C Is Trying To Determine Whether To Convert
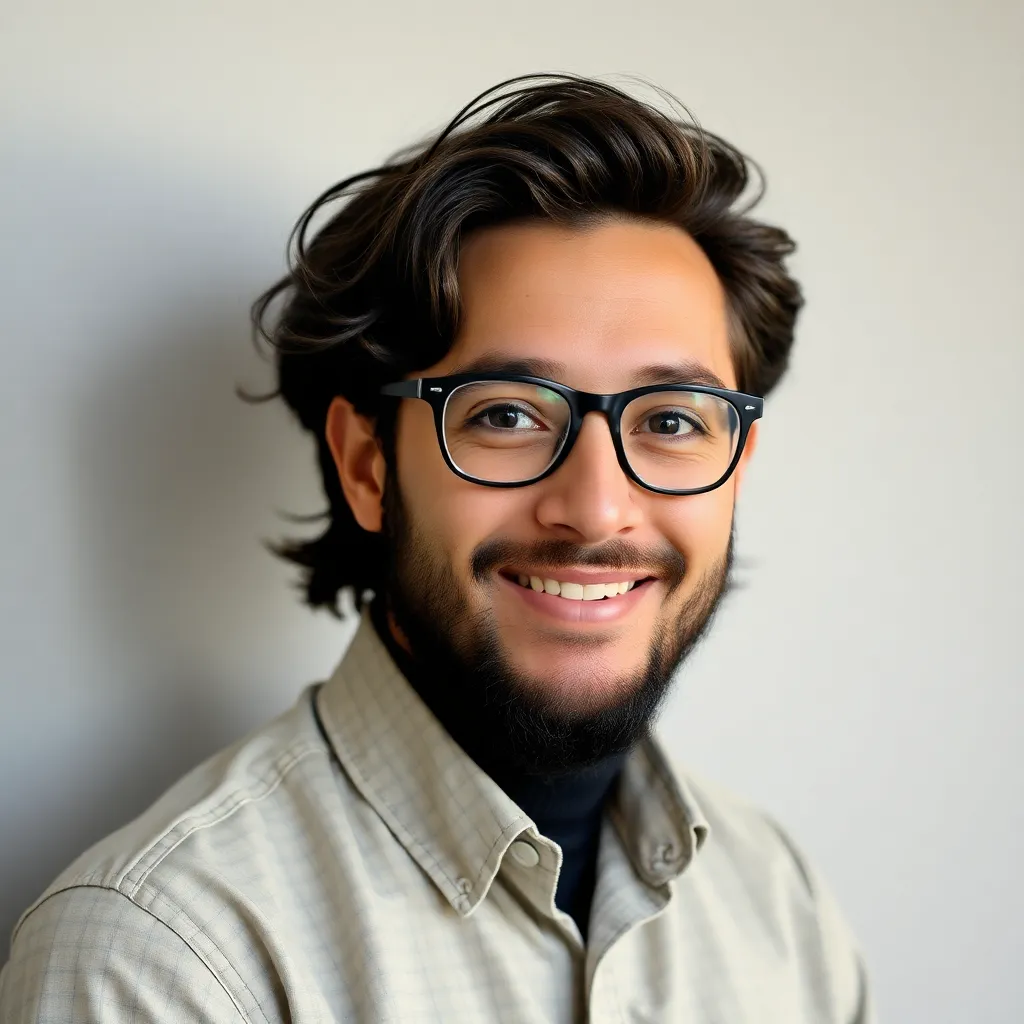
Holbox
Mar 24, 2025 · 6 min read
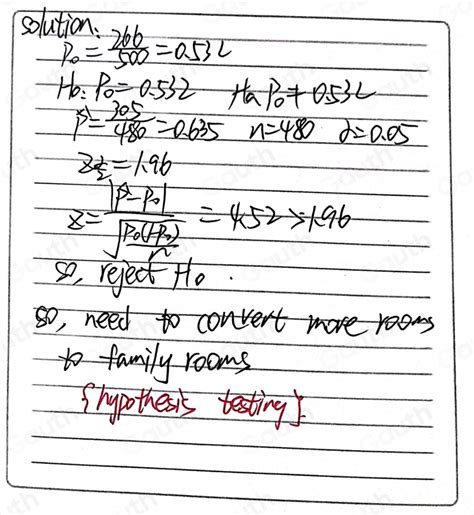
Table of Contents
- C Is Trying To Determine Whether To Convert
- Table of Contents
- C is Trying to Determine Whether to Convert: A Deep Dive into Data Type Conversion
- Implicit vs. Explicit Conversions: The Core Distinction
- Implicit Conversions (Type Coercion): The Automatic Approach
- Explicit Conversions (Casting): Taking Control
- Common Conversion Scenarios and Best Practices
- Integer to Floating-Point Conversions
- Floating-Point to Integer Conversions
- Character to Integer Conversions
- Pointers and Conversions
- Void Pointers
- Advanced Considerations: Type Promotion and Operator Precedence
- Debugging and Avoiding Conversion Errors
- Conclusion: Mastering Data Type Conversion in C
- Latest Posts
- Latest Posts
- Related Post
C is Trying to Determine Whether to Convert: A Deep Dive into Data Type Conversion
C, a powerful and widely used programming language, offers a flexible yet sometimes complex system for handling data type conversions. Understanding these conversions is crucial for writing efficient, bug-free, and maintainable C code. This article delves deep into the nuances of data type conversion in C, examining the different types of conversions, potential pitfalls, and best practices to ensure your code handles conversions safely and effectively.
Implicit vs. Explicit Conversions: The Core Distinction
C supports two primary methods for data type conversion: implicit and explicit. Understanding the differences between these approaches is fundamental to avoiding common errors.
Implicit Conversions (Type Coercion): The Automatic Approach
Implicit conversions, also known as type coercion, occur automatically when the compiler deems it necessary. This often happens when you mix different data types in an expression. The compiler implicitly promotes the "lower" data type to the "higher" data type to ensure the operation is performed correctly.
Example:
int x = 10;
float y = 2.5;
float z = x + y; // Implicit conversion of x (int) to float
In this example, the integer x
is implicitly converted to a float before being added to the float y
