Which Method Or Operator Can Be Used To Concatenate Lists
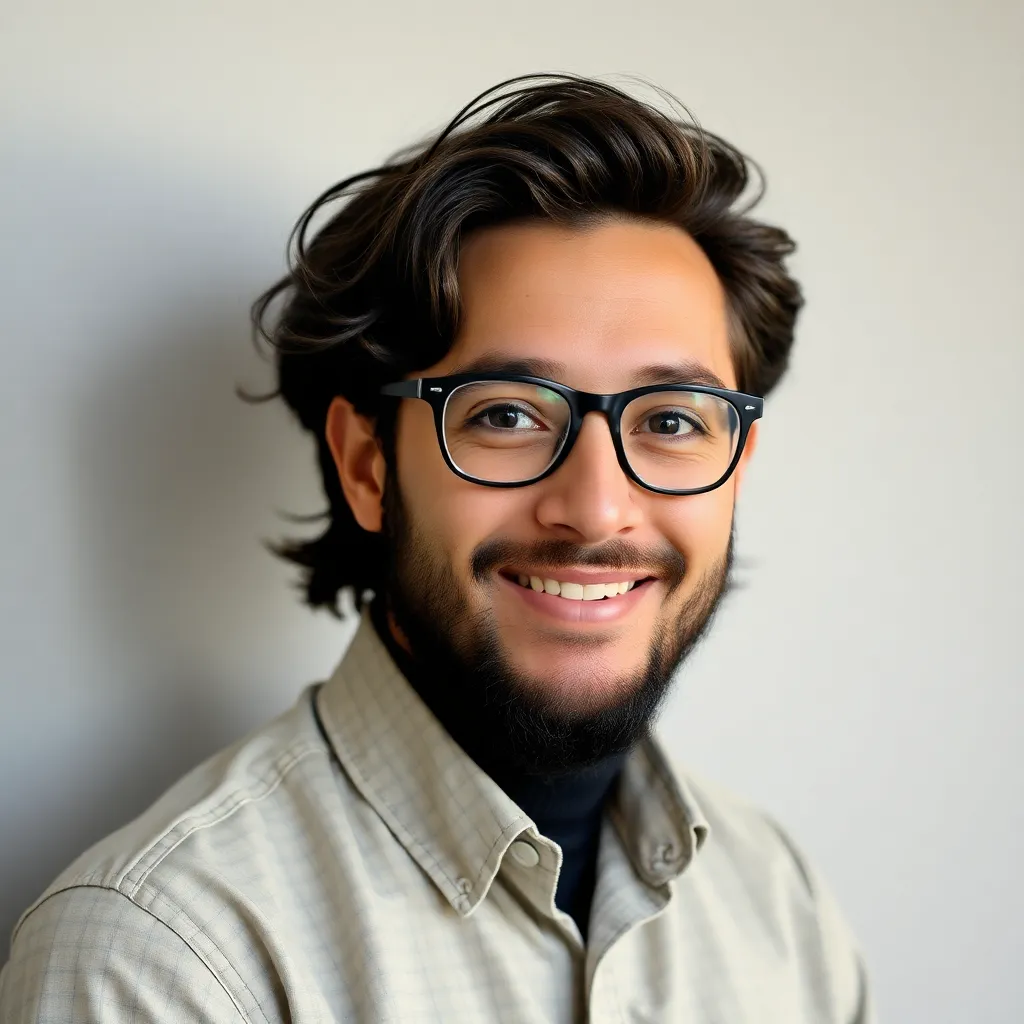
Holbox
Apr 02, 2025 · 6 min read

Table of Contents
- Which Method Or Operator Can Be Used To Concatenate Lists
- Table of Contents
- Which Method or Operator Can Be Used to Concatenate Lists? A Deep Dive into List Concatenation Techniques
- Python's Elegant Approach: The + Operator and extend() Method
- The + Operator: Simplicity and Readability
- The extend() Method: In-place Modification for Efficiency
- JavaScript's Concatenation Techniques
- The concat() Method: A Versatile Approach
- The Spread Syntax (...): Modern and Concise
- Java's List Concatenation: Using addAll()
- C#'s List Concatenation: AddRange() and the + Operator
- AddRange() Method
- The + Operator
- Choosing the Right Method: A Summary
- Latest Posts
- Latest Posts
- Related Post
Which Method or Operator Can Be Used to Concatenate Lists? A Deep Dive into List Concatenation Techniques
Concatenating lists—combining two or more lists into a single, unified list—is a fundamental operation in many programming tasks. Whether you're processing data, manipulating arrays, or building complex data structures, understanding the efficient and elegant ways to join lists is crucial. This comprehensive guide explores various methods and operators for list concatenation in several popular programming languages, highlighting their strengths, weaknesses, and best-use cases. We'll delve into the nuances of each technique, ensuring you're equipped to choose the optimal approach for your specific needs.
Python's Elegant Approach: The +
Operator and extend()
Method
Python offers two primary methods for list concatenation: the +
operator and the extend()
method. Both achieve the same outcome – joining lists – but differ significantly in their underlying mechanisms and efficiency.
The +
Operator: Simplicity and Readability
The +
operator provides a straightforward and highly readable way to concatenate lists. It creates a new list containing all the elements from the original lists.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = list1 + list2 # Concatenates list1 and list2
print(list3) # Output: [1, 2, 3, 4, 5, 6]
Advantages:
- Intuitive and easy to understand: The
+
operator's syntax is clear and immediately understandable, even for beginners. - Readability: It improves code readability, making it easier to maintain and debug.
