Populating An Array With A For Loop
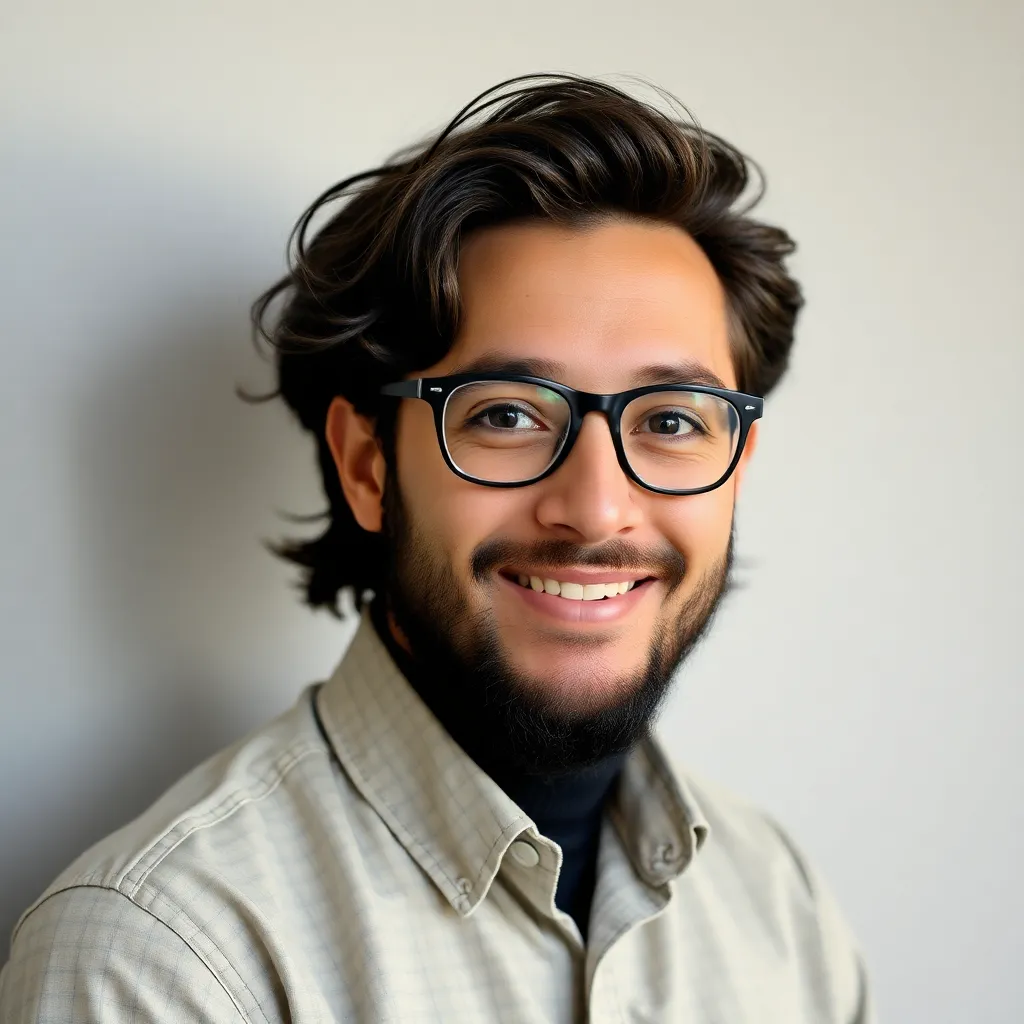
Holbox
Mar 28, 2025 · 6 min read
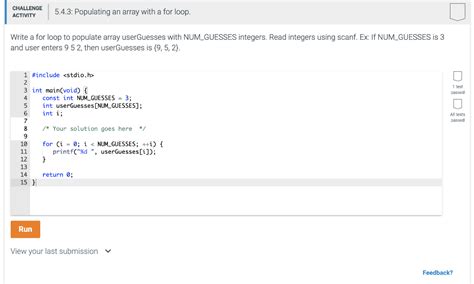
Table of Contents
- Populating An Array With A For Loop
- Table of Contents
- Populating an Array with a For Loop: A Comprehensive Guide
- Understanding Arrays and For Loops
- Populating Arrays with For Loops: A Step-by-Step Guide
- Examples Across Programming Languages
- Python
- JavaScript
- Java
- C++
- C#
- Advanced Techniques and Best Practices
- Populating with Specific Patterns
- Handling User Input
- Multi-dimensional Arrays
- Using Enhanced for Loops (Where Applicable)
- Error Handling and Input Validation
- Choosing Appropriate Data Structures
- Common Pitfalls to Avoid
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
Populating an Array with a For Loop: A Comprehensive Guide
Populating an array using a for
loop is a fundamental programming concept. It's a powerful and versatile technique used across various programming languages to efficiently create and fill arrays with data. This comprehensive guide will explore this concept in detail, covering different scenarios, best practices, and common pitfalls. We'll delve into various programming languages to illustrate the universality of this technique.
