Write An Iterative Version Of Randomized_select
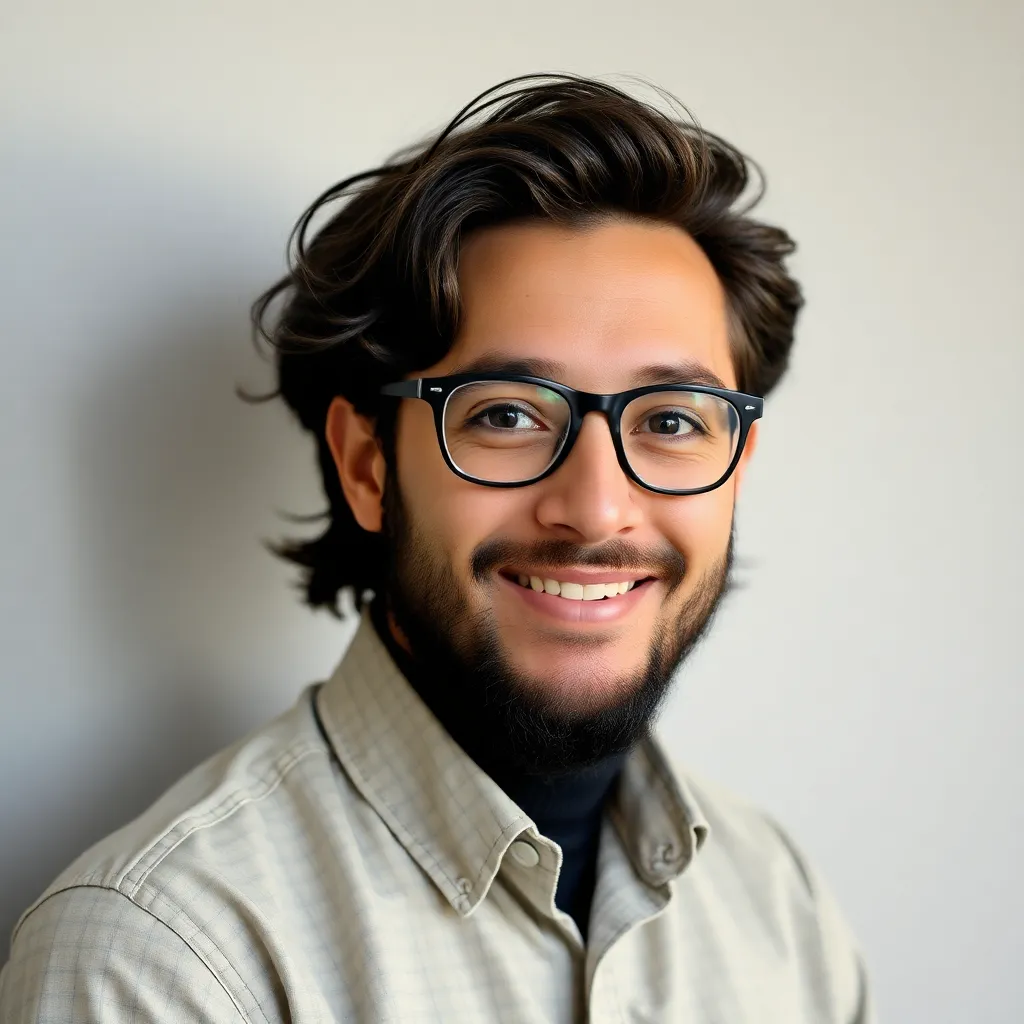
Holbox
Mar 19, 2025 · 6 min read
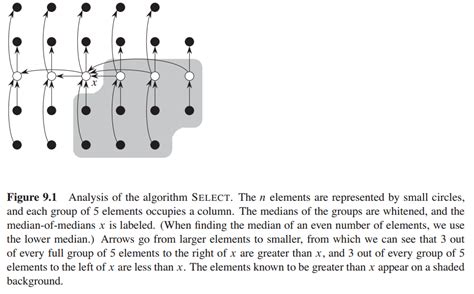
Table of Contents
An Iterative Version of Randomized Select
Randomized select is a powerful algorithm for finding the i-th smallest element in an unordered array. Its average-case linear time complexity makes it significantly faster than sorting-based approaches for this specific problem. While the recursive implementation is often presented first due to its elegance and clear mirroring of the algorithm's logic, an iterative version offers benefits in terms of space complexity and potential performance improvements in certain scenarios. This article will delve into the intricacies of designing and implementing an iterative version of randomized select, exploring its advantages and disadvantages compared to its recursive counterpart.
Understanding Randomized Select: A Quick Recap
Before diving into the iterative implementation, let's briefly revisit the core concepts of randomized select. The algorithm leverages the power of random partitioning to efficiently pinpoint the desired element.
The algorithm's steps are as follows:
-
Random Pivot Selection: A pivot element is randomly selected from the input array.
-
Partitioning: The array is partitioned around the chosen pivot, such that elements smaller than the pivot are placed before it, and elements larger than the pivot are placed after it. This partitioning step is crucial for the algorithm's efficiency.
-
Recursive Calls (or Iterative Steps):
- If the pivot's position is equal to i, the pivot is the i-th smallest element, and the algorithm terminates.
- If the pivot's position is greater than i, the search continues recursively (or iteratively) in the subarray to the left of the pivot.
- If the pivot's position is less than i, the search continues recursively (or iteratively) in the subarray to the right of the pivot.
