Part A Drag The Appropriate Labels To Their Respective Targets
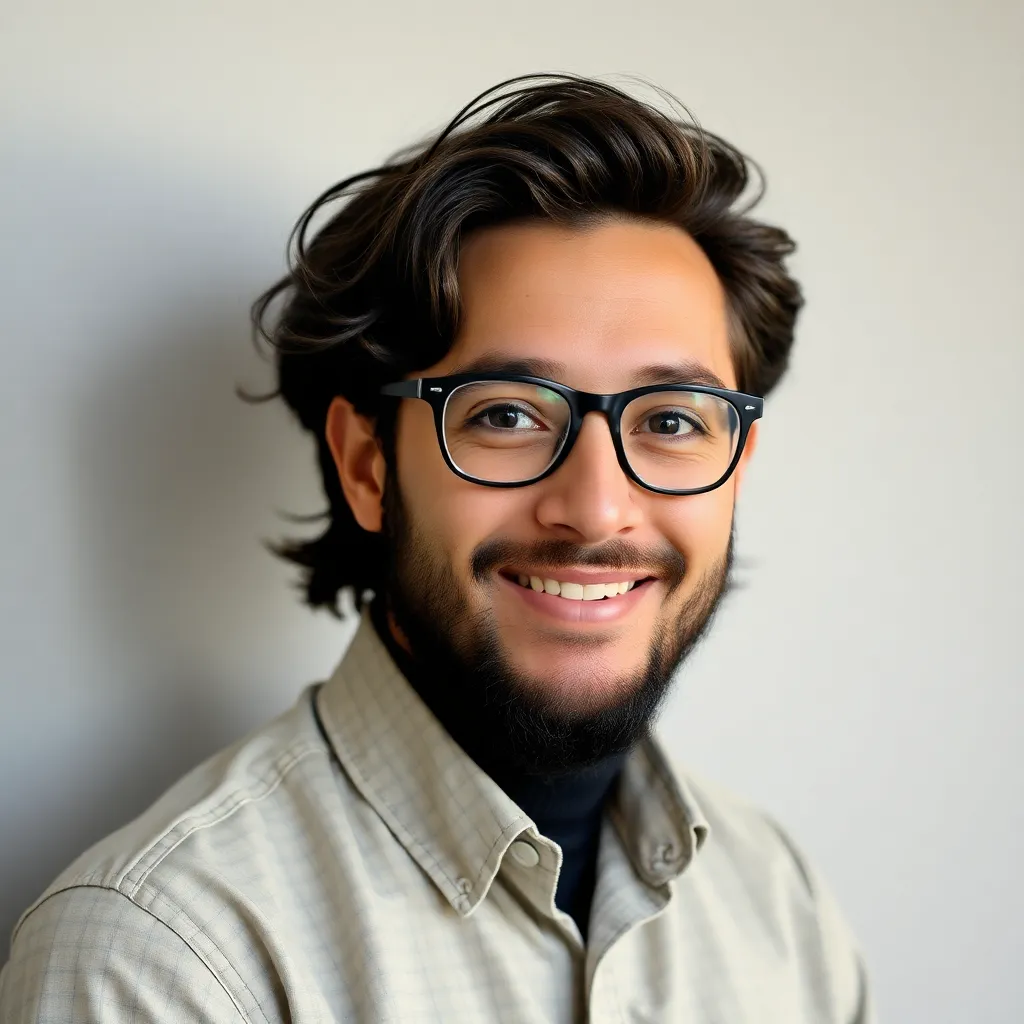
Holbox
May 12, 2025 · 7 min read
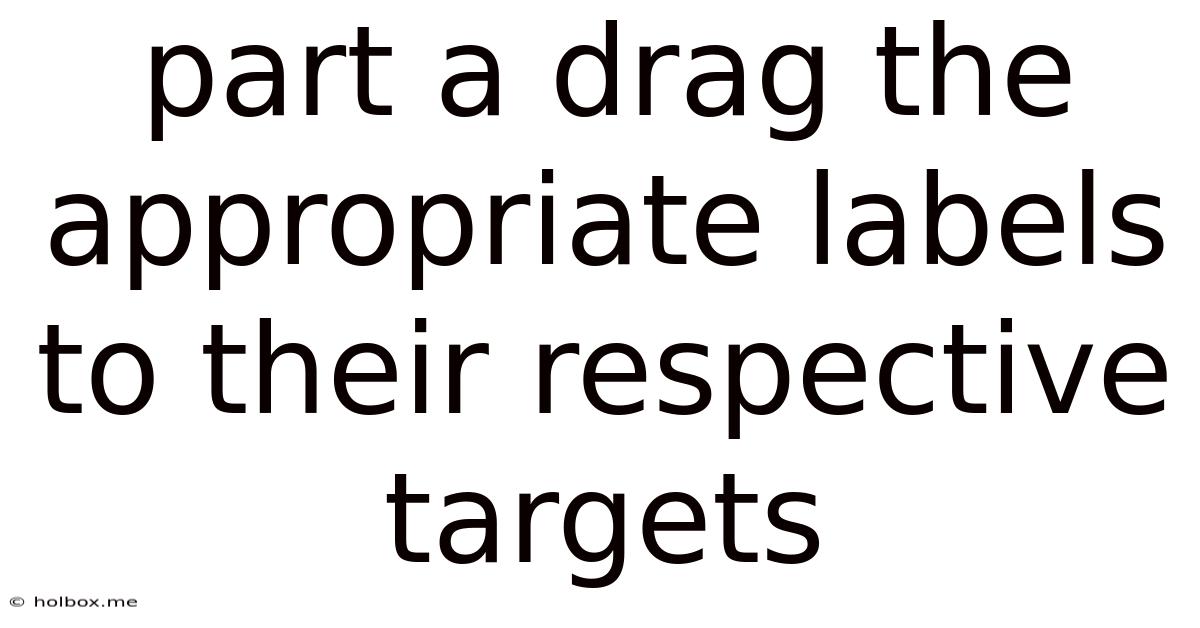
Table of Contents
- Part A Drag The Appropriate Labels To Their Respective Targets
- Table of Contents
- Mastering the Art of Drag-and-Drop Interactions: A Comprehensive Guide
- Part A: Understanding the Fundamentals of Drag-and-Drop
- Part B: Implementing Drag-and-Drop Interactions: A Practical Approach
- Part C: Advanced Techniques and Considerations
- Part D: Optimizing Drag-and-Drop for User Experience
- Part E: Troubleshooting Common Challenges
- Conclusion
- Latest Posts
- Related Post
Mastering the Art of Drag-and-Drop Interactions: A Comprehensive Guide
Drag-and-drop interactions are ubiquitous in modern user interfaces. From sorting items in a to-do list to arranging furniture in a virtual room, this intuitive method of interaction simplifies complex tasks and enhances user experience. However, the seemingly simple act of dragging and dropping involves a sophisticated interplay of programming logic and user interface design. This comprehensive guide delves deep into the intricacies of drag-and-drop functionality, covering everything from the basic principles to advanced techniques. We will explore how to implement effective drag-and-drop interactions, optimize them for user experience, and address potential challenges in different contexts.
Part A: Understanding the Fundamentals of Drag-and-Drop
Before diving into the complexities, it's crucial to grasp the fundamental principles governing drag-and-drop interactions. These principles form the bedrock upon which more advanced techniques are built.
1. The Drag Initiation: The process begins when a user initiates a drag operation. This typically involves clicking and holding on a draggable element. The element then becomes a "drag source," ready to be moved. The exact method of initiating a drag varies depending on the platform (web, desktop) and the specific library or framework used.
