expressions
String message = "Hello, " + "world!";
// Boolean expressions
boolean isGreater = x > y;
boolean isEqual = x == 15;
// Function call expressions
double result = Math.sqrt(25);
// Array element access
int[] numbers = {1, 2, 3, 4, 5};
int thirdNumber = numbers[2];
System.out.println(message); // Output: Hello, world!
}
}
Again, the core concept of an expression producing a value remains consistent across Java.
Identifying Expressions: A Practical Guide
To determine if a piece of code is an expression, ask yourself these questions:
-
Does it produce a value? If the code snippet doesn't result in a single value, it's likely a statement.
-
Can it be used as part of a larger expression? Expressions can be nested within other expressions. If a piece of code can be seamlessly integrated into a more complex calculation, it's probably an expression.
-
Does it involve operators and operands? Most expressions involve operators performing operations on operands (values or variables).
-
Can you assign its result to a variable? If you can assign the output of a code snippet to a variable, it is most likely an expression.
Common Pitfalls and Misconceptions
-
Assignments are not always expressions: While
x = 5
is an assignment, it's not an expression in many languages. In some languages, it might return a value (the value assigned), but its primary function is assignment, not value generation. -
Function calls are expressions: Calling a function that returns a value (as opposed to a void function) creates an expression. The result of the function call becomes the value of the expression.
-
Conditional expressions (ternary operator): While they look conditional, ternary operators (
condition ? value1 : value2
) in languages like JavaScript, Python, and C++ ultimately produce a single value, making them expressions.
Conclusion: Mastering Expressions for Better Code
Understanding the nuances of expressions is fundamental to writing clean, efficient, and readable code. By grasping the core characteristics of expressions and being able to distinguish them from statements, you lay a strong foundation for more advanced programming concepts. This guide, with its diverse examples and practical tips, empowers you to confidently identify expressions and leverage their power in your coding journey. Remember to always practice, experiment, and consult language documentation for specific details. Consistent practice will solidify your understanding and make you a more proficient programmer.
Latest Posts
Latest Posts
-
What Would Be The Effect Of A Reduced Venous Return
Apr 06, 2025
-
Iso 9000 Seeks Standardization In Terms Of
Apr 06, 2025
-
If Xy 2 X 2 Y 5 Then Dy Dx
Apr 06, 2025
-
Below Is The Lewis Structure Of The Formaldehyde Molecule
Apr 06, 2025
-
Art Labeling Activity Anatomy And Histology Of The Thyroid Gland
Apr 06, 2025
Related Post
Thank you for visiting our website which covers about Which Code Example Is An Expression . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.