Understanding Arrays and For Loops
Before diving into the specifics of populating arrays, let's briefly review the concepts of arrays and for
loops.
Arrays: An array is a data structure that stores a collection of elements of the same data type in contiguous memory locations. Each element can be accessed using its index, which is its position within the array. Indices typically start at 0 in most programming languages.
For Loops: A for
loop is a control flow statement that allows you to repeatedly execute a block of code for a specified number of times. It's particularly useful when you know the number of iterations in advance. The general structure of a for
loop is:
for (initialization; condition; increment/decrement) {
// Code to be executed repeatedly
}
Populating Arrays with For Loops: A Step-by-Step Guide
The process of populating an array using a for
loop involves initializing an array, then iterating through the loop, assigning values to each element of the array based on your needs. Here's a breakdown:
-
Array Initialization: First, you need to create an array of the desired size. The method for this varies slightly depending on the programming language.
-
Loop Initialization: The
for
loop's initialization step sets up a counter variable that will track the current index of the array. -
Loop Condition: The loop's condition determines how many times the loop will execute. Typically, this is a comparison involving the counter variable and the array's size.
-
Element Assignment: Inside the loop, you assign a value to each array element using the counter variable as the index.
-
Loop Increment/Decrement: The increment/decrement step updates the counter variable after each iteration, moving to the next array element.
Examples Across Programming Languages
Let's illustrate this process with examples in several popular programming languages:
Python
# Creating an array (list in Python) of size 5 and populating it with numbers
my_array = [0] * 5 # Initialize with 5 zeros
for i in range(5):
my_array[i] = i * 2 # Assign values: 0, 2, 4, 6, 8
print(my_array) # Output: [0, 2, 4, 6, 8]
# Populating with user input
my_array = [0] * 5
for i in range(5):
my_array[i] = int(input(f"Enter value for element {i}: "))
print(my_array)
JavaScript
// Creating an array of size 5 and populating it with numbers
let myArray = new Array(5).fill(0); // Initialize with 5 zeros
for (let i = 0; i < 5; i++) {
myArray[i] = i * 3; // Assign values: 0, 3, 6, 9, 12
}
console.log(myArray); // Output: [0, 3, 6, 9, 12]
//Populating with random numbers between 1 and 100
let randomArray = new Array(10).fill(0);
for(let i = 0; i < 10; i++){
randomArray[i] = Math.floor(Math.random() * 100) + 1;
}
console.log(randomArray);
Java
// Creating an array of size 5 and populating it with numbers
int[] myArray = new int[5];
for (int i = 0; i < myArray.length; i++) {
myArray[i] = i * 4; // Assign values: 0, 4, 8, 12, 16
}
for (int i = 0; i < myArray.length; i++) {
System.out.print(myArray[i] + " "); // Output: 0 4 8 12 16
}
System.out.println(); // New line for better readability
C++
#include
#include
int main() {
// Creating an array (vector in C++) of size 5 and populating it with numbers
std::vector myArray(5);
for (int i = 0; i < myArray.size(); i++) {
myArray[i] = i * 5; // Assign values: 0, 5, 10, 15, 20
}
for (int i = 0; i < myArray.size(); i++) {
std::cout << myArray[i] << " "; // Output: 0 5 10 15 20
}
std::cout << std::endl; // New line for better readability
return 0;
}
C#
// Creating an array of size 5 and populating it with numbers
int[] myArray = new int[5];
