Use A Row Level Button To Collapse
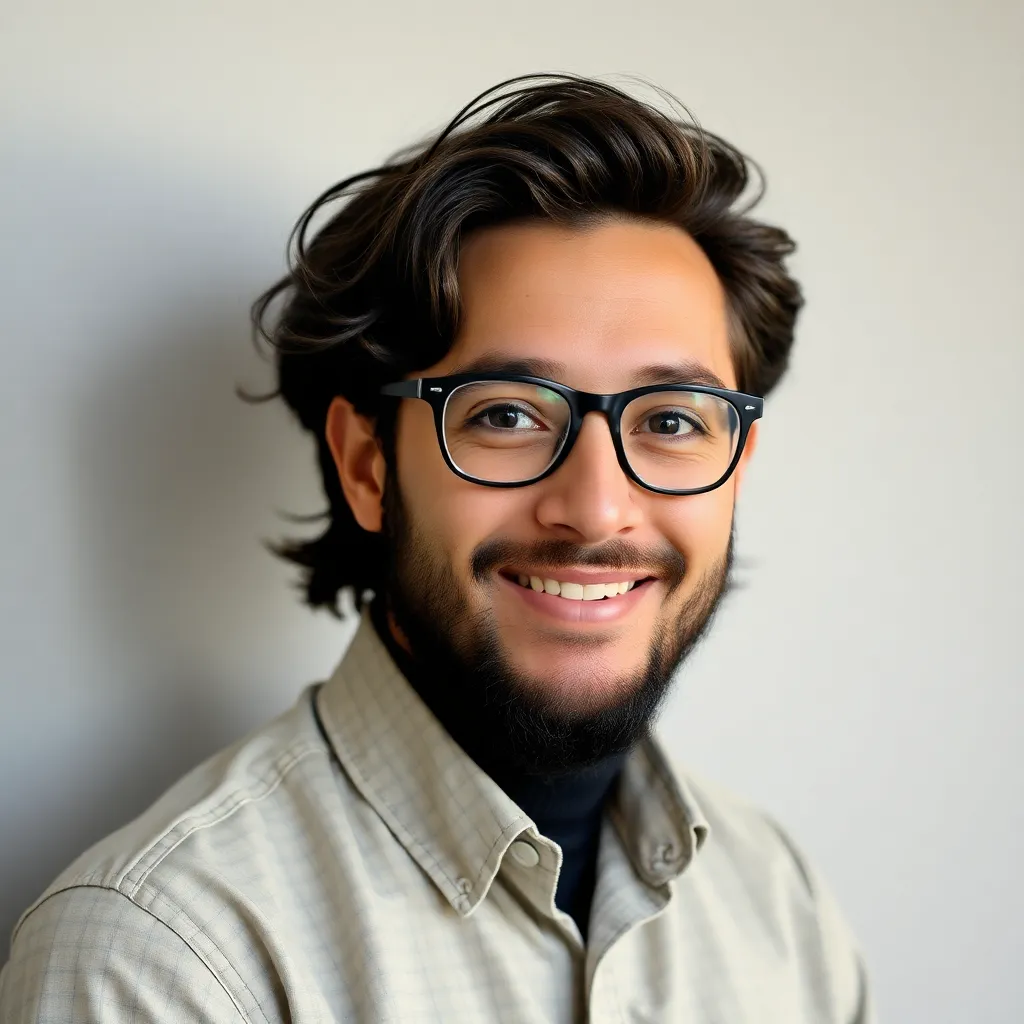
Holbox
Mar 22, 2025 · 6 min read
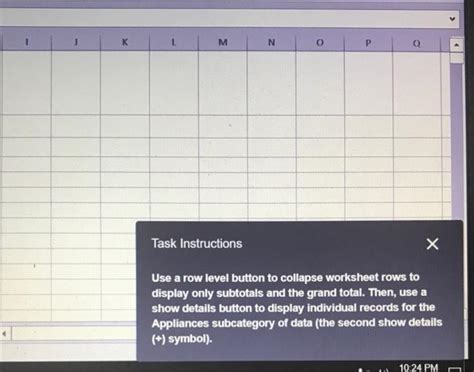
Table of Contents
- Use A Row Level Button To Collapse
- Table of Contents
- Use a Row-Level Button to Collapse: Enhancing User Experience and Efficiency
- Understanding the Advantages of Row-Level Collapse
- Implementing Row-Level Collapse: A Multi-faceted Approach
- JavaScript Implementation: Pure Vanilla JavaScript
- jQuery Implementation: Leveraging jQuery's Power
- Server-Side Frameworks (Example: Node.js with React)
- Best Practices and Considerations
- Advanced Techniques and Extensions
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
Use a Row-Level Button to Collapse: Enhancing User Experience and Efficiency
Row-level buttons that collapse or expand sections of data within a table or grid are a powerful tool for improving user experience and managing large datasets. This approach offers a dynamic and interactive way to present information, allowing users to focus on specific areas of interest while minimizing visual clutter. This comprehensive guide explores various methods for implementing row-level collapse functionality, addressing considerations for different programming languages and frameworks, and highlighting best practices for optimizing performance and usability.
Understanding the Advantages of Row-Level Collapse
Before diving into implementation details, let's examine the key advantages of using row-level buttons to collapse data:
-
Improved Readability and Scannability: Large datasets can be overwhelming. Collapsing allows users to quickly scan key information without being bogged down in details. This significantly improves readability and reduces cognitive load.
-
Enhanced User Experience: By giving users control over what information they see, row-level collapse provides a more personalized and interactive experience. They can selectively expand only the rows that are relevant to their current task.
