Which Of The Following Is An Example Of A Parameter
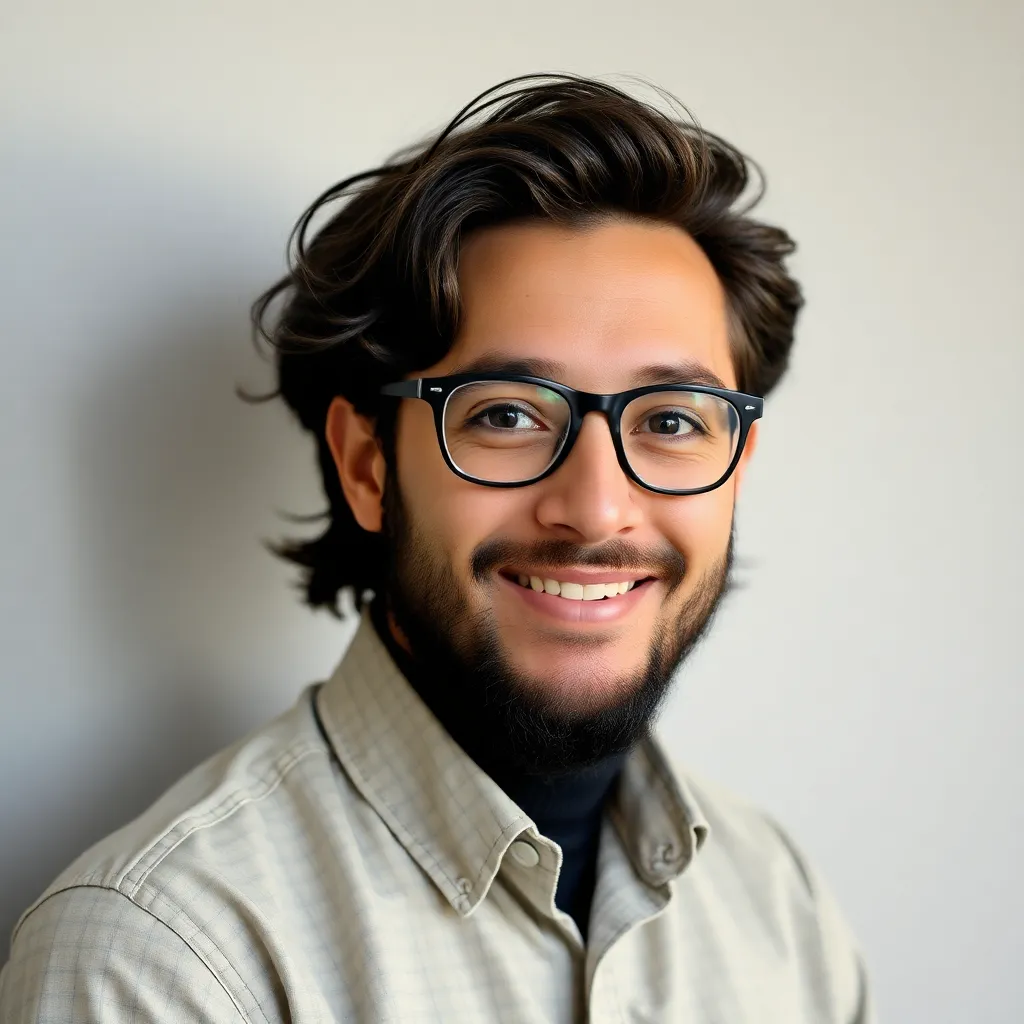
Holbox
May 07, 2025 · 5 min read
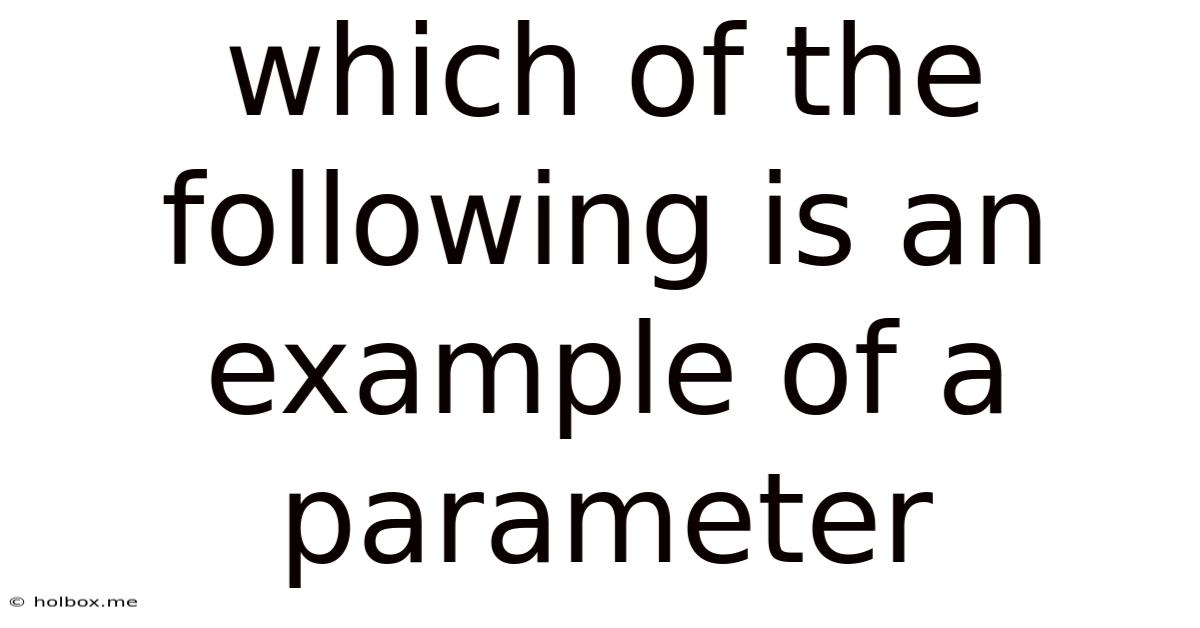
Table of Contents
- Which Of The Following Is An Example Of A Parameter
- Table of Contents
- Which of the following is an example of a parameter? Understanding Parameters in Programming
- What are Parameters?
- Parameters vs. Arguments: A Crucial Distinction
- Types of Parameters
- 1. Positional Parameters:
- 2. Keyword Parameters:
- 3. Default Parameters:
- 4. Variable-Length Positional Parameters (*args):
- 5. Variable-Length Keyword Parameters (**kwargs):
- Examples of Parameters in Different Programming Contexts
- 1. Mathematical Functions:
- 2. String Manipulation:
- 3. Data Processing:
- 4. File Handling:
- 5. Database Interactions:
- Best Practices for Using Parameters
- Conclusion
- Latest Posts
- Related Post
Which of the following is an example of a parameter? Understanding Parameters in Programming
Parameters are fundamental to programming, representing the crucial link between a function's definition and its execution. They act as placeholders for values that a function needs to perform its task. Understanding parameters is crucial for writing clean, reusable, and efficient code. This article dives deep into the concept of parameters, explaining what they are, how they differ from arguments, and providing numerous examples to solidify your understanding. We'll also explore various types of parameters and their applications.
What are Parameters?
In simple terms, parameters are variables listed within the parentheses of a function's definition. They are essentially named placeholders that receive values when the function is called. These values are then used internally by the function to achieve its intended purpose. Think of them as the function's inputs – the raw materials it needs to work with. A function can have zero, one, or many parameters, depending on its design and functionality.
Parameters vs. Arguments: A Crucial Distinction
Often, the terms "parameter" and "argument" are used interchangeably, leading to confusion. However, there's a key distinction:
- Parameters: Defined within the function's declaration. They act as placeholders.
- Arguments: The actual values passed to the function when it's called. They are the concrete data the function processes.
Consider the following Python example:
def greet(name): # 'name' is a parameter
print(f"Hello, {name}!")
greet("Alice") # "Alice" is an argument
In this code snippet, name
is a parameter in the function definition, while "Alice" is the argument passed to the function during its invocation. The function greet
uses the argument "Alice" to personalize the greeting.
