What Are The Data Items In A List Called
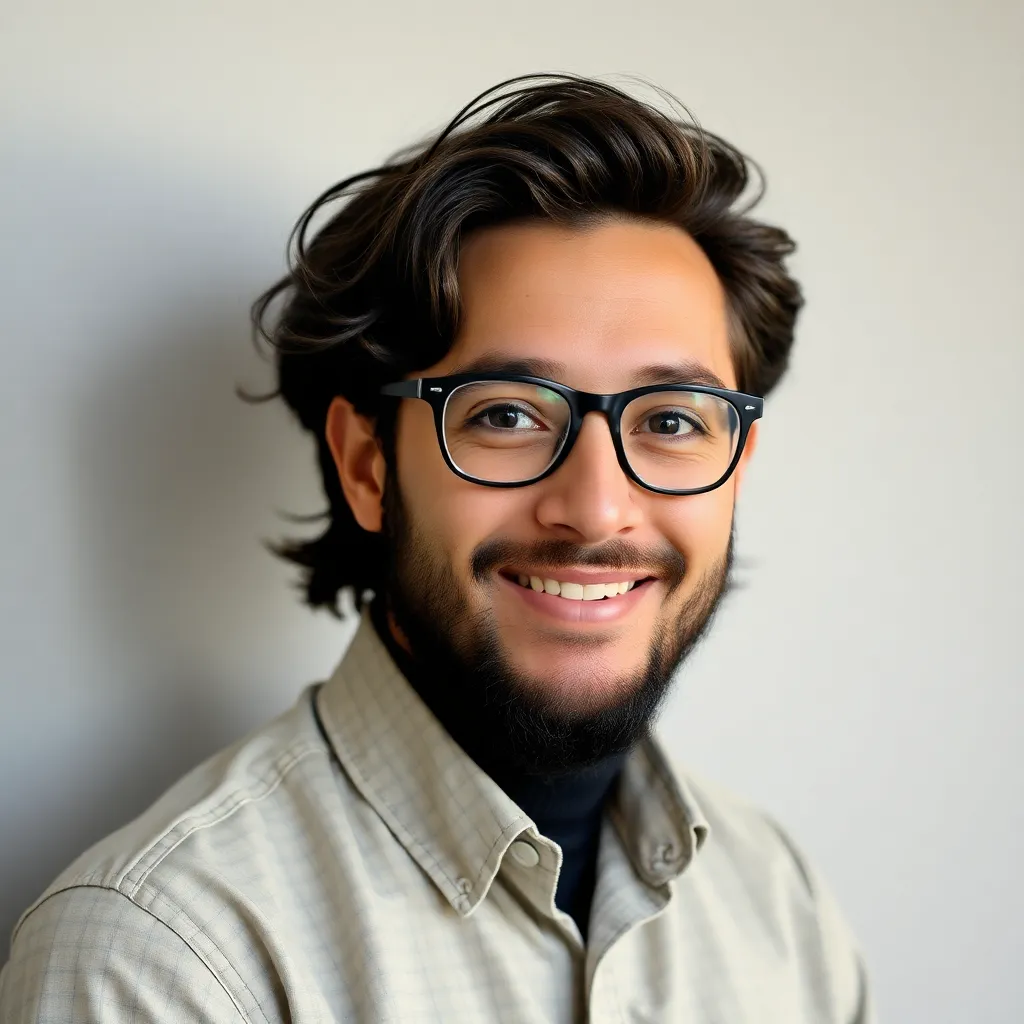
Holbox
May 02, 2025 · 5 min read
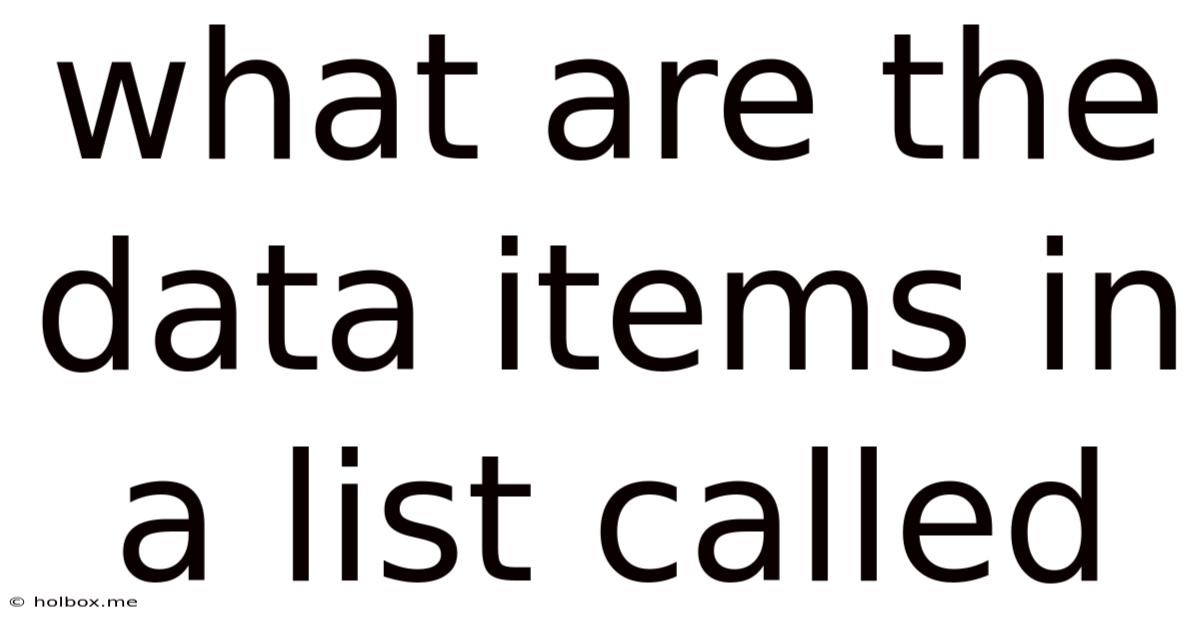
Table of Contents
- What Are The Data Items In A List Called
- Table of Contents
- What are the Data Items in a List Called? Understanding Elements, Members, and More
- Common Names for Data Items in a List
- Understanding the Context: Programming Languages and Data Structures
- Python Lists
- C++ Vectors and Arrays
- Java ArrayLists
- Beyond Basic Lists: More Complex Data Structures
- Sets
- Dictionaries (or Maps)
- Trees
- Accessing Data Items: Indexing and Iteration
- Indexing
- Iteration
- Practical Examples: Working with Lists and Their Elements
- Example 1: Finding the Largest Element
- Example 2: Summing the Elements
- Conclusion: Choosing the Right Terminology
- Latest Posts
- Related Post
What are the Data Items in a List Called? Understanding Elements, Members, and More
Data structures are fundamental building blocks in programming. Lists, a ubiquitous data structure, allow us to store collections of items. But what exactly are those individual items called? The terminology can vary slightly depending on the programming language and context, but we'll explore the common names and their nuances.
Common Names for Data Items in a List
While there isn't one universally accepted term, several names frequently describe the individual data items within a list:
-
Elements: This is arguably the most common and widely understood term. It's a general term that applies across many programming languages and contexts. Think of each item as a fundamental element contributing to the whole list.
-
Members: Similar to "elements," "members" denotes the individual components of the list. This term is often used in the context of object-oriented programming, where lists might be considered collections of member objects.
-
Items: A simple and straightforward term. "Items" clearly conveys the idea of individual entities within the list, without getting overly technical.
-
Nodes (in Linked Lists): In the specific case of linked lists (a type of list where each element points to the next), the individual data items are often referred to as nodes. Each node contains both the data and a pointer to the next node in the sequence.
-
Values: This term emphasizes the data held by each element. Each element holds a value, which could be a number, string, object, or any other data type.
