What Is The Output Of The Following Program
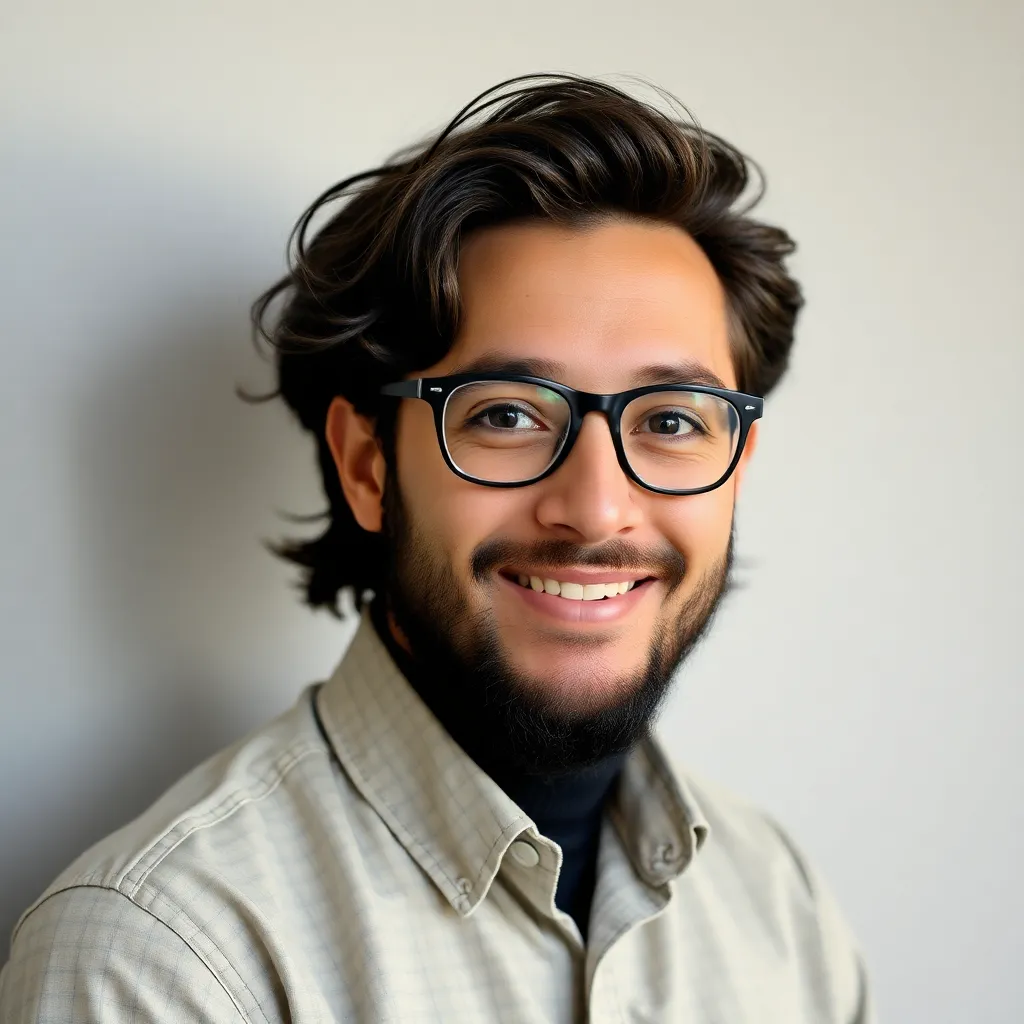
Holbox
May 12, 2025 · 5 min read
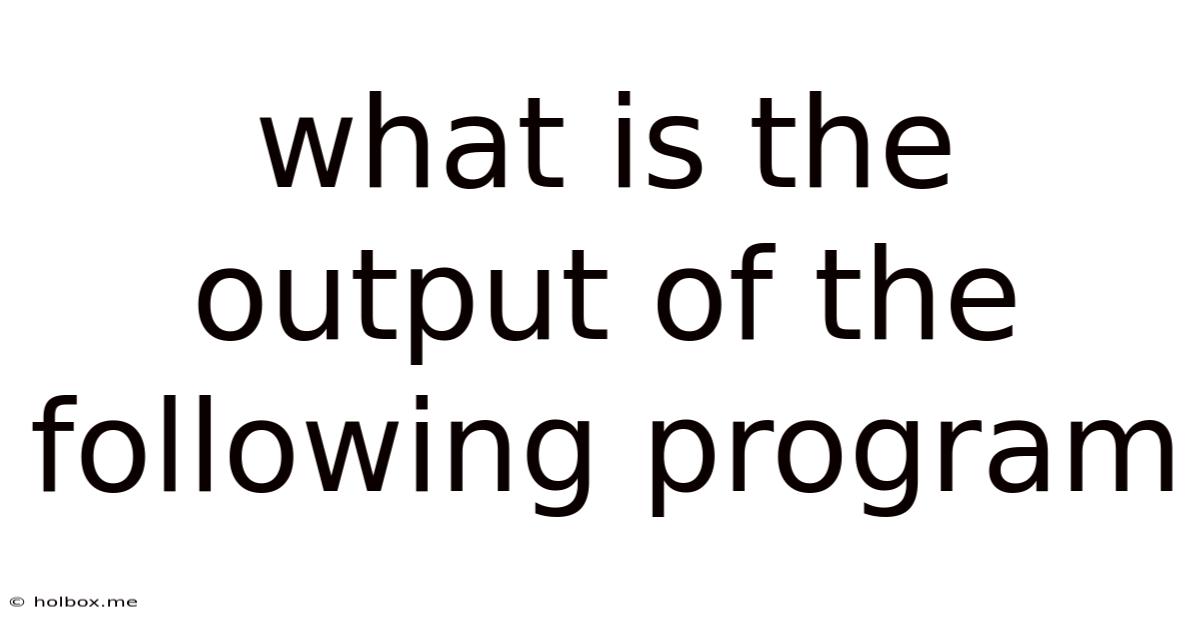
Table of Contents
- What Is The Output Of The Following Program
- Table of Contents
- Decoding Program Output: A Comprehensive Guide
- Understanding Program Structure and Execution Flow
- Example 1: A Simple Arithmetic Program
- Example 2: Conditional Statements and Output
- Example 3: Looping and Output
- Example 4: Functions and Output
- Example 5: Handling User Input and Output
- Example 6: Arrays and Output
- Example 7: Error Handling and Output
- Advanced Scenarios and Challenges
- Strategies for Accurate Output Prediction
- Conclusion
- Latest Posts
- Related Post
Decoding Program Output: A Comprehensive Guide
Predicting the output of a program requires a deep understanding of programming concepts, including data types, control flow, functions, and memory management. This article will delve into the process of analyzing code and predicting its output, using various examples to illustrate different scenarios. We'll explore common programming paradigms and potential pitfalls that can lead to unexpected results. The complexity of accurately predicting output varies greatly based on the program's size and complexity, but this guide provides a structured approach to tackle even the most challenging cases.
Understanding Program Structure and Execution Flow
Before we dive into specific examples, let's establish the fundamentals. A program's output is determined by its sequence of instructions and how these instructions interact with data. Understanding the execution flow is paramount:
- Sequential Execution: Instructions are executed one after another, line by line, unless otherwise specified.
- Conditional Statements (if-else): The execution path depends on the evaluation of conditions. Different code blocks will execute based on whether a condition is true or false.
- Loops (for, while): These statements allow for repeated execution of a block of code. The number of iterations depends on the loop's condition.
- Functions/Methods: Code is organized into reusable blocks. Functions take input, perform operations, and potentially return output.
- Data Structures: How data is organized (arrays, lists, dictionaries, etc.) significantly affects program behavior.
