Which Option Rotates The Square 90 Degrees
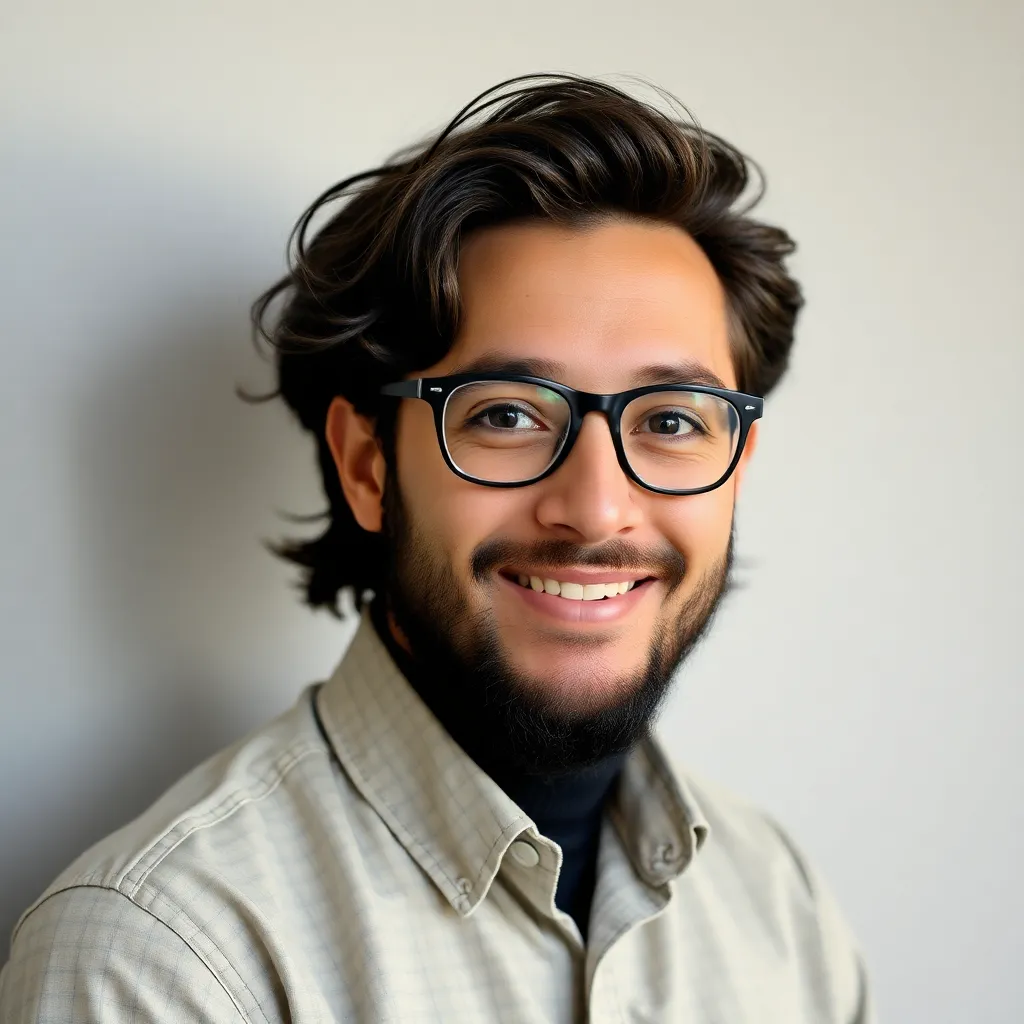
Holbox
May 09, 2025 · 5 min read

Table of Contents
- Which Option Rotates The Square 90 Degrees
- Table of Contents
- Which Option Rotates the Square 90 Degrees? A Deep Dive into 2D Transformations
- Understanding 2D Rotation
- The Role of the Rotation Center
- Clockwise vs. Counter-clockwise Rotation
- Method 1: Rotation Matrix Transformation
- The Rotation Matrix
- Applying the Transformation
- Method 2: Quaternion Rotation (for 3D extension)
- Quaternion Representation
- Applying the Quaternion Rotation
- Method 3: Coordinate System Transformation
- Transforming the Coordinates
- Method 4: Programming Implementation (Illustrative Example)
- Choosing the Right Method
- Conclusion: Mastering 2D Rotation
- Latest Posts
- Latest Posts
- Related Post
Which Option Rotates the Square 90 Degrees? A Deep Dive into 2D Transformations
Rotating a square 90 degrees might seem like a simple task, but understanding the underlying principles of 2D transformations is crucial for various applications, from game development and computer graphics to robotics and engineering. This comprehensive guide explores different methods for achieving a 90-degree rotation of a square, examining their mathematical foundations and practical implications. We'll delve into matrix transformations, quaternion rotations, and even consider alternative approaches involving coordinate system changes.
Understanding 2D Rotation
Before diving into specific methods, let's establish a common understanding of 2D rotation. A 90-degree rotation implies turning the square by a right angle around a specific point, often its center. This transformation alters the coordinates of each vertex of the square. The choice of rotation (clockwise or counter-clockwise) also impacts the final orientation.
The Role of the Rotation Center
The point around which the rotation occurs significantly affects the result. Rotating around the center of the square keeps its position unchanged while changing its orientation. Rotating around a vertex will cause both a change in orientation and a change in position. Rotating around an arbitrary point external to the square will result in a complex transformation that combines both rotation and translation.