for (int i = 0; i < myArray.Length; i++) {
myArray[i] = i * 6; // Assign values: 0, 6, 12, 18, 24
}
foreach (int number in myArray) {
Console.Write(number + " "); // Output: 0 6 12 18 24
}
Console.WriteLine(); // New line for better readability
Advanced Techniques and Best Practices
Beyond the basic examples, let's explore some advanced techniques and best practices:
Populating with Specific Patterns
You can use for
loops to populate arrays with various patterns, such as:
- Arithmetic sequences:
myArray[i] = a + i * d
(wherea
is the first term andd
is the common difference). - Geometric sequences:
myArray[i] = a * r^i
(wherea
is the first term andr
is the common ratio). - Fibonacci sequence: Requires a slightly more complex loop to handle the recursive nature of the sequence.
Handling User Input
You can use for
loops to prompt the user for input and populate the array based on their responses, as demonstrated in some examples above. Error handling is crucial here to manage potential exceptions (e.g., non-numeric input).
Multi-dimensional Arrays
The principles extend to multi-dimensional arrays (matrices). You would use nested for
loops, one for each dimension.
# Creating a 3x3 matrix and populating it with values
matrix = [[0 for _ in range(3)] for _ in range(3)]
for i in range(3):
for j in range(3):
matrix[i][j] = i * 3 + j
print(matrix) #Output: [[0, 1, 2], [3, 4, 5], [6, 7, 8]]
Using Enhanced for
Loops (Where Applicable)
Some languages offer enhanced for
loops (e.g., for...of
in JavaScript, for...in
in Python for iterating over dictionaries) that can simplify array population in certain scenarios.
Error Handling and Input Validation
When populating arrays with user input, always include robust error handling and input validation to prevent crashes or unexpected behavior due to incorrect data.
Choosing Appropriate Data Structures
Consider whether an array is the most suitable data structure for your task. Other data structures, like linked lists or hash tables, might be more efficient for certain operations.
Common Pitfalls to Avoid
- Off-by-one errors: Carefully check your loop conditions to avoid going beyond the array's bounds. This is a very common source of errors.
- Incorrect index usage: Ensure you're using the correct index for accessing and modifying array elements.
- Uninitialized arrays: Always initialize your arrays before attempting to populate them.
- Inefficient algorithms: For very large arrays, consider more efficient algorithms or data structures to improve performance.
Conclusion
Populating an array with a for
loop is a fundamental skill for any programmer. Mastering this technique allows for efficient data manipulation and lays the groundwork for more advanced programming concepts. By understanding the process, applying best practices, and avoiding common pitfalls, you can effectively and efficiently populate arrays with data, creating robust and efficient programs. Remember to always consider the specific context of your task and choose the most appropriate techniques for optimal performance and code readability.
Latest Posts
Latest Posts
-
Getting A Bonus For Every Five Customer Referrals
Mar 31, 2025
-
All Of The Following Statements Are True Except
Mar 31, 2025
-
Label The Features Of A Neuromuscular Junction
Mar 31, 2025
-
Which Plane Is Represented By The Following Image
Mar 31, 2025
-
How Do You Cite A Letter In Apa
Mar 31, 2025
Related Post
Thank you for visiting our website which covers about Populating An Array With A For Loop . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.