An Example Of A Control Statement Is:
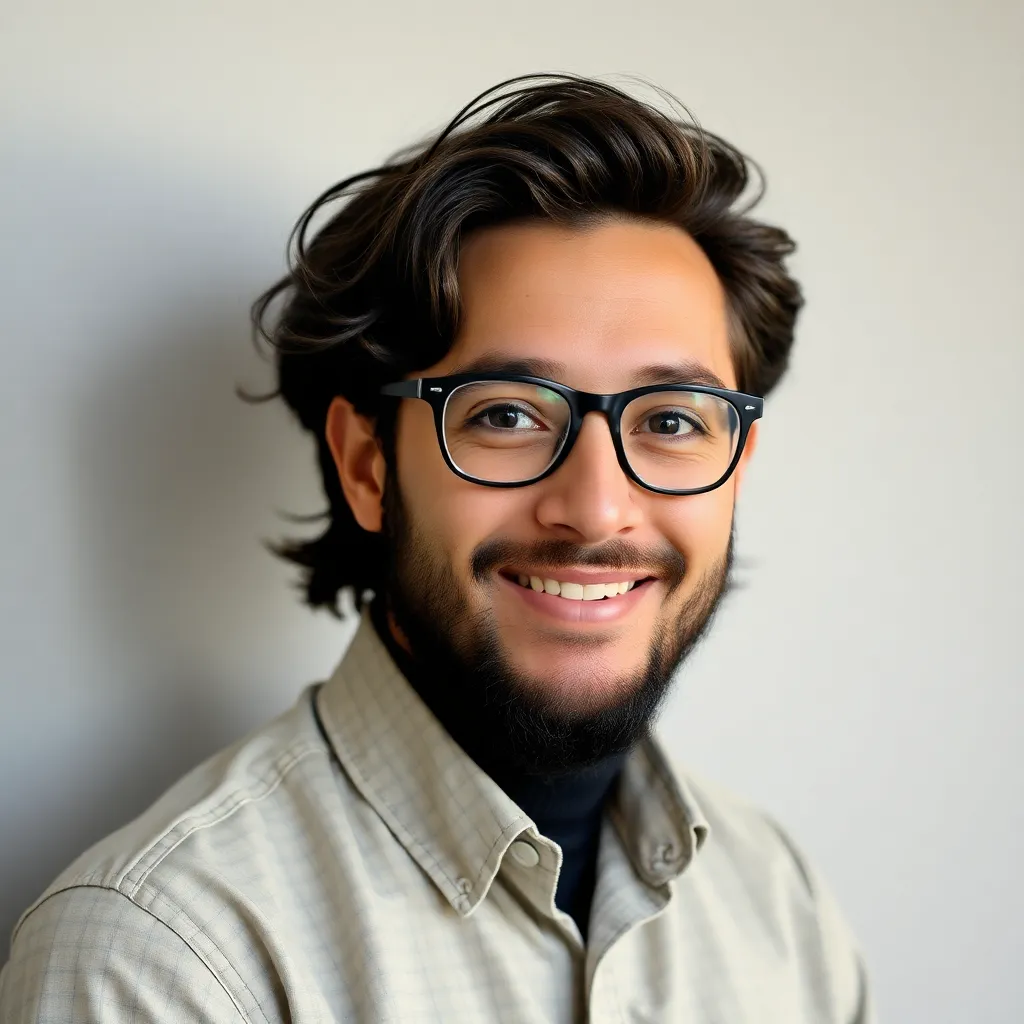
Holbox
Mar 18, 2025 · 6 min read
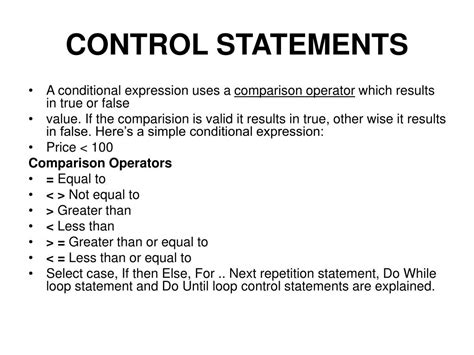
Table of Contents
An Example of a Control Statement is: Exploring Conditional Logic and Program Flow
Control statements are the backbone of any programming language. They dictate the flow of execution, determining which parts of a program are executed and in what order. Understanding control statements is crucial for writing efficient, robust, and readable code. This article will delve deep into the world of control statements, using illustrative examples in Python to explain their functionality and importance. We'll cover various types, including if
, elif
, else
, for
, while
, and break
and continue
statements, highlighting their practical applications and demonstrating how they are fundamental to building complex programs.
What are Control Statements?
Control statements, also known as control structures or control flow statements, are programming constructs that allow you to control the order in which statements are executed within a program. Without them, your code would simply execute line by line, from top to bottom, severely limiting its capabilities. Control statements enable you to create dynamic and responsive programs that can handle different scenarios and make decisions based on various conditions.