-
Optimized Performance: For extremely large datasets, loading and rendering all data simultaneously can be slow. Collapsing only loads and renders the expanded rows, leading to improved performance, especially on less powerful devices.
-
Better Information Management: Row-level collapse helps organize and manage large amounts of data effectively. Users can easily focus on specific entries while keeping the overall structure of the data visible.
-
Space Optimization: Collapsing unused rows frees up valuable screen real estate, making the interface cleaner and more efficient, particularly on smaller screens or displays.
Implementing Row-Level Collapse: A Multi-faceted Approach
The implementation of row-level collapse varies significantly based on the technology stack. However, the core principles remain consistent. We will explore common approaches using JavaScript, jQuery, and considerations for server-side frameworks.
JavaScript Implementation: Pure Vanilla JavaScript
This approach uses only plain JavaScript without relying on external libraries like jQuery. While slightly more verbose, it provides a fundamental understanding of the underlying mechanisms.
// Sample HTML structure (assuming a table)
Name
Details
Row 1
CollapseDetailed information for Row 1...
Row 2
CollapseDetailed information for Row 2...
function toggleRow(rowId) {
const row = document.querySelector(`tr[data-row-id="${rowId}"]`);
const details = row.querySelector('.details');
details.style.display = details.style.display === 'none' ? 'block' : 'none';
const button = row.querySelector('button');
button.textContent = details.style.display === 'none' ? 'Expand' : 'Collapse';
}
This code uses data attributes (data-row-id
) for easy identification and manipulation of rows. The toggleRow
function handles the display of the details section and updates the button text accordingly. This is a simple example; more sophisticated implementations might involve animations or more complex data handling.
jQuery Implementation: Leveraging jQuery's Power
jQuery simplifies many DOM manipulation tasks, making row-level collapse implementation more concise and efficient.
// Sample HTML (similar to the JavaScript example)
// jQuery code
$(document).ready(function() {
$('.collapse-button').click(function() {
$(this).closest('tr').find('.details').slideToggle();
$(this).text(function(_, text){
return text === 'Collapse' ? 'Expand' : 'Collapse';
});
});
});
This jQuery code utilizes the slideToggle()
method for a smoother visual transition. The closest()
method efficiently finds the parent <tr>
element, and find()
targets the .details
section. The .text()
method dynamically updates the button label.
Server-Side Frameworks (Example: Node.js with React)
Server-side frameworks often involve more complex data management. React, for instance, allows for efficient rendering and updating of components. Here's a conceptual overview; the exact implementation depends on your chosen framework and data source.
// Conceptual React Component (simplified)
function Row({ data }) {
const [isExpanded, setIsExpanded] = useState(false);
return (
{data.name}
setIsExpanded(!isExpanded)}>
{isExpanded ? 'Collapse' : 'Expand'}
{isExpanded && {data.details}}
);
}
React's component-based structure facilitates efficient updating of the UI when the isExpanded
state changes. This approach leverages React's virtual DOM for optimal performance, especially with extensive datasets.
Best Practices and Considerations
-
Accessibility: Ensure your implementation adheres to accessibility guidelines (WCAG). Use appropriate ARIA attributes to improve accessibility for screen readers and assistive technologies. For example, using ARIA attributes like
aria-expanded
on the button to indicate the expansion state is crucial. -
Performance Optimization: For very large datasets, consider lazy loading or pagination to prevent performance bottlenecks. Only load and render the necessary data when a row is expanded.
-
Error Handling: Implement robust error handling to gracefully manage potential issues, such as network errors when fetching data or unexpected data structures.
-
User Feedback: Provide clear visual feedback to the user, such as animations or loading indicators, during the collapse/expand process. This improves the overall user experience.
-
Responsiveness: Ensure your implementation works well across different devices and screen sizes. Use responsive design techniques to provide an optimal experience on mobile, tablets, and desktops.
-
CSS Styling: Use CSS to style the collapsed and expanded states for visual clarity and consistency. This helps users easily understand the current state of each row.
-
Testing: Thoroughly test your implementation to ensure it functions correctly across different browsers and devices. Test with both small and large datasets.
Advanced Techniques and Extensions
-
Nested Collapses: Allow for nested levels of collapsing, enabling users to drill down into increasingly granular details.
-
Customizable Collapse Behavior: Provide users with options to customize the collapse behavior, such as setting default collapse states or configuring animation speeds.
-
Integration with other UI elements: Integrate row-level collapse with other UI elements such as search filters or sorting capabilities to create a more comprehensive data management system.
-
Data Persistence: Consider preserving the collapse/expand state of rows even after page refresh or navigation using local storage or server-side persistence.
Conclusion
Implementing row-level collapse buttons is a powerful technique to enhance the user experience and manage large datasets effectively. By carefully considering various implementation approaches, optimizing performance, and adhering to best practices, developers can create intuitive and efficient interfaces that empower users to interact with data more meaningfully. The choice of implementation (pure JavaScript, jQuery, or a server-side framework) depends on your project requirements and existing technology stack. However, regardless of the approach, always prioritize accessibility, performance, and a user-centered design. By following the guidelines outlined in this guide, you can successfully integrate this valuable feature into your applications, leading to improved user satisfaction and a more engaging user experience. Remember that continuous testing and refinement are crucial for ensuring optimal performance and usability.
Latest Posts
Latest Posts
-
Only A Person Could Believe Her Tale
Mar 24, 2025
-
Which Statement About Schizophrenia Is True
Mar 24, 2025
-
What Is The Adjective Form Of Heart
Mar 24, 2025
-
Histamine Serotonin And Bradykinin Are All
Mar 24, 2025
-
30 40 50 40 45 50
Mar 24, 2025
Related Post
Thank you for visiting our website which covers about Use A Row Level Button To Collapse . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.