. The result z
is a float. The compiler handles this conversion automatically, without requiring explicit intervention from the programmer.
The typical implicit conversion hierarchy is as follows (from lowest to highest):
- char: Smallest integer type.
- short: Short integer.
- int: Standard integer.
- unsigned int: Unsigned integer.
- long: Long integer.
- unsigned long: Unsigned long integer.
- long long: Very long integer.
- unsigned long long: Unsigned very long integer.
- float: Single-precision floating-point number.
- double: Double-precision floating-point number.
- long double: Extended-precision floating-point number.
Potential Issues with Implicit Conversions:
While convenient, implicit conversions can introduce subtle bugs if not carefully considered. For example, truncation can occur when converting a larger type to a smaller type.
float a = 123.45;
int b = a; // Truncation: b will be 123, the fractional part is lost
Data loss is a common consequence of implicit narrowing conversions. Always be mindful of the potential for data loss when relying on implicit conversions.
Explicit Conversions (Casting): Taking Control
Explicit conversions, or casting, provide programmers with direct control over the data type transformation process. You explicitly tell the compiler to convert a value from one data type to another using parentheses.
Example:
float a = 123.45;
int b = (int)a; // Explicit conversion (casting) to int. Truncation still occurs
int c = 10;
float d = (float)c; //Explicit Conversion from int to float.
Explicit casting allows you to perform conversions that might not be automatically handled by the compiler. It's particularly useful when you need to control how a conversion is performed, or when you need to ensure a specific data type is used in a particular operation.
Advantages of Explicit Conversions:
- Clarity: Makes the programmer's intent explicit in the code, enhancing readability and maintainability.
- Control: Enables fine-grained control over the conversion process.
- Error Prevention: Can be used to prevent potential errors associated with implicit conversions.
Disadvantages of Explicit Conversions:
- Potential for Errors: Incorrect casting can lead to unexpected results or program crashes.
