The Array Perimeter Should Not Be Empty
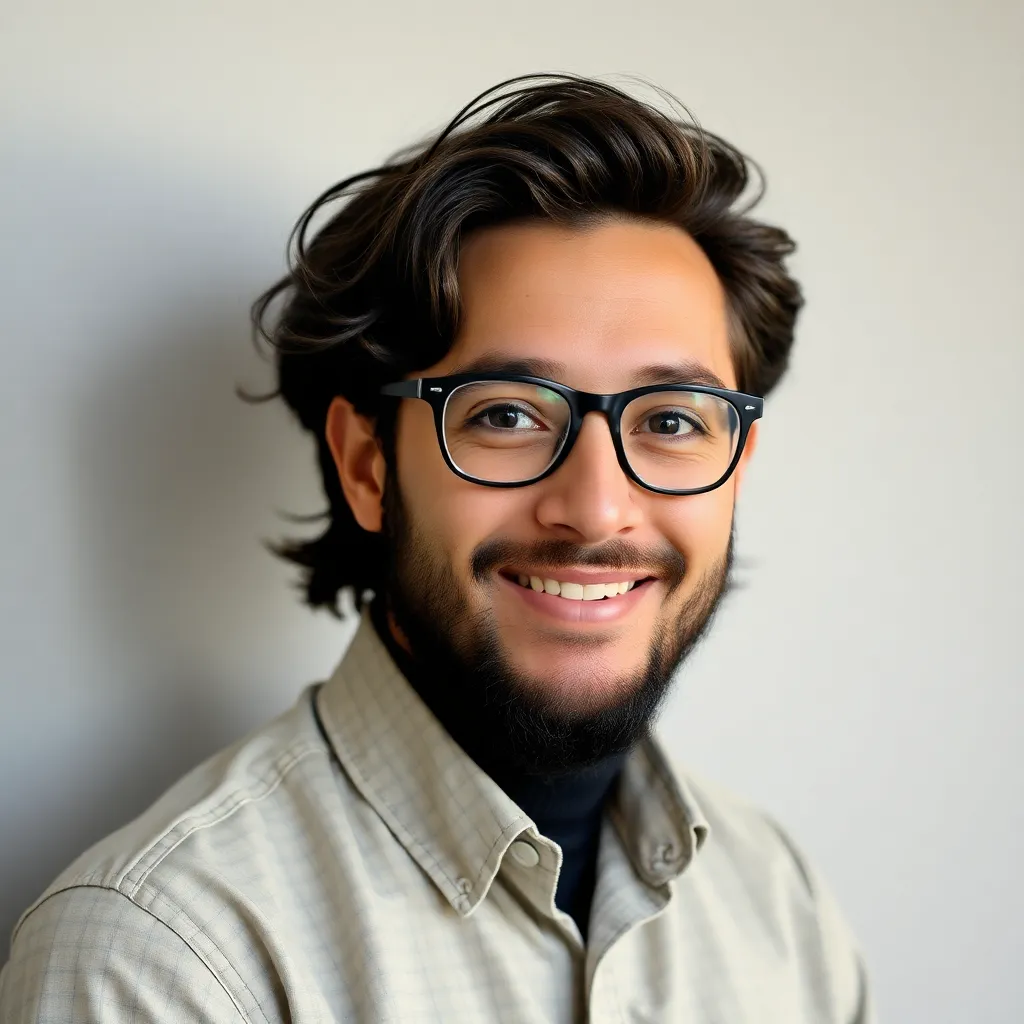
Holbox
Apr 05, 2025 · 6 min read

Table of Contents
- The Array Perimeter Should Not Be Empty
- Table of Contents
- The Array Perimeter Should Not Be Empty: A Comprehensive Guide to Handling Empty Arrays and Preventing Errors
- Understanding the "Array Perimeter Should Not Be Empty" Error
- 1. Attempting to Access Elements of an Empty Array:
- 2. Iterating Over an Empty Array Without a Check:
- 3. Using Array Functions on Empty Arrays that Require Elements:
- 4. Incorrect Data Handling Leading to Empty Arrays:
- Strategies for Preventing and Handling Empty Arrays
- 1. Input Validation:
- 2. Conditional Logic and Default Values:
- 3. Exception Handling (Try-Catch Blocks):
- 4. Defensive Programming Techniques:
- 5. Choosing Appropriate Data Structures:
- Advanced Techniques and Best Practices
- 1. Custom Exceptions:
- 2. Functional Programming Approaches:
- 3. Testing and Validation:
- Case Studies: Real-World Examples of Empty Array Issues
- Conclusion: Robust Code Requires Empty Array Awareness
- Latest Posts
- Latest Posts
- Related Post
The Array Perimeter Should Not Be Empty: A Comprehensive Guide to Handling Empty Arrays and Preventing Errors
Empty arrays, while seemingly innocuous, can be a significant source of frustration and errors in programming. The ubiquitous "array perimeter should not be empty" error, or variations thereof, signals a crucial flaw in your code's logic: you're attempting to perform an operation on an array that doesn't exist or contains no elements. This article delves deep into understanding this issue, exploring its various manifestations, and providing robust strategies to prevent and handle such situations effectively.
Understanding the "Array Perimeter Should Not Be Empty" Error
The error message itself can be misleading. It doesn't always explicitly refer to the perimeter in a geometric sense. Instead, it generally indicates that your code is trying to access or manipulate elements of an array where no elements exist. This can occur in numerous scenarios, depending on the programming language and the specific operation you're performing. Here are some common culprits:
1. Attempting to Access Elements of an Empty Array:
This is the most straightforward cause. Imagine you're trying to access the first element of an array using myArray[0]
