What Is The First Negative Index In A List
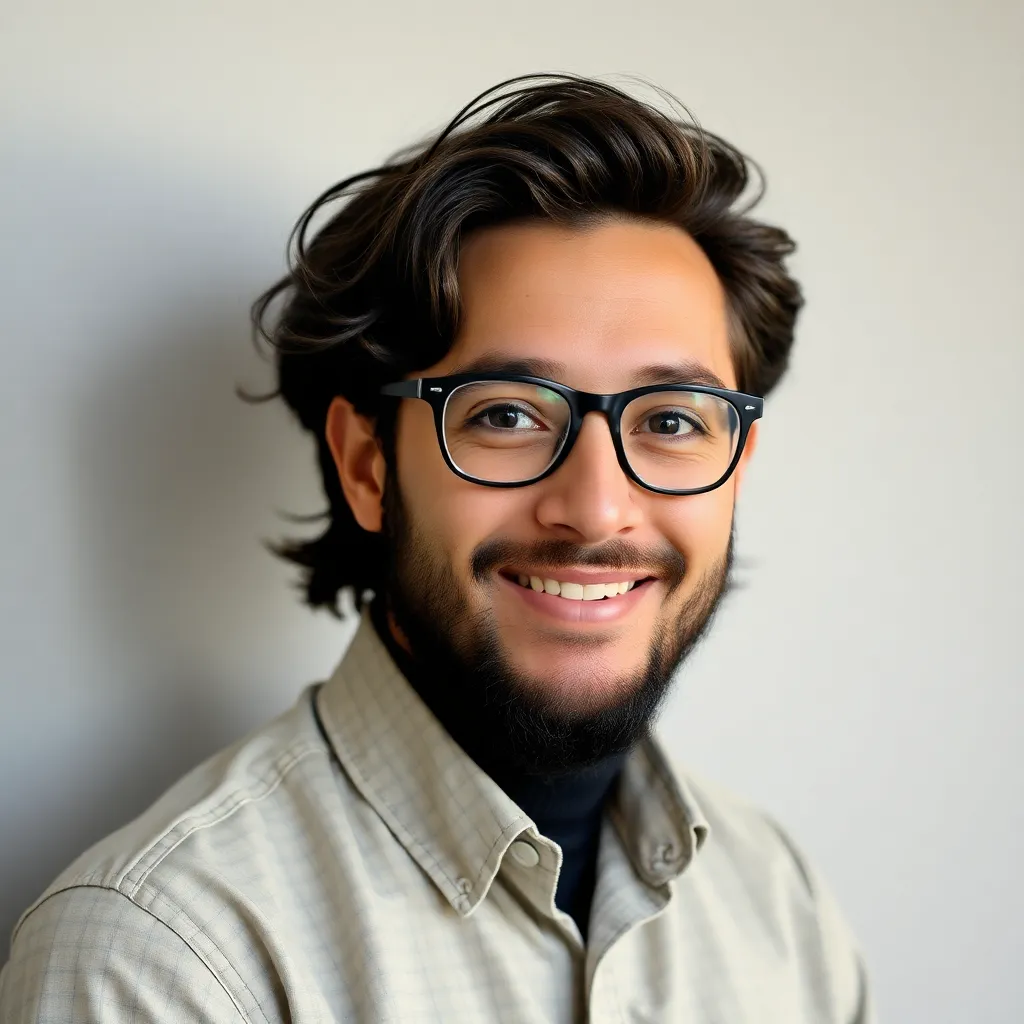
Holbox
May 11, 2025 · 5 min read
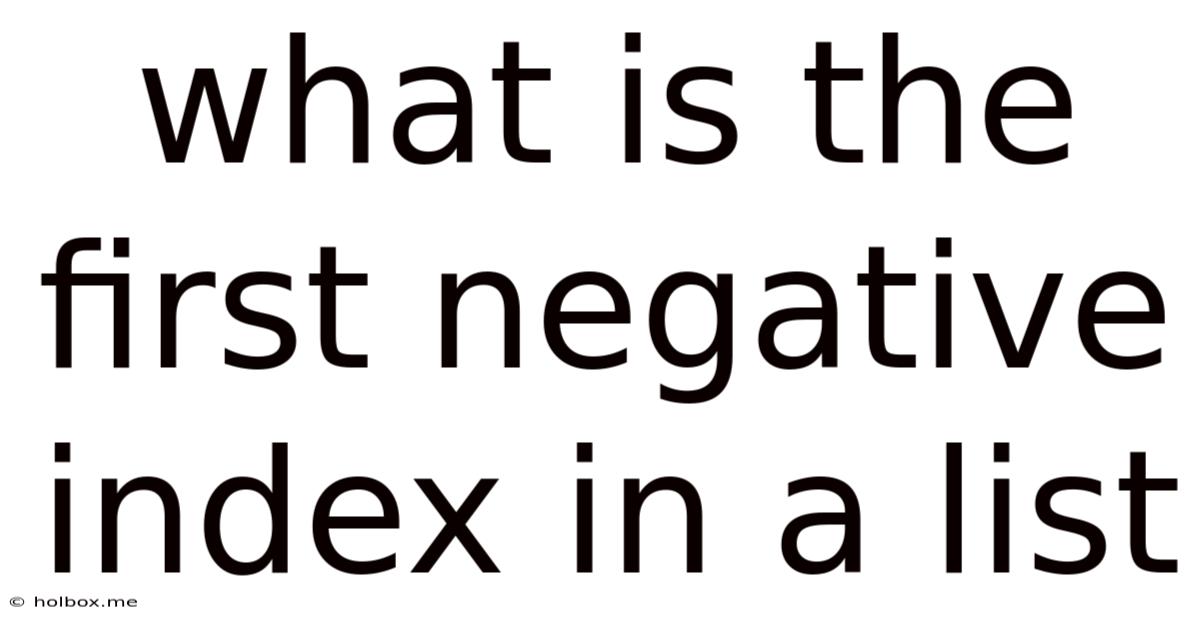
Table of Contents
- What Is The First Negative Index In A List
- Table of Contents
- What is the First Negative Index in a List? A Deep Dive into Indexing and Data Structures
- Understanding Positive and Negative Indexing
- Identifying the "First" Negative Index
- Why -1 and not -0?
- Practical Applications of Negative Indexing
- Negative Indexing Across Programming Languages
- Advanced Concepts and Considerations
- Illustrative Examples
- Conclusion
- Latest Posts
- Related Post
What is the First Negative Index in a List? A Deep Dive into Indexing and Data Structures
Understanding indexing in lists and other data structures is fundamental to programming. While positive indexing, starting from 0, is widely understood, negative indexing often introduces confusion. This article will thoroughly explore the concept of negative indexing, specifically focusing on what constitutes the "first" negative index in a list, and its implications across various programming languages and contexts. We'll delve into practical examples, clarifying misconceptions and solidifying your understanding of this powerful tool.
Understanding Positive and Negative Indexing
Before diving into negative indexing, let's solidify our grasp on positive indexing. In most programming languages, list elements (or array elements) are accessed using an index, a numerical representation of the element's position within the list. Positive indexing begins at 0; the first element is at index 0, the second at index 1, and so on.
my_list = ["apple", "banana", "cherry", "date"]
print(my_list[0]) # Output: apple
print(my_list[1]) # Output: banana
print(my_list[3]) # Output: date
Negative indexing, conversely, starts from the end of the list. The last element is at index -1, the second to last at index -2, and so forth. This provides a convenient way to access elements from the end without needing to know the list's length.
my_list = ["apple", "banana", "cherry", "date"]
print(my_list[-1]) # Output: date
print(my_list[-2]) # Output: cherry
print(my_list[-4]) # Output: apple
Identifying the "First" Negative Index
The "first" negative index is unequivocally -1. It always refers to the last element in the list, regardless of the list's size or content. This consistency is a key strength of negative indexing; it allows for concise and reliable code, even when dealing with dynamically sized lists.
Why -1 and not -0?
