Which Of The Following Are Common To All Programming Languages
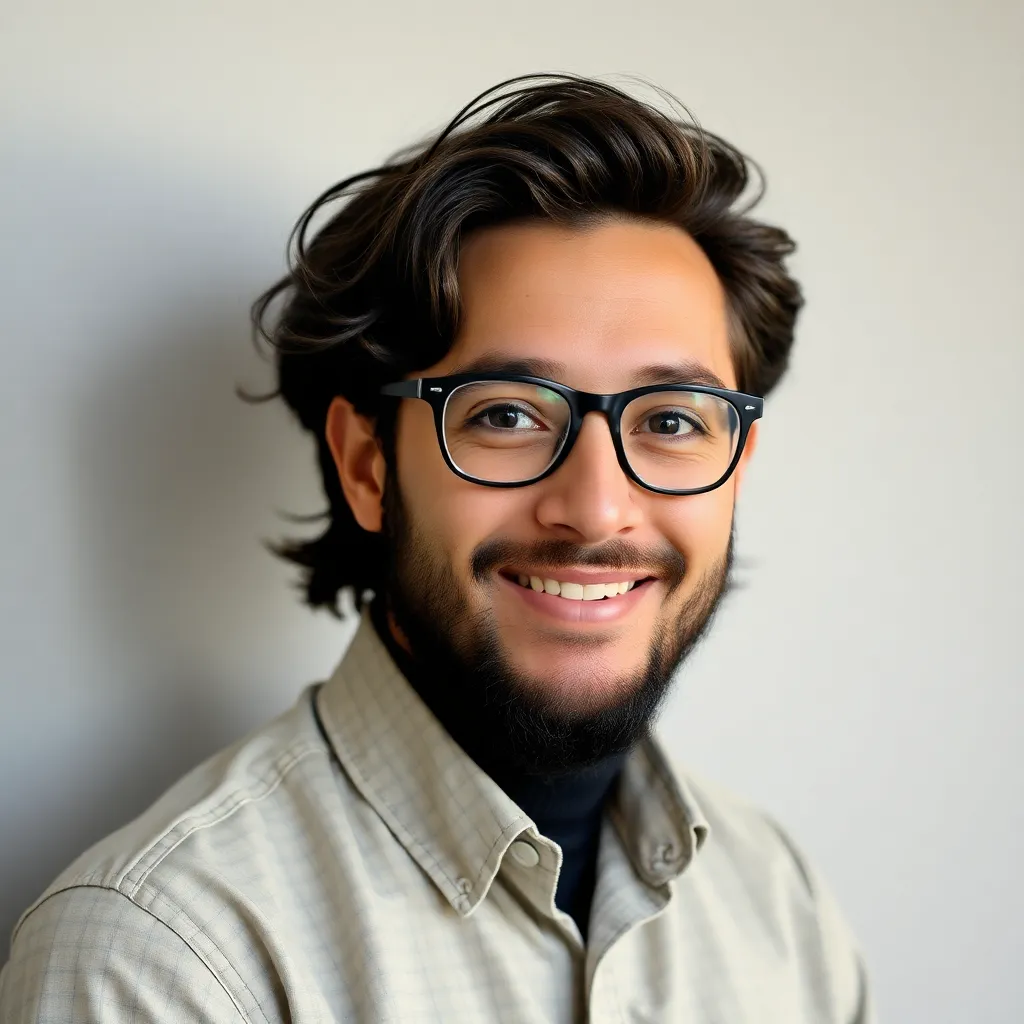
Holbox
May 12, 2025 · 6 min read
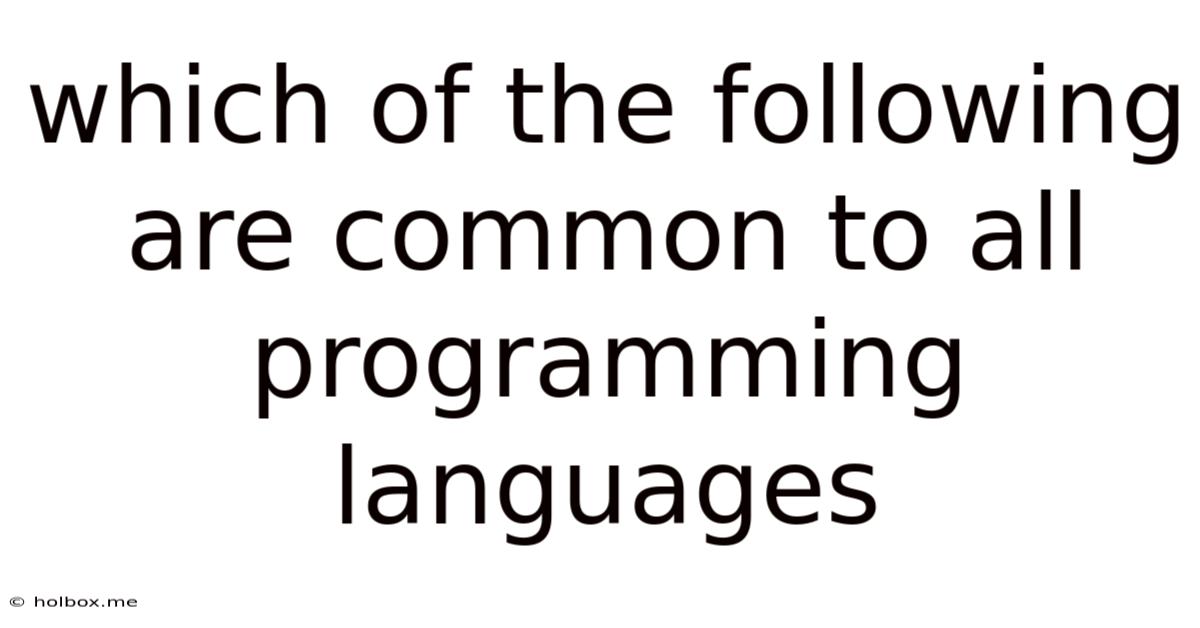
Table of Contents
- Which Of The Following Are Common To All Programming Languages
- Table of Contents
- Which of the following are common to all programming languages?
- Fundamental Building Blocks: Common to All Programming Languages
- 1. Syntax and Semantics: The Language's Rules
- 2. Data Types: Defining Information
- 3. Variables and Constants: Storing Data
- 4. Operators: Performing Operations
- 5. Control Structures: Managing Program Flow
- 6. Functions/Procedures/Methods: Modularizing Code
- 7. Input/Output (I/O): Interacting with the World
- 8. Comments: Enhancing Readability
- 9. Error Handling and Exception Management: Robustness
- 10. Memory Management: Resource Allocation and Deallocation
- Beyond the Fundamentals: Higher-Level Concepts Shared Across Languages
- Conclusion: The Unifying Principles of Programming Languages
- Latest Posts
- Related Post
Which of the following are common to all programming languages?
Programming languages, despite their diversity and unique features, share a fundamental set of commonalities. While the syntax and specific functionalities differ significantly across languages like Python, Java, C++, JavaScript, and more, certain core elements remain consistent. Understanding these commonalities is crucial for any programmer, irrespective of their preferred language. This article will delve deep into these shared characteristics, exploring their significance and how they form the bedrock of all programming.
Fundamental Building Blocks: Common to All Programming Languages
Several key elements act as the foundation upon which all programming languages are built. These include:
1. Syntax and Semantics: The Language's Rules
Every programming language has a syntax, a set of rules that govern how code is written. Think of it as the language's grammar. This includes things like how to declare variables, define functions, use operators, and structure statements. While the specific syntax varies drastically (e.g., int x = 5;
in C++ vs. x = 5
in Python), the underlying concept of structured code remains.
Semantics, on the other hand, defines the meaning of the code. It dictates what each statement or instruction does. For instance, the +
operator generally signifies addition across most languages, but the specific way it handles different data types might differ. A deep understanding of both syntax and semantics is essential to write error-free and functional code in any language.
Keywords: All languages utilize keywords, reserved words with special meanings within the language's context. These keywords cannot be used as variable or function names. Examples include if
, else
, for
, while
, function
, class
, etc. While their specific spellings vary, the underlying functionality remains consistent. For example, the if
statement is used for conditional logic in almost all programming languages.
2. Data Types: Defining Information
Programming languages need a way to represent and manipulate data. This is achieved through data types. These categorize data based on its kind and how it's stored in memory. Common data types include:
- Integers: Whole numbers (e.g., 5, -10, 0).
- Floating-point numbers: Numbers with decimal points (e.g., 3.14, -2.5).
- Booleans: True/False values representing logical states.
- Characters: Single letters, symbols, or numbers.
- Strings: Sequences of characters (e.g., "Hello, world!").
- Arrays/Lists: Ordered collections of data elements.
