Coding Exercise: Decoding A Message From A Text File Pytthon
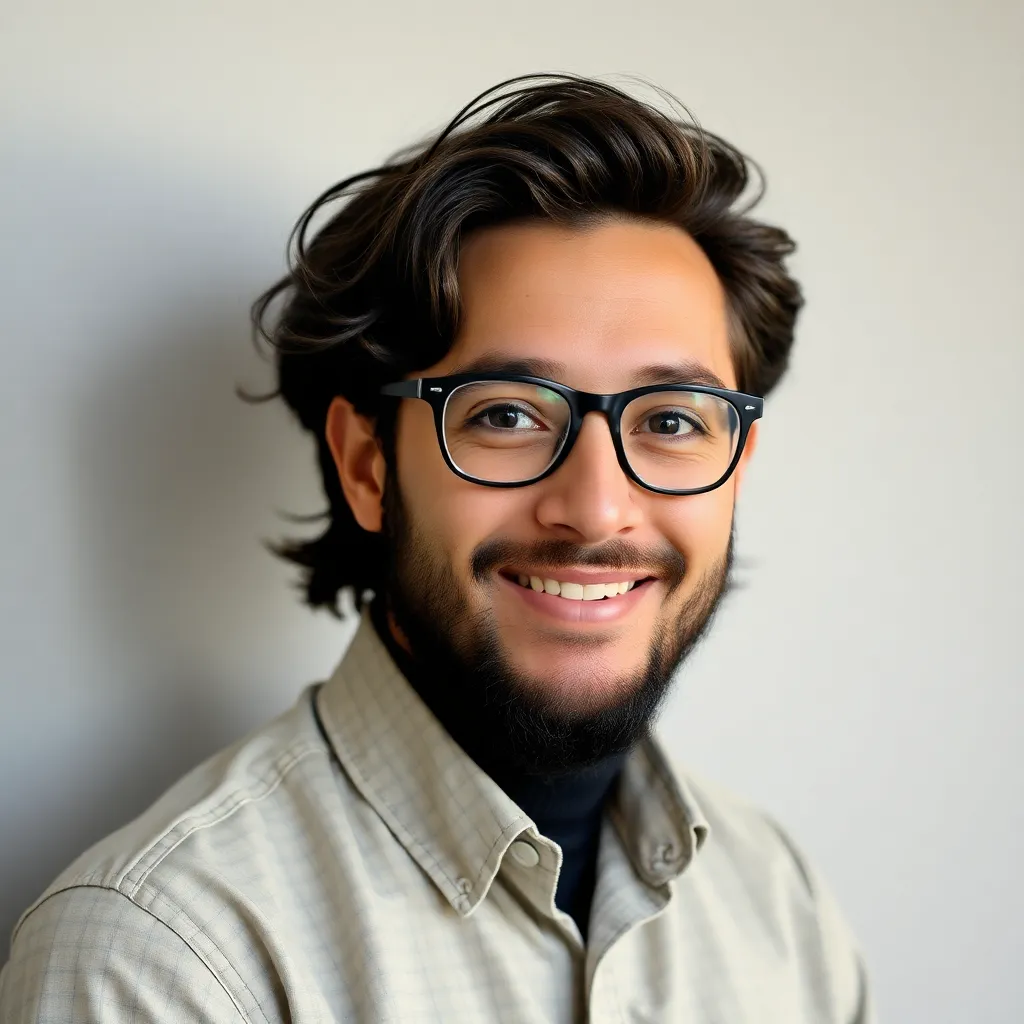
Holbox
Mar 20, 2025 · 5 min read
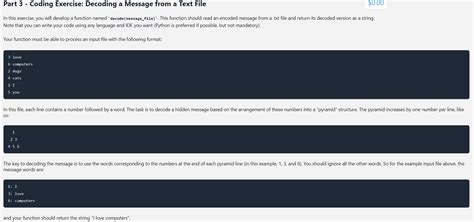
Table of Contents
Coding Exercise: Decoding a Message from a Text File (Python)
This comprehensive guide delves into a coding exercise focused on decoding a hidden message within a text file using Python. We'll explore various techniques, handle potential errors gracefully, and optimize the code for efficiency and readability. This exercise is perfect for honing your Python skills, particularly file handling, string manipulation, and error management. We'll also discuss best practices for writing clean, maintainable code, crucial for larger projects.
Understanding the Challenge
The core challenge is to read a text file containing an encoded message, decipher the code, and output the decoded message. The encoding method itself isn't specified, allowing for flexibility and encouraging creative problem-solving. This opens the door to exploring different encoding techniques and developing robust decoding solutions.
We'll assume the encoding is relatively simple, avoiding complex cryptographic methods. This allows us to focus on the core programming aspects without getting bogged down in intricate cryptography. However, the principles learned here are transferable to more complex scenarios.