You might wonder why the first negative index isn't -0. The reason is rooted in the underlying implementation of lists and how indices are translated into memory addresses. A -0 index would be numerically equivalent to 0, creating ambiguity and potentially leading to unexpected behavior. Using -1 as the starting point for negative indexing avoids this conflict, providing a clear and unambiguous system.
Practical Applications of Negative Indexing
Negative indexing is far more than a mere syntactic curiosity. It significantly improves code readability and efficiency in various scenarios:
-
Retrieving the last element: Accessing the last element of a list becomes trivial:
my_list[-1]
. This is especially useful when processing data streams where the latest entry is of primary importance. -
Slicing from the end: Negative indices are indispensable when slicing lists from the end. For example,
my_list[-3:]
extracts the last three elements. This is often used for tasks like examining recent activity logs or processing the tail end of a data stream. -
Efficient manipulation of the end of a list: Tasks like adding or removing elements from the end of a list are naturally expressed using negative indexing. Appending an element is inherently linked to the last position (-1).
-
Enhanced readability and conciseness: Using negative indices often leads to more compact and readable code, as demonstrated in the examples above. It avoids the need to calculate the length of the list and reduces the chances of off-by-one errors.
Negative Indexing Across Programming Languages
While the concept of negative indexing is common, its specific implementation and behavior might vary slightly across programming languages. However, the fundamental principle of starting from -1 to access elements from the end remains consistent.
Python: Python boasts robust support for negative indexing, as seen in the earlier examples. It seamlessly integrates with list slicing and other list manipulation functions.
Java: Java's arrays use positive indexing only. To access the last element, you would need to use my_array[my_array.length - 1]
. While Java doesn't directly support negative indexing for arrays, similar functionalities can be achieved using methods and libraries.
JavaScript: JavaScript arrays support negative indexing, mirroring Python's behavior. Accessing the last element is straightforward using my_array[-1]
.
C++: C++'s standard library for vectors and arrays employs positive indexing. Accessing elements from the end requires explicit calculation like in Java. However, libraries like Boost provide functionalities that might simplify accessing elements from the end in a manner similar to negative indexing.
Advanced Concepts and Considerations
-
Empty Lists: Attempting to access an element using a negative index in an empty list will generally result in an
IndexError
(or similar exception) in most languages. -
Multi-dimensional Arrays: Negative indexing can extend to multi-dimensional arrays, allowing access to elements from the end of each dimension. The interpretation becomes slightly more complex, requiring careful consideration of the indices for each dimension.
-
Error Handling: Always handle potential
IndexError
exceptions that might arise when using negative indices, especially when dealing with user input or dynamic data.
Illustrative Examples
Let's solidify our understanding with some more comprehensive examples:
Example 1: Reversing a list using negative indexing:
my_list = [10, 20, 30, 40, 50]
reversed_list = my_list[::-1] # Elegant reversal using slicing with negative step
print(reversed_list) # Output: [50, 40, 30, 20, 10]
Example 2: Extracting a sublist from the end:
my_list = ["a", "b", "c", "d", "e", "f"]
sublist = my_list[-3:] # Extract the last 3 elements
print(sublist) # Output: ['d', 'e', 'f']
Example 3: Processing a log file (simulated):
log_entries = ["Entry 1", "Entry 2", "Entry 3", "Error: System Failure"]
if log_entries[-1].startswith("Error"):
print("System failure detected in the latest log entry!")
These examples highlight the practical utility and elegance of negative indexing in various programming scenarios.
Conclusion
The first negative index in a list is undeniably -1. This seemingly simple concept is a powerful tool in a programmer's arsenal, enhancing code readability, efficiency, and maintainability. Understanding negative indexing is crucial for effectively working with lists and other data structures. Its consistent behavior across languages, albeit with variations in implementation details, makes it an indispensable part of modern programming practices. By mastering negative indexing, you equip yourself with a more efficient and expressive approach to data manipulation. Remember to handle potential errors and consider the specific nuances of your chosen programming language, but the fundamental principle remains constant: -1 always points to the last element, providing a concise and powerful way to access and manipulate data.
Latest Posts
Related Post
Thank you for visiting our website which covers about What Is The First Negative Index In A List . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.