While specific implementations differ, the fundamental concept of classifying and managing data based on type is universal.
3. Variables and Constants: Storing Data
Variables are named storage locations that hold data values. These values can change during program execution. In contrast, constants store values that remain fixed throughout the program's lifespan. The ability to store and manipulate data through variables and constants is a core feature of all programming languages. The method of declaring and using them may differ (e.g., int age = 30;
vs. age = 30
), but the underlying purpose is identical.
4. Operators: Performing Operations
Operators are symbols that perform operations on data. Common operators include:
- Arithmetic operators:
+
,-
,*
,/
,%
(modulo). - Relational operators:
>
,<
,>=
,<=
,==
(equals),!=
(not equals). - Logical operators:
&&
(AND),||
(OR),!
(NOT). - Assignment operators:
=
,+=
,-=
,*=
etc.
These operators, or their functional equivalents, exist in every programming language, allowing for complex calculations, comparisons, and manipulations of data.
5. Control Structures: Managing Program Flow
Control structures determine the order in which code is executed. They allow programmers to create dynamic and responsive programs. The most common control structures include:
- Sequential execution: Code is executed line by line, from top to bottom.
- Conditional statements (if-else): Execute code blocks based on certain conditions.
- Loops (for, while): Repeatedly execute a block of code until a specific condition is met.
While the syntax varies (e.g., for
loops in C++ vs. Python's for
loops), the fundamental concept of controlling program flow remains the same across all languages.
6. Functions/Procedures/Methods: Modularizing Code
Functions (or procedures/methods) are blocks of code designed to perform specific tasks. They promote code reusability, modularity, and readability. Breaking down a large program into smaller, manageable functions is a crucial aspect of software development, regardless of the language used. The concept of encapsulating a set of instructions within a reusable unit is present in all programming languages.
7. Input/Output (I/O): Interacting with the World
All programs need to interact with the external world, whether it's reading data from a file, taking user input, or displaying results on the screen. Input/Output operations provide this capability. The specific mechanisms for I/O vary, but the core functionality—taking in data and sending out results—is universal.
8. Comments: Enhancing Readability
Comments are explanatory notes within the code. They don't affect the program's execution but greatly improve readability and maintainability. While the syntax for comments differs (e.g., //
in C++ vs. #
in Python), their purpose—to document code and make it easier to understand—remains unchanged.
9. Error Handling and Exception Management: Robustness
Real-world programs encounter errors. Error handling mechanisms allow developers to gracefully manage unexpected situations, preventing crashes and ensuring program stability. Techniques like try-catch
blocks (or their equivalents) are common across many languages, providing a structured way to deal with potential errors.
10. Memory Management: Resource Allocation and Deallocation
Programs need memory to store data and execute instructions. Memory management is the process of allocating and deallocating memory during program execution. While some languages (like Python) have automatic garbage collection, others (like C++) require manual memory management. The fundamental need to manage resources efficiently remains consistent, though the approach varies based on language design.
Beyond the Fundamentals: Higher-Level Concepts Shared Across Languages
While the above are foundational, several higher-level concepts are also common across programming paradigms:
- Object-Oriented Programming (OOP): Concepts like encapsulation, inheritance, and polymorphism are present, even if their implementation differs, across various OOP languages like Java, C++, Python, and C#.
- Data Structures: Though implementation may vary, data structures like linked lists, trees, graphs, stacks, and queues are used for efficient data organization and manipulation across numerous languages.
- Algorithms and Data Structures: The core algorithms for searching, sorting, and other common operations are applicable across languages, irrespective of their syntactic differences.
- Debugging and Testing: The need for thorough debugging and testing remains consistent. While debugging tools vary, the principles of identifying and fixing errors are fundamental to all software development.
Conclusion: The Unifying Principles of Programming Languages
Despite the vast landscape of programming languages, a set of core principles and fundamental concepts unites them all. Understanding these commonalities empowers programmers to learn new languages more effectively and appreciate the underlying logic of computation. The ability to manipulate data, control program flow, modularize code, handle errors, and manage resources—these are the essential skills that transcend any specific programming language, forming the basis of all software development. The specific syntax might change, but the fundamental building blocks of programming remain strikingly consistent across the board. This shared foundation allows for a rich exchange of ideas and practices across the diverse community of programmers worldwide.
Latest Posts
Related Post
Thank you for visiting our website which covers about Which Of The Following Are Common To All Programming Languages . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.