Types of Parameters
Programming languages offer various ways to define parameters, each with its own characteristics and use cases:
1. Positional Parameters:
These are the most common type of parameters. Their order matters. The values passed as arguments are assigned to the parameters based on their position in the function call.
def add(x, y):
return x + y
result = add(5, 3) # 5 is assigned to x, 3 is assigned to y
print(result) # Output: 8
If you change the order of the arguments, the result will also change.
2. Keyword Parameters:
Keyword parameters allow you to specify the argument values explicitly using parameter names. This makes the code more readable and less prone to errors, especially when dealing with functions having many parameters.
def describe_pet(animal_type, pet_name):
print(f"\nI have a {animal_type}.")
print(f"My {animal_type}'s name is {pet_name.title()}.")
describe_pet(animal_type='hamster', pet_name='harry')
describe_pet(pet_name='willie', animal_type='dog')
Notice how the order of the arguments doesn't matter in this case because we explicitly specify which parameter each value should be assigned to.
3. Default Parameters:
Default parameters assign a default value to a parameter. If a value for that parameter isn't provided during the function call, the default value is used. This enhances code flexibility and readability.
def greet(name, greeting="Hello"):
print(f"{greeting}, {name}!")
greet("Bob") # Output: Hello, Bob!
greet("Charlie", "Good morning") # Output: Good morning, Charlie!
Here, if the greeting
argument isn't provided, "Hello" is used. However, you can override the default value by providing a different argument.
4. Variable-Length Positional Parameters (*args):
The *args
parameter allows a function to accept a variable number of positional arguments. These arguments are collected into a tuple. This is useful when you don't know in advance how many arguments will be passed.
def sum_all(*args):
total = 0
for number in args:
total += number
return total
print(sum_all(1, 2, 3)) # Output: 6
print(sum_all(10, 20, 30, 40)) # Output: 100
5. Variable-Length Keyword Parameters (**kwargs):
Similar to *args
, **kwargs
allows a function to accept a variable number of keyword arguments. These arguments are collected into a dictionary.
def print_details(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
print_details(name="Alice", age=30, city="New York")
This would print:
name: Alice
age: 30
city: New York
Examples of Parameters in Different Programming Contexts
Let's examine how parameters are used in various programming scenarios:
1. Mathematical Functions:
Parameters represent the inputs to mathematical operations.
def calculate_area(length, width):
return length * width
area = calculate_area(5, 10) # length and width are parameters
2. String Manipulation:
Parameters define the strings to be manipulated.
def concatenate_strings(str1, str2):
return str1 + str2
result = concatenate_strings("Hello", "World") #str1 and str2 are parameters
3. Data Processing:
Parameters specify the data sets to be processed.
def process_data(data, function):
processed_data = [function(x) for x in data]
return processed_data
4. File Handling:
Parameters can represent file paths or modes.
def read_file(filepath):
with open(filepath, 'r') as file:
contents = file.read()
return contents
5. Database Interactions:
Parameters are used to pass query parameters to a database.
#Illustrative example - specific syntax varies across database systems.
def fetch_data(query, parameters):
# Execute SQL query with parameters
# ... code to interact with the database ...
return data
Best Practices for Using Parameters
- Use descriptive names: Choose names that clearly indicate the purpose of each parameter.
- Maintain consistency: Follow a consistent naming convention throughout your codebase.
- Document parameters: Clearly document the purpose and expected data type of each parameter using docstrings or comments.
- Validate parameters: Check the validity of parameters before using them to prevent unexpected errors.
- Avoid excessive parameters: If a function has too many parameters, consider refactoring it into smaller, more manageable functions.
Conclusion
Parameters are fundamental building blocks of functions in any programming language. Understanding their different types, how they interact with arguments, and best practices for their usage is crucial for writing robust, efficient, and maintainable code. By mastering the art of using parameters effectively, you can significantly improve the quality and readability of your programs. Remember the distinction between parameters (placeholders) and arguments (actual values) and leverage the power of keyword parameters, default parameters, and variable-length parameters to write flexible and adaptable code. Through consistent application of these principles, you'll build a strong foundation for creating sophisticated and efficient applications.
Latest Posts
Related Post
Thank you for visiting our website which covers about Which Of The Following Is An Example Of A Parameter . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.