Type An Integer Or A Decimal
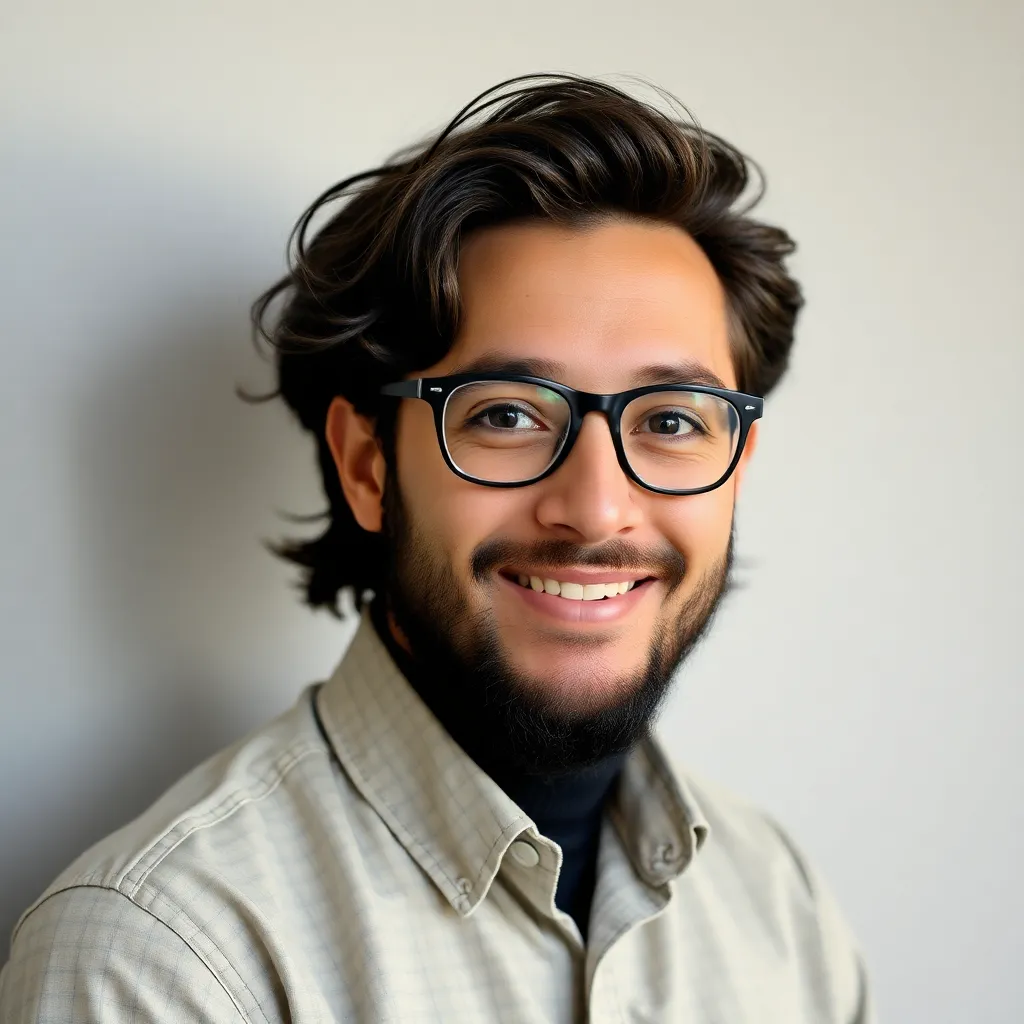
Holbox
May 12, 2025 · 6 min read
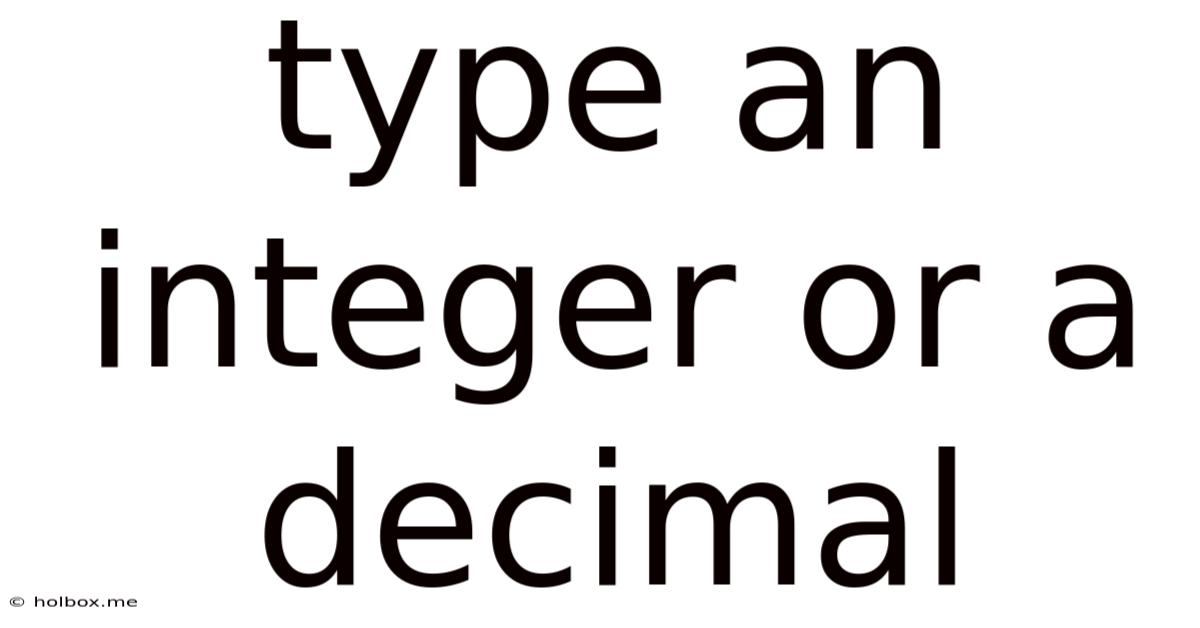
Table of Contents
- Type An Integer Or A Decimal
- Table of Contents
- Type an Integer or a Decimal: A Deep Dive into Number Representation and Data Types
- Integers: The Whole Story
- Representing Integers in Memory:
- Common Integer Data Types:
- Integer Arithmetic:
- Decimals: Embracing Fractions
- Representing Decimals in Memory:
- Common Decimal Data Types:
- Decimal Arithmetic:
- Choosing the Right Data Type: Precision vs. Range vs. Memory
- Potential Pitfalls and Best Practices
- Specific Language Examples
- Python:
- Java:
- C++:
- Conclusion
- Latest Posts
- Related Post
Type an Integer or a Decimal: A Deep Dive into Number Representation and Data Types
Understanding how computers represent numbers is fundamental to programming. This article delves into the crucial difference between integers and decimals, exploring their representation, use cases, and potential pitfalls in various programming languages. We'll also examine how choosing the right data type significantly impacts the accuracy and efficiency of your code.
Integers: The Whole Story
Integers are whole numbers, both positive and negative, including zero. They lack a fractional component. In programming, integers are typically represented using a fixed number of bits, leading to limitations on the range of values that can be stored. The size of the integer data type (e.g., 8-bit, 16-bit, 32-bit, 64-bit) directly determines its range.
Representing Integers in Memory:
Computers use binary (base-2) to represent integers. Each bit can hold a 0 or a 1. For example, a 4-bit integer can represent numbers from 0 to 15 (2<sup>4</sup> - 1). Negative numbers are often handled using techniques like two's complement, which allows for efficient arithmetic operations.
Common Integer Data Types:
Most programming languages offer several integer data types, each with a different size and range:
int
(orinteger
): A standard integer type, often 32-bit or 64-bit depending on the system architecture and the programming language.short
(orshort int
): Usually a smaller integer type, typically 16-bit.long
(orlong int
): Typically a larger integer type, often 64-bit or even larger, offering a wider range than a standardint
.long long
: An even larger integer type, providing an exceptionally wide range, suitable for handling extremely large numbers.unsigned int
(and similar variations): These data types only represent non-negative integers, effectively doubling the positive range compared to their signed counterparts.
Integer Arithmetic:
Arithmetic operations on integers are generally straightforward. However, it's crucial to be aware of potential overflow and underflow issues. If the result of an arithmetic operation exceeds the maximum value or falls below the minimum value representable by the integer data type, it can lead to unexpected and potentially erroneous results.
Example (Illustrative, language-agnostic):
Let's assume a 4-bit signed integer (range: -8 to 7). If you add 7 + 5, the expected result is 12, but this exceeds the maximum value. The actual result will depend on the specific implementation (often it will wrap around to a negative value).