Setting up the Environment
Before we begin, ensure you have Python installed on your system. A suitable IDE (Integrated Development Environment) like VS Code, PyCharm, or even a simple text editor will help manage your code. No external libraries are strictly required for this basic exercise, making it accessible to beginners.
Defining the Encoding Method
For this example, let's assume the encoding is a simple Caesar cipher. A Caesar cipher shifts each letter in the alphabet a certain number of places. For instance, with a shift of 3, 'A' becomes 'D', 'B' becomes 'E', and so on. This is a classic cipher, allowing us to focus on the file handling and decoding logic.
Python Code Implementation
The Python code below demonstrates the decoding process. It handles file I/O, error handling, and the Caesar cipher decryption.
def caesar_decode(text, shift):
"""Decodes a Caesar cipher."""
decoded_text = ""
for char in text:
if char.isalpha():
start = ord('a') if char.islower() else ord('A')
shifted_char = chr((ord(char) - start - shift) % 26 + start)
elif char.isdigit():
shifted_char = str((int(char) - shift) % 10)
else:
shifted_char = char # Keep non-alphanumeric characters as they are
decoded_text += shifted_char
return decoded_text
def decode_message(filepath, shift):
"""Reads a file, decodes the message, and handles potential errors."""
try:
with open(filepath, 'r') as file:
encoded_message = file.read().strip() # Strip removes leading/trailing whitespace
decoded_message = caesar_decode(encoded_message, shift)
return decoded_message
except FileNotFoundError:
return "Error: File not found."
except Exception as e:
return f"An error occurred: {e}"
# Example usage:
filepath = "encoded_message.txt"
shift_value = 3 # Adjust the shift value as needed
decoded_result = decode_message(filepath, shift_value)
print(decoded_result)
Breaking Down the Code
Let's analyze the code section by section:
caesar_decode(text, shift)
function:
This function is the heart of the decoding process. It iterates through each character in the input text
. If a character is an alphabet, it shifts it back by the shift
value, handling both uppercase and lowercase letters. It also handles digits using the modulo operator (%
) to ensure the result remains within the valid range. Non-alphanumeric characters are left unchanged. The ord()
function converts characters to their ASCII values, enabling numerical manipulation. The chr()
function converts the ASCII value back to a character.
decode_message(filepath, shift)
function:
This function handles file input and error management. It uses a try-except
block to gracefully handle FileNotFoundError
and other potential exceptions during file operations. The with open(...)
statement ensures the file is automatically closed even if errors occur. The .strip()
method removes any leading or trailing whitespace from the encoded message.
Example Usage:
The example usage shows how to call the decode_message
function, passing the file path and shift value. The decoded result is then printed to the console.
Expanding the Exercise: Handling Different Encoding Schemes
The Caesar cipher is a simple example. We can expand the exercise to handle more complex encoding schemes:
-
Substitution Ciphers: These replace each letter with another letter, not necessarily following a sequential shift. A dictionary could map the encoded letters to their decoded counterparts.
-
Vigenère Cipher: This is a polyalphabetic substitution cipher using a keyword to determine the shift for each letter. The decoding would involve iterating through the keyword and applying the appropriate shift for each character.
-
Base64 Encoding: This encoding scheme represents binary data in an ASCII string format. Python's
base64
module provides functions for encoding and decoding.
Advanced Techniques and Optimization
-
Regular Expressions: Regular expressions (regex) can be used to identify and extract specific patterns within the encoded message, potentially simplifying the decoding process.
-
Efficiency Improvements: For very large files, consider using generators or iterators to process the file line by line, improving memory efficiency.
-
Modular Design: Break down the code into smaller, well-defined functions for better organization and maintainability.
Testing and Debugging
Thorough testing is crucial. Create several test files with different encoded messages and verify the accuracy of your decoding functions. Use print statements or a debugger to trace the execution flow and identify any issues.
Conclusion
This coding exercise provides valuable hands-on experience with file handling, string manipulation, and error handling in Python. By implementing the Caesar cipher decoding and exploring more advanced encoding schemes, you can significantly enhance your Python programming skills. Remember that robust error handling and well-structured code are essential for creating reliable and maintainable programs. The ability to adapt your code to handle different encoding methods demonstrates a strong understanding of the core principles involved. Continue to experiment, refine your techniques, and tackle increasingly complex challenges to further develop your coding proficiency.
Latest Posts
Latest Posts
-
An Example Of An Intermediate Good Would Be
Mar 20, 2025
-
How Do Smartphones Achieve Images With High Dynamic Range
Mar 20, 2025
-
Based On Values In Cells A51 A55
Mar 20, 2025
-
A Frequent Reason For A Stock Split Is To
Mar 20, 2025
-
Mutual Interdependence Means That Each Firm In An Oligopoly
Mar 20, 2025
Related Post
Thank you for visiting our website which covers about Coding Exercise: Decoding A Message From A Text File Pytthon . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.