The algorithm's efficiency stems from the fact that, on average, each recursive call reduces the problem size by a constant factor. This leads to an average-case time complexity of O(n), where n is the size of the input array.
The Need for an Iterative Approach
While the recursive implementation is conceptually straightforward and easy to understand, it has limitations:
-
Stack Space: Recursive calls consume stack space. For extremely large arrays, this could lead to stack overflow errors. An iterative approach avoids this problem entirely.
-
Tail Recursion Optimization: While some compilers optimize tail recursion, this isn't universally guaranteed. An iterative version eliminates any reliance on tail recursion optimization.
-
Potential Performance Gains: In some specific scenarios, the overhead associated with function calls in recursion might outweigh the benefits, making an iterative implementation marginally faster. This difference is typically small but could be significant in performance-critical applications.
Designing the Iterative Randomized Select
The core challenge in converting the recursive algorithm to an iterative one lies in managing the subarray boundaries and tracking the search progress without the implicit stack management of recursion. We'll use a while
loop and carefully maintain indices to mimic the recursive calls.
Here's a pseudocode outline of the iterative algorithm:
iterative_randomized_select(arr, i):
low = 0
high = length(arr) - 1
while low <= high:
pivot_index = random_partition(arr, low, high)
if pivot_index == i - 1:
return arr[pivot_index]
elif pivot_index > i - 1:
high = pivot_index - 1
else:
low = pivot_index + 1
return -1 //Should never reach here in a correctly implemented algorithm.
The random_partition
function remains largely unchanged from the recursive version: it randomly selects a pivot, partitions the array around the pivot, and returns the pivot's index.
Implementing random_partition
The random_partition
function is vital for the algorithm's efficiency. It should, on average, divide the array into roughly equal halves. Here's a possible implementation:
import random
def random_partition(arr, low, high):
"""Partitions the array around a randomly chosen pivot."""
random_pivot_index = random.randint(low, high)
arr[low], arr[random_pivot_index] = arr[random_pivot_index], arr[low] #swap pivot to the beginning
pivot = arr[low]
i = low + 1
for j in range(low + 1, high + 1):
if arr[j] < pivot:
arr[i], arr[j] = arr[j], arr[i]
i += 1
arr[low], arr[i - 1] = arr[i - 1], arr[low] #swap pivot to its correct position
return i - 1
This implementation uses the Lomuto partitioning scheme, which is relatively simple to implement. Other partitioning schemes (like Hoare's scheme) could also be used, potentially offering slight performance improvements.
Complete Iterative Python Code
Putting it all together, here’s the complete Python code for the iterative randomized select algorithm:
import random
def random_partition(arr, low, high):
# ... (Implementation from above) ...
def iterative_randomized_select(arr, i):
low = 0
high = len(arr) - 1
while low <= high:
pivot_index = random_partition(arr, low, high)
if pivot_index == i - 1:
return arr[pivot_index]
elif pivot_index > i - 1:
high = pivot_index - 1
else:
low = pivot_index + 1
return -1 # Should not reach here in a correct implementation
# Example usage:
arr = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
i = 5 #Find the 5th smallest element
result = iterative_randomized_select(arr, i)
print(f"The {i}th smallest element is: {result}")
arr2 = [1,2,3,4,5,6,7,8,9,10]
i2 = 5
result2 = iterative_randomized_select(arr2,i2)
print(f"The {i2}th smallest element is: {result2}")
arr3 = [10,9,8,7,6,5,4,3,2,1]
i3 = 5
result3 = iterative_randomized_select(arr3,i3)
print(f"The {i3}th smallest element is: {result3}")
Advantages and Disadvantages of the Iterative Version
Advantages:
- No Stack Overflow: The iterative approach eliminates the risk of stack overflow errors, making it suitable for processing extremely large datasets.
- Deterministic Space Complexity: The space complexity is O(1), regardless of the input size, offering a significant advantage over the recursive version's O(log n) space complexity in the average case (due to recursive call stack).
- Potential Performance Improvements: In some situations, the elimination of function call overhead can lead to slight performance gains, although this is often negligible compared to the dominant O(n) time complexity.
Disadvantages:
- Increased Complexity: The iterative implementation is generally more complex and less intuitive to understand than the recursive version. The code is slightly less readable and requires more careful management of indices.
- Minor Performance Differences: The performance difference between the iterative and recursive versions is usually marginal. The recursive version's elegance and readability often outweigh the minuscule performance gains of iteration in most practical applications.
Conclusion
The iterative version of randomized select provides a robust alternative to the commonly used recursive implementation. While the recursive version offers greater elegance and readability, the iterative approach offers significant advantages in terms of space complexity and robustness for extremely large datasets, eliminating the risk of stack overflow. The choice between the two depends on the specific application's requirements and priorities. For most cases, the recursive version is perfectly adequate, but for situations demanding absolute control over memory usage or dealing with exceptionally large arrays, the iterative version shines. Understanding both approaches allows for informed decision-making based on the constraints and goals of a given project.
Latest Posts
Latest Posts
-
Select The Correct Answer From The Drop Down Menu
Mar 20, 2025
-
To Brake Safely While Traveling Downhill
Mar 20, 2025
-
What Should You Assess Regardless Of Age Group
Mar 20, 2025
-
Assignment 7 Project Stem Leapp Year
Mar 20, 2025
-
Work For The Same Employer And Often Have Lunch Together
Mar 20, 2025
Related Post
Thank you for visiting our website which covers about Write An Iterative Version Of Randomized_select . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.