Disadvantages:
- Inefficient for large lists: The
+
operator creates a completely new list in memory each time it's used. For very large lists, this can be computationally expensive and consume significant memory. This is because it involves copying all elements from both lists into the new list. - Not in-place: The original lists remain unchanged. A new list is created to hold the concatenated result.
The extend()
Method: In-place Modification for Efficiency
The extend()
method offers a more memory-efficient approach, particularly when dealing with large lists. Instead of creating a new list, it modifies the list in-place by adding all items from the iterable (another list, tuple, etc.) to the end of the existing list.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2) # Modifies list1 in-place
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Advantages:
- Memory efficient:
extend()
avoids the overhead of creating a new list, making it significantly faster and more memory-friendly for large lists. - In-place modification: Modifies the existing list directly, saving memory and improving performance.
Disadvantages:
- Modifies the original list: This can be a disadvantage if you need to preserve the original lists.
Choosing Between +
and extend()
:
For small lists or situations where readability is paramount, the +
operator is perfectly acceptable. However, for large lists or performance-critical applications, the extend()
method is the preferred choice due to its superior memory efficiency and speed.
JavaScript's Concatenation Techniques
JavaScript offers several ways to concatenate arrays, each with its own strengths and weaknesses.
The concat()
Method: A Versatile Approach
The concat()
method is JavaScript's primary array concatenation method. It creates a new array containing the elements from the original arrays and any additional items provided as arguments.
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const array3 = array1.concat(array2); // Creates a new array
console.log(array3); // Output: [1, 2, 3, 4, 5, 6]
Advantages:
- Versatile: Handles multiple arrays as arguments.
- Creates a new array: Leaves the original arrays unchanged.
Disadvantages:
- Inefficient for very large arrays: Similar to Python's
+
operator, it creates a new array, which can be inefficient for extremely large arrays.
The Spread Syntax (...
): Modern and Concise
The spread syntax (...
) provides a more modern and concise way to concatenate arrays in JavaScript. It's particularly useful for combining multiple arrays in a single expression.
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const array3 = [...array1, ...array2]; // Uses the spread syntax
console.log(array3); // Output: [1, 2, 3, 4, 5, 6]
Advantages:
- Concise and readable: The spread syntax leads to cleaner and more readable code.
- Efficient for multiple arrays: Handles multiple array concatenations elegantly.
Disadvantages:
- Creates a new array: Similar to
concat()
, it creates a new array, impacting efficiency for very large arrays.
Java's List Concatenation: Using addAll()
In Java, the addAll()
method is the standard way to concatenate lists. This method modifies the list it's called upon, adding all elements from the specified collection (another list) to the end.
import java.util.ArrayList;
import java.util.List;
public class ListConcatenation {
public static void main(String[] args) {
List list1 = new ArrayList<>(List.of(1, 2, 3));
List list2 = new ArrayList<>(List.of(4, 5, 6));
list1.addAll(list2); // Modifies list1 in-place
System.out.println(list1); // Output: [1, 2, 3, 4, 5, 6]
}
}
Advantages:
- In-place modification:
addAll()
modifies the list directly, improving efficiency for larger lists.
Disadvantages:
- Modifies the original list: Similar to Python's
extend()
, it alters the original list, which may not be desirable in all situations.
C#'s List Concatenation: AddRange()
and the +
Operator
C# offers similar options to other languages. You can use the AddRange()
method for in-place concatenation or the +
operator to create a new list.
AddRange()
Method
using System;
using System.Collections.Generic;
using System.Linq;
public class ListConcatenation
{
public static void Main(string[] args)
{
List list1 = new List { 1, 2, 3 };
List list2 = new List { 4, 5, 6 };
list1.AddRange(list2); // Modifies list1 in-place
Console.WriteLine(string.Join(", ", list1)); // Output: 1, 2, 3, 4, 5, 6
}
}
The +
Operator
using System;
using System.Collections.Generic;
using System.Linq;
public class ListConcatenation
{
public static void Main(string[] args)
{
List list1 = new List { 1, 2, 3 };
List list2 = new List { 4, 5, 6 };
List list3 = list1.Concat(list2).ToList(); // Creates a new list
Console.WriteLine(string.Join(", ", list3)); // Output: 1, 2, 3, 4, 5, 6
}
}
The +
operator in C# for lists isn't a direct concatenation; it uses the Concat
method internally, which creates a new sequence. ToList()
converts it back to a List.
Choosing the Right Method: A Summary
The optimal method for concatenating lists depends heavily on the specific context:
- For small lists and readability: The
+
operator (Python, C#) orconcat()
(JavaScript) often suffices. - For large lists and efficiency: In-place methods like
extend()
(Python),addAll()
(Java), orAddRange()
(C#) are strongly recommended to avoid excessive memory usage and improve performance. - For multiple lists and conciseness: JavaScript's spread syntax offers a highly readable and efficient way to combine multiple arrays.
Remember to consider the trade-off between readability and efficiency when choosing your concatenation method. For extremely large datasets, the memory footprint of creating new lists can become a significant bottleneck, making in-place methods crucial. Always profile your code to determine the best approach for your specific application. Understanding the nuances of these different approaches empowers you to write more efficient and maintainable code.
Latest Posts
Latest Posts
-
When An Object Is Located Very Far Away From
Apr 05, 2025
-
The Industrial Revolution And Modern Economic Growth Resulted In
Apr 05, 2025
-
In Constructing A Demand Curve For Product X
Apr 05, 2025
-
Today Is June 21 2019 Based On These Birth Dates
Apr 05, 2025
-
What Should You Do With Reagents Slides With Patient Samples
Apr 05, 2025
Related Post
Thank you for visiting our website which covers about Which Method Or Operator Can Be Used To Concatenate Lists . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.