but myArray
is empty. This will result in an error, often an IndexOutOfBoundsException
(Java), IndexError
(Python), or a similar exception depending on your language.
Example (Python):
my_array = []
first_element = my_array[0] # This will raise an IndexError
2. Iterating Over an Empty Array Without a Check:
Iterating with loops (like for
loops) over an empty array won't cause an immediate crash but can lead to unexpected behavior or errors down the line if your code relies on the loop processing at least one element.
Example (JavaScript):
let myArray = [];
let sum = 0;
for (let i = 0; i < myArray.length; i++) {
sum += myArray[i]; // This loop will execute zero times, but no error is thrown immediately. Problems arise if 'sum' is used later expecting a value.
}
3. Using Array Functions on Empty Arrays that Require Elements:
Many array functions (like pop
, shift
, slice
in JavaScript, or similar functions in other languages) expect at least one element to operate on. Attempting to use them on an empty array can result in errors or unpredictable outputs.
Example (JavaScript):
let myArray = [];
let firstElement = myArray.shift(); // This will return undefined, not an error, but might lead to issues later.
4. Incorrect Data Handling Leading to Empty Arrays:
Sometimes, the empty array is a symptom of a larger problem. Incorrect data parsing, flawed database queries, or network issues might result in your array being empty when it should contain data.
Strategies for Preventing and Handling Empty Arrays
The best approach is a multi-pronged strategy combining preventative measures and graceful error handling.
1. Input Validation:
Before working with an array, always validate its contents. Check its length (myArray.length
in many languages) before attempting any operations that rely on the array having elements.
Example (Java):
int[] myArray = {/*...some array...*/};
if (myArray.length == 0) {
System.out.println("The array is empty. Cannot proceed.");
//Handle the empty array condition appropriately - return a default value, throw a custom exception etc.
} else {
// Process the array
}
2. Conditional Logic and Default Values:
Employ if
statements to handle cases where the array is empty. Provide a default value or behavior to avoid errors. This might involve using a placeholder value, returning a default object, or simply skipping the operation.