Clockwise vs. Counter-clockwise Rotation
The direction of rotation—clockwise or counter-clockwise—is another critical aspect. A clockwise rotation of 90 degrees is different from a counter-clockwise rotation of 90 degrees. This difference is reflected in the mathematical formulas and transformation matrices used to achieve the rotation.
Method 1: Rotation Matrix Transformation
The most common and mathematically elegant method for rotating a square (or any 2D object) is using a rotation matrix. This method leverages linear algebra to define the transformation concisely.
The Rotation Matrix
For a 90-degree counter-clockwise rotation around the origin (0, 0), the rotation matrix is:
R(90°) = | cos(90°) -sin(90°) | = | 0 -1 |
| sin(90°) cos(90°) | | 1 0 |
For a 90-degree clockwise rotation:
R(-90°) = | cos(-90°) -sin(-90°) | = | 0 1 |
| sin(-90°) cos(-90°) | | -1 0 |
Applying the Transformation
To rotate a point (x, y) using the rotation matrix, we perform matrix multiplication:
| x' | | 0 -1 | | x |
| y' | = | 1 0 | * | y |
This results in the new coordinates (x', y') = (-y, x) for a counter-clockwise rotation and (y, -x) for a clockwise rotation. This process is repeated for each vertex of the square.
Method 2: Quaternion Rotation (for 3D extension)
While not strictly necessary for a 2D square rotation, understanding quaternion rotations is crucial if you intend to extend this to 3D transformations. Quaternions provide a more robust and efficient way to handle rotations in three dimensions, avoiding issues like gimbal lock that can occur with Euler angles.
Quaternion Representation
A quaternion is represented as q = w + xi + yj + zk, where w, x, y, and z are real numbers, and i, j, k are imaginary units with specific multiplication rules. For a 90-degree rotation around the z-axis (in 3D space, which corresponds to a 2D rotation around the origin in the xy-plane), the quaternion would be:
q = cos(45°) + sin(45°)k
Applying the Quaternion Rotation
Rotating a point using a quaternion involves converting the point to a pure quaternion (with w=0), then performing quaternion multiplication to obtain the rotated point. This involves more complex calculations than matrix transformations but offers advantages in 3D scenarios.
Method 3: Coordinate System Transformation
Another approach involves changing the coordinate system. Instead of rotating the square, we can rotate the coordinate system itself. This conceptually achieves the same result.
Transforming the Coordinates
If we rotate the coordinate system by 90 degrees counter-clockwise, the square's coordinates relative to the new coordinate system will appear as if the square itself was rotated clockwise by 90 degrees relative to the original coordinate system. The mathematical formulas involved would be similar to those used in matrix transformations, but the interpretation is different.
Method 4: Programming Implementation (Illustrative Example)
Let's consider a simplified Python example demonstrating the rotation matrix approach:
import numpy as np
def rotate_point(x, y, angle_degrees):
angle_radians = np.radians(angle_degrees)
rotation_matrix = np.array([[np.cos(angle_radians), -np.sin(angle_radians)],
[np.sin(angle_radians), np.cos(angle_radians)]])
point = np.array([x, y])
rotated_point = np.dot(rotation_matrix, point)
return rotated_point
# Example usage: Rotating a point (1, 0) 90 degrees counter-clockwise
x, y = 1, 0
rotated_x, rotated_y = rotate_point(x, y, 90)
print(f"Original point: ({x}, {y}), Rotated point: ({rotated_x}, {rotated_y})") #Output: (0.0, 1.0)
#Rotating a square: (needs to be adapted for all 4 vertices)
square_vertices = [(0,0), (1,0), (1,1), (0,1)]
rotated_square = []
for x,y in square_vertices:
rotated_x, rotated_y = rotate_point(x,y, 90)
rotated_square.append((rotated_x, rotated_y))
print(f"Rotated Square vertices:{rotated_square}") #Output: [(0.0, 0.0), (0.0, 1.0), (-1.0, 1.0), (-1.0, 0.0)]
This code snippet provides a basic illustration. A complete implementation would involve handling multiple vertices and potentially incorporating visualization libraries for graphical representation.
Choosing the Right Method
The optimal method depends on the specific application and context.
- Rotation Matrix: Most efficient and widely used for 2D rotations due to its simplicity and computational efficiency.
- Quaternion: Preferred for 3D rotations and situations requiring more complex rotational sequences.
- Coordinate System Transformation: Offers a different perspective on the problem, useful for understanding the underlying concepts.
Remember to consider the rotation center and the direction (clockwise or counter-clockwise) when implementing any of these methods. The choice of implementation language and libraries will also affect the specific details of the code.
Conclusion: Mastering 2D Rotation
Mastering 2D rotation is fundamental to numerous fields. By understanding the underlying mathematical principles and different approaches—rotation matrices, quaternions, and coordinate system transformations—you can effectively manipulate 2D objects and build sophisticated applications. This comprehensive guide provides a strong foundation for tackling more complex geometric transformations in the future. Remember to practice and experiment with different methods to solidify your understanding. The provided Python example is a starting point for hands-on exploration. Further exploration into libraries such as OpenGL or libraries within game engines will provide additional tools to visualize and manipulate these rotations.
Latest Posts
Latest Posts
-
How Many Cm In 58 Inches
May 21, 2025
-
How Many Inches Are 18 Cm
May 21, 2025
-
What Is 40 Kg In Stone
May 21, 2025
-
What Is 79 Cm In Inches
May 21, 2025
-
137 Cm To Inches And Feet
May 21, 2025
Related Post
Thank you for visiting our website which covers about Which Option Rotates The Square 90 Degrees . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.