Think of it like this: Imagine a cookbook recipe. A simple recipe might just list ingredients and steps sequentially. But a sophisticated recipe might include instructions like "If the dough is too sticky, add more flour," or "Repeat steps 3-5 until the mixture is smooth." These "if" and "repeat" instructions are analogous to control statements in programming. They allow the cook (the program) to adapt to changing circumstances (data) and achieve the desired outcome (the program's goal).
Conditional Statements: Making Decisions
The most fundamental type of control statement is the conditional statement. Conditional statements allow your program to execute different blocks of code based on whether a specific condition is true or false. In most programming languages, this is achieved using if
, elif
(else if), and else
keywords.
The if
Statement
The simplest form is the if
statement. It executes a block of code only if a condition evaluates to True
.
temperature = 25
if temperature > 20:
print("It's a warm day!")
In this example, the print
statement will only be executed if the variable temperature
is greater than 20.
The if-else
Statement
To handle both true and false cases, we use the if-else
statement.
age = 15
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
This code checks if age
is greater than or equal to 18. If it is, the first message is printed; otherwise, the second message is printed. One and only one of these blocks will execute.
The if-elif-else
Statement
For handling multiple conditions, the if-elif-else
statement provides a structured approach.
grade = 85
if grade >= 90:
print("A")
elif grade >= 80:
print("B")
elif grade >= 70:
print("C")
else:
print("F")
This example assigns letter grades based on numerical scores. The code evaluates each condition sequentially. As soon as a condition is met, the corresponding block is executed, and the rest are skipped. If none of the conditions are met, the else
block is executed.
Looping Statements: Repetition Made Easy
Looping statements allow you to execute a block of code repeatedly. This is incredibly useful when dealing with collections of data or when you need to perform a task multiple times. The two main types of loops are for
and while
loops.
The for
Loop
The for
loop is typically used to iterate over a sequence (like a list, tuple, or string) or other iterable object.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
This loop iterates through the fruits
list, printing each fruit name.
Iterating with Range: You can also use the range()
function to create a sequence of numbers for iteration.
for i in range(5): # Iterates from 0 to 4
print(i)
The while
Loop
The while
loop repeatedly executes a block of code as long as a specified condition remains true.
count = 0
while count < 5:
print(count)
count += 1
This loop continues to print the value of count
and increment it until count
becomes 5 (at which point the condition count < 5
becomes false). Caution: Be careful to avoid infinite loops by ensuring your loop condition eventually becomes false.
Breaking and Continuing Loops: Fine-Grained Control
The break
and continue
statements offer more granular control over loop execution.
The break
Statement
The break
statement immediately terminates the loop, regardless of whether the loop condition is still true.
for i in range(10):
if i == 5:
break
print(i)
This loop will stop when i
reaches 5, and the remaining iterations will not be executed.
The continue
Statement
The continue
statement skips the rest of the current iteration and proceeds to the next iteration of the loop.
for i in range(10):
if i % 2 == 0: # Skip even numbers
continue
print(i)
This loop will print only odd numbers because the continue
statement skips the even numbers.
Nested Control Statements: Building Complexity
Control statements can be nested within each other to create more complex program logic. This allows for intricate decision-making and iterative processes.
for i in range(3):
for j in range(2):
print(f"i = {i}, j = {j}")
This code demonstrates nested for
loops. The inner loop (with j
) completes all its iterations for each iteration of the outer loop (with i
).
Practical Applications of Control Statements
Control statements are fundamental to many programming tasks. Here are a few examples:
- Data Validation: Checking user input to ensure it meets certain criteria.
- Error Handling: Handling exceptions and preventing program crashes.
- Game Development: Controlling game flow, responding to player actions.
- Web Development: Creating dynamic web pages that respond to user interactions.
- Data Processing: Filtering and manipulating large datasets.
- Algorithm Design: Implementing algorithms that involve decisions and repetitions.
Conclusion: Mastering Control Flow
Control statements are the building blocks of any program's logic. By mastering their use, you gain the ability to write programs that are not only functional but also elegant, efficient, and easily maintainable. This article has provided a comprehensive overview of common control statements, demonstrating their usage with practical examples in Python. Remember to practice regularly, experimenting with different scenarios and complexities to solidify your understanding and develop your programming skills. As you progress, you'll find that the creative application of these fundamental tools is key to designing powerful and sophisticated software applications. The ability to effectively manage program flow through the skillful use of control structures is a cornerstone of successful programming.
Latest Posts
Latest Posts
-
Use Retrosynthetic Analysis To Suggest A Way
Mar 18, 2025
-
Notch Is A Receptor Protein Displayed On The Surface
Mar 18, 2025
-
Which Of The Following Applies To The Electron
Mar 18, 2025
-
Electrolytes In Body Fluids Report Sheet
Mar 18, 2025
-
Industrial Services Are Support Products That Include Items Such As
Mar 18, 2025
Related Post
Thank you for visiting our website which covers about An Example Of A Control Statement Is: . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.