Example (Python):
my_array = []
if my_array: #Checks if the array is not empty
# Process my_array
average = sum(my_array) / len(my_array)
else:
average = 0 #Default value if the array is empty
print(f"The average is: {average}")
3. Exception Handling (Try-Catch Blocks):
For languages that support exception handling (Java, Python, C++, etc.), wrap potentially problematic code within try-catch
blocks. This allows you to gracefully handle exceptions, such as IndexOutOfBoundsException
, preventing your program from crashing.
Example (Java):
try {
int firstElement = myArray[0]; // Potential IndexOutOfBoundsException
// Process firstElement
} catch (IndexOutOfBoundsException e) {
System.err.println("Array is empty! " + e.getMessage());
// Handle the empty array condition
}
4. Defensive Programming Techniques:
-
Null Checks: If your array could potentially be
null
(especially when dealing with objects or data from external sources), add explicit null checks before checking the length. -
Assertions: Assertions (like
assert myArray.length > 0
in Python) can be used during development to identify conditions that shouldn't occur. Assertions help catch bugs early. -
Logging: Use logging statements to record when an empty array is encountered. This can help in debugging and understanding the flow of data in your application.
5. Choosing Appropriate Data Structures:
Consider whether an array is the most suitable data structure for your needs. If the potential for empty collections is high, alternative structures like linked lists or sets might be more appropriate, depending on your specific use case.
Advanced Techniques and Best Practices
1. Custom Exceptions:
Define custom exception classes to represent specific scenarios related to empty arrays. This enhances code readability and makes error handling more informative.
Example (Java):
class EmptyArrayException extends Exception {
public EmptyArrayException(String message) {
super(message);
}
}
// ... later in your code ...
if (myArray.length == 0) {
throw new EmptyArrayException("The array cannot be empty for this operation.");
}
2. Functional Programming Approaches:
Functional programming paradigms, emphasizing immutability, can simplify handling empty arrays. Functions can be designed to gracefully handle empty input, avoiding the need for explicit checks in many cases. Techniques like map, filter, and reduce in languages like Python, JavaScript, or Scala can be used safely even on empty collections; they simply return empty results.
3. Testing and Validation:
Thorough testing is crucial. Write unit tests that explicitly test your code's behavior with empty arrays to ensure robustness.
Case Studies: Real-World Examples of Empty Array Issues
Let's examine how empty array issues can manifest in real-world scenarios:
Scenario 1: Data Processing Pipeline: Imagine a data processing pipeline where data is fetched from a database, transformed, and stored. If the database query returns no results, the array holding the data will be empty. The subsequent transformation steps must handle this gracefully to avoid errors.
Scenario 2: Web Application: In a web application, user input might be processed as an array. If a user submits a form with no data related to a specific field, the corresponding array will be empty. The backend needs to check for this emptiness before processing to avoid issues.
Scenario 3: Game Development: In a game, an array might represent the list of enemies on the screen. An empty array would imply there are no enemies present. The game logic needs to handle this case appropriately, perhaps by displaying a message, starting a new level, or performing other actions.
Conclusion: Robust Code Requires Empty Array Awareness
The "array perimeter should not be empty" error is a common yet preventable issue. By implementing the techniques outlined in this comprehensive guide, including input validation, conditional logic, exception handling, and defensive programming, developers can create more robust and resilient code that gracefully handles the possibility of empty arrays, thus ensuring smooth application execution and a better user experience. Remember that proactive prevention, coupled with well-structured error handling, is the key to writing high-quality, reliable software.
Latest Posts
Latest Posts
-
Draw A Human Epithelial Cell And An Elodea Cell
Apr 09, 2025
-
Specialized Electronic Batteries Such As Those For Laptops
Apr 09, 2025
-
Competitive Advantage Can Be Defined As
Apr 09, 2025
-
Place The Following Events Of Transcription In The Correct Order
Apr 09, 2025
-
Sophisticated Modeling Software Is Helping International Researchers
Apr 09, 2025
Related Post
Thank you for visiting our website which covers about The Array Perimeter Should Not Be Empty . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.