Decimals: Embracing Fractions
Decimals, also known as floating-point numbers, represent numbers with fractional parts. They're essential for handling real-world values that are not whole numbers, like temperatures, weights, or monetary amounts.
Representing Decimals in Memory:
Decimals are typically stored using the IEEE 754 standard, which defines formats like single-precision (float) and double-precision (double). These formats use a combination of a sign bit, an exponent, and a mantissa (fractional part) to represent a wide range of values, including very large and very small numbers.
Common Decimal Data Types:
float
(single-precision): Uses 32 bits. Offers a balance between precision and range but is susceptible to rounding errors.double
(double-precision): Uses 64 bits. Provides greater precision and a wider range thanfloat
, making it the preferred choice for many applications requiring higher accuracy.long double
: Offers even higher precision and range thandouble
but might not be uniformly supported across all platforms.
Decimal Arithmetic:
While decimal arithmetic is generally more flexible than integer arithmetic, it's crucial to be aware of the limitations of floating-point representation. Not all decimal numbers can be represented exactly in binary format. This can lead to rounding errors, which can accumulate over multiple calculations.
Example (Illustrative):
Consider the simple calculation 0.1 + 0.2. In many programming languages, the result might not be exactly 0.3 due to floating-point limitations. The difference might be extremely small, but it can become significant in complex computations.
Choosing the Right Data Type: Precision vs. Range vs. Memory
The choice between integers and decimals depends on the nature of your data and the requirements of your application.
Consider these factors:
- Accuracy: If you need precise representations of numbers with fractional parts, decimals are essential. If whole numbers suffice, integers offer efficiency and simplicity.
- Range: Integers have limited ranges; decimals offer a much wider range to accommodate extremely large or small numbers.
- Memory Usage: Integers typically require less memory than decimals. Choosing appropriate data types can help optimize your application's memory footprint.
- Computational Speed: Integer arithmetic is generally faster than decimal arithmetic. If performance is critical, using integers when possible can improve speed.
Potential Pitfalls and Best Practices
- Overflow/Underflow: Be mindful of the limits of integer data types. Use larger integer types or consider using specialized libraries designed to handle arbitrarily large integers if necessary.
- Rounding Errors: In decimal arithmetic, be aware of rounding errors and their potential accumulation. Use appropriate rounding techniques or libraries designed for increased precision if needed.
- Type Conversion: When converting between integers and decimals, be aware of potential loss of precision. Always check for any unintended side effects.
- Data Validation: Always validate user input or data from external sources to ensure it's compatible with the chosen data type to prevent errors.
- Library Support: If you need advanced features, such as handling arbitrary-precision decimals, explore available libraries for your programming language.
Specific Language Examples
While the general principles are consistent across languages, the specific data types and their behavior can vary. Let's consider a few examples:
Python:
Python offers flexible and dynamic typing, and it automatically handles integer and floating-point arithmetic. However, be mindful of the inherent limitations of floating-point representations.
integer_var = 10
decimal_var = 3.14159
result = integer_var + decimal_var # Python handles this automatically
print(result) # Output: 13.14159
#Arbitrarily large integers are supported
large_integer = 123456789012345678901234567890
print(large_integer)
Java:
Java has explicit data types. You need to declare whether a variable is an integer (int
, long
, etc.) or a decimal (float
, double
).
int integerVar = 10;
double decimalVar = 3.14159;
double result = integerVar + decimalVar;
System.out.println(result); // Output: 13.14159
//For very high precision, use BigDecimal
import java.math.BigDecimal;
BigDecimal preciseDecimal = new BigDecimal("0.1").add(new BigDecimal("0.2"));
System.out.println(preciseDecimal); //Output: 0.3
C++:
Similar to Java, C++ requires explicit type declarations.
#include
#include //For setting precision
int main() {
int integerVar = 10;
double decimalVar = 3.14159;
double result = integerVar + decimalVar;
std::cout << std::fixed << std::setprecision(10) << result << std::endl; //Output: 13.1415900000
return 0;
}
Conclusion
Understanding the nuances of integer and decimal representation is vital for writing robust and efficient programs. Carefully choosing the right data type, being aware of potential pitfalls, and employing appropriate error-handling techniques are crucial for producing reliable and accurate results. Remember to consider the specific requirements of your project to ensure you utilize the most appropriate data type and optimize your code for both precision and performance. The choices made at this fundamental level significantly impact the overall quality and success of your software.
Latest Posts
Related Post
Thank you for visiting our website which covers about Type An Integer Or A Decimal . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.