Understanding the Context: Programming Languages and Data Structures
The preferred term can subtly shift depending on the programming language and the specific type of list being used.
Python Lists
In Python, the terms elements, items, and values are all frequently used interchangeably to refer to the individual items in a list. Python's flexible and dynamic nature allows for lists to contain diverse data types, further supporting the use of these general terms.
my_list = [10, "hello", 3.14, True] # Elements/Items/Values in a Python list
print(my_list[0]) # Accessing the first element (10)
C++ Vectors and Arrays
In C++, vectors (dynamic arrays) and arrays often use elements as the primary term. The term is precise and clearly distinguishes the individual components from the overall vector or array structure.
std::vector myVector = {1, 2, 3, 4, 5}; // Elements in a C++ vector
std::cout << myVector[2] << std::endl; // Accessing the third element (3)
Java ArrayLists
Java's ArrayList
typically uses elements as the standard terminology. The structure's design and its common usage reinforce the consistent application of this term.
ArrayList myList = new ArrayList<>();
myList.add("apple");
myList.add("banana");
myList.add("cherry"); // Elements in a Java ArrayList
System.out.println(myList.get(1)); // Accessing the second element ("banana")
Beyond Basic Lists: More Complex Data Structures
The naming conventions extend beyond simple lists to more intricate data structures.
Sets
In sets (unordered collections of unique elements), the individual items are often referred to as elements or members. The focus is on the presence or absence of a particular element within the set.
Dictionaries (or Maps)
Dictionaries, also known as maps, consist of key-value pairs. While the entire pair is a single entry or item, the individual components are often called keys and values. The key uniquely identifies the associated value.
Trees
In tree-like structures (such as binary trees or trees), the individual data items are typically called nodes. Each node typically holds data and pointers to its children nodes.
Accessing Data Items: Indexing and Iteration
Regardless of what you call them, accessing individual items within a list is a core aspect of working with list-based data structures. Most programming languages provide mechanisms for accessing elements using their index (position) or by iterating through the list.
Indexing
Indexing provides direct access to an element at a specific position. In most languages, indexing starts at 0 (the first element is at index 0, the second at index 1, and so on).
Iteration
Iteration allows you to process each element in the list sequentially. Looping constructs (like for
loops) are used to traverse the entire list, performing an action on each item.
Practical Examples: Working with Lists and Their Elements
Let's explore practical examples showcasing how to work with list elements in different programming languages. These examples emphasize the commonality of operations, despite potential variations in terminology.
Example 1: Finding the Largest Element
This code snippet demonstrates how to find the largest element in a list, regardless of whether you call the elements "items," "values," or "elements." The core logic remains consistent.
my_list = [15, 2, 8, 42, 7]
largest_element = my_list[0] # Initialize with the first element
for element in my_list: # Iterate through each element
if element > largest_element:
largest_element = element
print("The largest element is:", largest_element) # Output: The largest element is: 42
Example 2: Summing the Elements
This example shows how to calculate the sum of all elements in a list. The method remains consistent even if the elements have different names.
ArrayList numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
numbers.add(30);
numbers.add(40);
int sum = 0;
for (int number : numbers) { // Iterate through each element (number)
sum += number;
}
System.out.println("The sum is: " + sum); // Output: The sum is: 100
Conclusion: Choosing the Right Terminology
While the precise terminology used to refer to individual data items in a list might vary slightly across programming languages and data structures, the underlying concepts remain consistent. Understanding the common names—elements, members, items, nodes, values—and their subtle nuances will significantly improve your understanding and ability to work effectively with list-based data structures. Choosing the appropriate term often depends on context and personal preference, but prioritizing clarity and consistency within your codebase is crucial for maintainability and readability. Focus on understanding the functionality of accessing and manipulating these elements, rather than getting bogged down in minor terminological variations.
Latest Posts
Related Post
Thank you for visiting our website which covers about What Are The Data Items In A List Called . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.