Example 1: A Simple Arithmetic Program
Let's start with a very basic example:
#include
int main() {
int a = 10;
int b = 5;
int sum = a + b;
std::cout << "The sum is: " << sum << std::endl;
return 0;
}
Output Prediction: This program is straightforward. It initializes two integer variables, a
and b
, adds them together, and then prints the result to the console. The output will be:
The sum is: 15
Example 2: Conditional Statements and Output
Now let's introduce a conditional statement:
x = 15
if x > 10:
print("x is greater than 10")
else:
print("x is not greater than 10")
Output Prediction: The condition x > 10
evaluates to True
, so the first print
statement will be executed. The output is:
x is greater than 10
Example 3: Looping and Output
Loops add another layer of complexity. Consider this Python example:
for i in range(1, 5):
print(i * 2)
Output Prediction: This program iterates four times (from 1 to 4). In each iteration, it prints the value of i
multiplied by 2. The output will be:
2
4
6
8
Example 4: Functions and Output
Functions encapsulate code, making programs more modular and readable. Here’s a C++ example:
#include
int square(int x) {
return x * x;
}
int main() {
int num = 7;
int result = square(num);
std::cout << "The square of " << num << " is: " << result << std::endl;
return 0;
}
Output Prediction: The square
function calculates the square of its input. The main
function calls square
with num = 7
, and the result (49) is printed. The output will be:
The square of 7 is: 49
Example 5: Handling User Input and Output
Many programs interact with the user. Let's examine a simple Python example:
name = input("Enter your name: ")
print("Hello, " + name + "!")
Output Prediction: This program prompts the user to enter their name. The program then prints a greeting using the entered name. The output will depend on the user's input. If the user enters "Alice", the output will be:
Enter your name: Alice
Hello, Alice!
Example 6: Arrays and Output
Arrays (or lists in Python) introduce complexities in output prediction, particularly when loops are involved:
public class ArrayExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.print(numbers[i] * 2 + " ");
}
}
}
Output Prediction: This Java code iterates through an integer array, multiplying each element by 2 and printing the result. The output will be:
2 4 6 8 10
Example 7: Error Handling and Output
Programs can encounter errors during execution. How errors are handled impacts the output:
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: Division by zero")
Output Prediction: This code attempts to divide 10 by 0, which will raise a ZeroDivisionError
. The except
block catches this error and prints an error message. The output will be:
Error: Division by zero
Advanced Scenarios and Challenges
Predicting the output of more complex programs requires a deeper understanding of several factors:
- Concurrency and Multithreading: In programs with multiple threads running concurrently, the output can be non-deterministic and depend on the timing of thread execution.
- External Libraries and APIs: The behavior of external code (libraries, APIs, system calls) needs to be considered.
- Memory Management: Issues like memory leaks or pointer errors can lead to unpredictable behavior and crashes, making output prediction unreliable.
- Recursion: Recursive functions can be challenging to analyze, requiring careful tracking of the call stack and recursive calls.
- Dynamically Typed Languages: In languages like Python, the type of a variable can change during runtime, affecting the program's behavior and output.
Strategies for Accurate Output Prediction
To effectively predict a program's output:
- Read the code carefully: Understand the logic and flow of the program step by step.
- Trace the execution: Manually walk through the code, keeping track of variable values and program state.
- Use debugging tools: Debuggers allow you to step through the code line by line, inspecting variables and identifying errors.
- Test with sample inputs: Run the program with various inputs to validate the output against your predictions.
- Break down complex programs: Divide large programs into smaller, more manageable parts.
Conclusion
Predicting program output is a fundamental skill for any programmer. While simple programs are relatively easy to analyze, more complex programs require a methodical approach, careful attention to detail, and a solid understanding of programming concepts. By combining careful code reading, manual tracing, and potentially debugging tools, you can significantly improve your accuracy in predicting and understanding program outputs. Remember to always test your predictions with real executions to validate your analysis and identify any unexpected behavior.
Latest Posts
Related Post
Thank you for visiting our website which covers about What Is The Output Of The Following Program . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.