- Reduced Readability (Potentially): Overuse of casting can make code less readable, if not used judiciously.
Common Conversion Scenarios and Best Practices
Let's examine several common data type conversion scenarios and discuss best practices for each.
Integer to Floating-Point Conversions
Converting integers to floating-point types is generally straightforward. The integer value is converted to its floating-point representation. No data is lost, though precision might be limited by the floating-point type's capacity.
Best Practice: Use explicit casting for clarity, especially when dealing with larger integer types or when high precision is critical.
Floating-Point to Integer Conversions
Converting floating-point values to integers involves truncation (the fractional part is discarded). This can lead to data loss.
Best Practice: Always use explicit casting. Consider rounding functions (e.g., round()
, floor()
, ceil()
) if you need to control how the fractional part is handled.
Character to Integer Conversions
Characters in C are represented as integers, typically ASCII or Unicode values. Conversions between characters and integers are often implicit and seamless.
Best Practice: Be mindful of character encodings (ASCII, UTF-8, etc.) to avoid unexpected results.
Pointers and Conversions
Pointer conversions can be tricky and should be approached with caution. Converting between pointer types can be dangerous if not done correctly. The compiler might not catch errors related to incompatible pointer types, which can result in program crashes or unpredictable behavior.
Best Practice: Avoid unnecessary pointer conversions. When conversions are unavoidable, use explicit casting and carefully consider memory alignment and data structures.
Void Pointers
Void pointers (void *
) are generic pointers that can point to any data type. They're often used in functions that need to handle data of various types. However, converting a void *
to a specific pointer type requires explicit casting.
Best Practice: Always cast void *
pointers to their intended data type before dereferencing them.
Advanced Considerations: Type Promotion and Operator Precedence
Type promotion plays a crucial role in how C handles mixed-type expressions. The compiler might implicitly promote operands to a common type before performing an operation to ensure type consistency. Understanding operator precedence is also essential; it dictates the order of evaluation in an expression, which can influence the outcome of implicit conversions.
Example Illustrating Type Promotion:
int a = 5;
float b = 2.5;
float c = a + b; // a (int) is promoted to float before addition
Here, the int
variable a
is implicitly promoted to a float
before being added to b
.
Debugging and Avoiding Conversion Errors
Conversion errors can be notoriously difficult to track down. Here's how to approach debugging and prevention:
- Compiler Warnings: Pay close attention to compiler warnings about potential data loss or type mismatches.
- Static Analysis Tools: Utilize static analysis tools to identify potential conversion issues before runtime.
- Careful Code Review: Peer review is invaluable for spotting subtle conversion errors.
- Defensive Programming: Use explicit casting when necessary to make your intentions clear and reduce the risk of implicit conversions leading to unexpected results.
- Testing: Thoroughly test your code with various input values to identify conversion errors.
- Use Assertions: Use assertions to check the validity of data types and values after conversions, ensuring data integrity.
Conclusion: Mastering Data Type Conversion in C
Mastering data type conversions is a vital aspect of becoming a proficient C programmer. Understanding the differences between implicit and explicit conversions, common scenarios, and potential pitfalls is crucial for writing safe, reliable, and efficient code. By adhering to best practices, using explicit casting when appropriate, and employing defensive programming techniques, you can minimize the risks associated with data type conversions and build robust and maintainable C applications. Remember that clear code, with explicit intentions, is the best defense against unexpected behaviors stemming from data type conversions. Prioritize readability and careful planning to avoid common pitfalls and build robust and reliable C programs.
Latest Posts
Latest Posts
-
Which Of The Following Compounds Is Most Basic
Mar 26, 2025
-
Ethical Behavior At Work Is Learned By
Mar 26, 2025
-
The Us Government Has Subsidized Ethanol Production Since 1978
Mar 26, 2025
-
If You Suspect Information Has Been Improperly Or Unnecessarily Classified
Mar 26, 2025
-
The Terms Multiple Sclerosis And Atherosclerosis Are Similar
Mar 26, 2025
Related Post
Thank you for visiting our website which covers about C Is Trying To Determine Whether To Convert . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.