2. The Drag Operation: Once initiated, the drag operation is characterized by the movement of the drag source element. This movement is usually tracked by the user's mouse or touch input. During this phase, visual feedback, such as a shadow or highlight, is often provided to indicate the element being dragged. This visual feedback is crucial for maintaining user context and providing a smooth interactive experience.
3. The Drop Target: The drop target is the area or element where the user intends to drop the dragged element. This could be a specific container, a particular region on the screen, or even another element within the interface. The target needs to be clearly identifiable to the user, often through visual cues such as highlighting or change in opacity upon the dragged item's hover.
4. The Drop Action: When the user releases the mouse button or lifts their finger (on a touch device), the drag operation ends. At this point, the drop action is triggered. This is where the application logic determines whether to accept the dropped element and how to handle it. This could involve adding the element to the target, rearranging the order of elements, or performing other actions depending on the context.
5. Feedback Mechanisms: Crucial to a positive user experience is providing consistent and informative feedback throughout the drag-and-drop process. This includes:
- Visual Cues: Highlights, shadows, and other visual changes on both the drag source and drop target.
- Haptic Feedback (for touch devices): Subtle vibrations to confirm actions.
- Auditory Feedback: Subtle sounds to indicate success or failure of a drop.
Part B: Implementing Drag-and-Drop Interactions: A Practical Approach
Implementing drag-and-drop functionality involves a combination of HTML, CSS, and JavaScript. The specific approach will vary based on the chosen framework or library.
1. HTML Structure: The HTML structure defines the draggable elements and potential drop targets. Crucially, the draggable
attribute is set to true
for elements intended to be dragged. The drop targets might require specific classes or IDs for easy identification in the JavaScript code.
Example:
Item 1
Item 2
Item 3
Drop here
2. CSS Styling: CSS is used to style the elements and provide visual feedback during the drag-and-drop operation. This might include styling the drag shadow, highlighting the drop target, and indicating acceptance or rejection of a drop.
Example:
.draggable {
border: 1px solid #ccc;
padding: 10px;
margin: 5px;
cursor: move; /* Indicate draggability */
}
#drop-target {
border: 2px dashed #ccc;
padding: 20px;
margin-top: 20px;
}
#drop-target.over { /* Style when drag is over */
border-color: #090;
}
3. JavaScript Event Handlers: JavaScript event handlers manage the drag-and-drop process. Key events include:
dragstart
: Fired when the drag operation starts. This is where data is often set for transfer to the drop target.drag
: Fired repeatedly during the drag operation. Used to update visual feedback.dragenter
: Fired when the dragged element enters the drop target.dragover
: Fired repeatedly while the dragged element is over the drop target. This event needs to havepreventDefault()
called to allow the drop.dragleave
: Fired when the dragged element leaves the drop target.drop
: Fired when the dragged element is dropped onto the drop target. This is where the actual drop action logic is executed.dragend
: Fired when the drag operation ends.
Example JavaScript (simplified):
let draggedItem = null;
document.querySelectorAll('.draggable').forEach(item => {
item.addEventListener('dragstart', (e) => {
draggedItem = e.target;
e.dataTransfer.setData('text/plain', e.target.textContent); // Transfer data
});
});
document.getElementById('drop-target').addEventListener('dragover', (e) => {
e.preventDefault(); // Allow drop
e.target.classList.add('over');
});
document.getElementById('drop-target').addEventListener('dragleave', (e) => {
e.target.classList.remove('over');
});
document.getElementById('drop-target').addEventListener('drop', (e) => {
e.preventDefault();
e.target.classList.remove('over');
e.target.appendChild(draggedItem);
});
Part C: Advanced Techniques and Considerations
While the basic implementation provides core drag-and-drop functionality, several advanced techniques can significantly enhance the user experience and expand the capabilities.
1. Data Transfer: The dataTransfer
object allows you to transfer data between the drag source and the drop target. This can be text, URLs, or custom data. This is essential for complex scenarios where more than just moving elements is required.
2. Drag-and-Drop Libraries and Frameworks: Libraries such as jQuery UI, React DnD, and Vue.Draggable simplify drag-and-drop implementation, providing pre-built components and functionalities that handle complexities like browser inconsistencies.
3. Custom Drag Handles: Instead of allowing the entire element to be dragged, you can specify a smaller area (e.g., a small icon) as the drag handle. This improves usability, especially with smaller elements.
4. Constraining Drag Movement: You can restrict drag movement to specific areas of the screen or within a container. This is crucial for scenarios like arranging items within a defined grid.
5. Drag-and-Drop with Multiple Targets: Allowing an element to be dropped onto multiple targets expands the possibilities. This necessitates more sophisticated logic to handle the drop action based on the target.
Part D: Optimizing Drag-and-Drop for User Experience
The success of drag-and-drop interactions heavily relies on optimizing for a smooth and intuitive user experience.
1. Clear Visual Feedback: Maintain consistent and informative visual feedback throughout the entire process. Use highlights, shadows, and animations to clearly indicate the dragged element and potential drop targets.
2. Intuitive Drag Handles: Use clear and obvious drag handles that are easy to identify and interact with. Avoid overly small or obscure handles.
3. Smooth Animations: Use smooth animations to guide the user's eye and provide a visually appealing experience. Avoid jerky or abrupt movements.
4. Prevent Unintended Drops: Implement mechanisms to prevent accidental drops in unintended locations. Consider providing confirmation dialogs for potentially destructive actions.
5. Accessibility Considerations: Ensure accessibility for users with disabilities. This might involve using keyboard navigation and screen reader-friendly labels.
6. Performance Optimization: For large datasets or complex interactions, optimize performance to avoid lag or delays. Use efficient algorithms and techniques to minimize computational overhead.
Part E: Troubleshooting Common Challenges
Implementing drag-and-drop can encounter various challenges.
1. Browser Compatibility: Different browsers handle drag-and-drop events slightly differently. Thorough testing across various browsers is crucial.
2. Event Order Issues: Unexpected behavior can result from incorrect event order handling. Carefully understand and handle events in the correct sequence.
3. Data Transfer Errors: Errors during data transfer can lead to unexpected results. Ensure proper data encoding and handling.
Conclusion
Mastering drag-and-drop interactions requires a deep understanding of both user interface design principles and underlying programming techniques. By focusing on user experience and utilizing best practices, developers can create intuitive and effective interfaces that enhance user engagement and overall productivity. This guide has provided a comprehensive overview of the key aspects involved, from foundational concepts to advanced techniques and troubleshooting strategies. The journey to creating compelling and efficient drag-and-drop interactions demands continuous learning and refinement, but the rewards of an intuitive and effective user experience are significant. Remember to always prioritize user experience and optimize your implementation for different devices and browsers to provide the best possible interaction.
Latest Posts
Related Post
Thank you for visiting our website which covers about Part A Drag The Appropriate Labels To Their Respective Targets . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.