Nested Loop Java Ascll Art Pictures
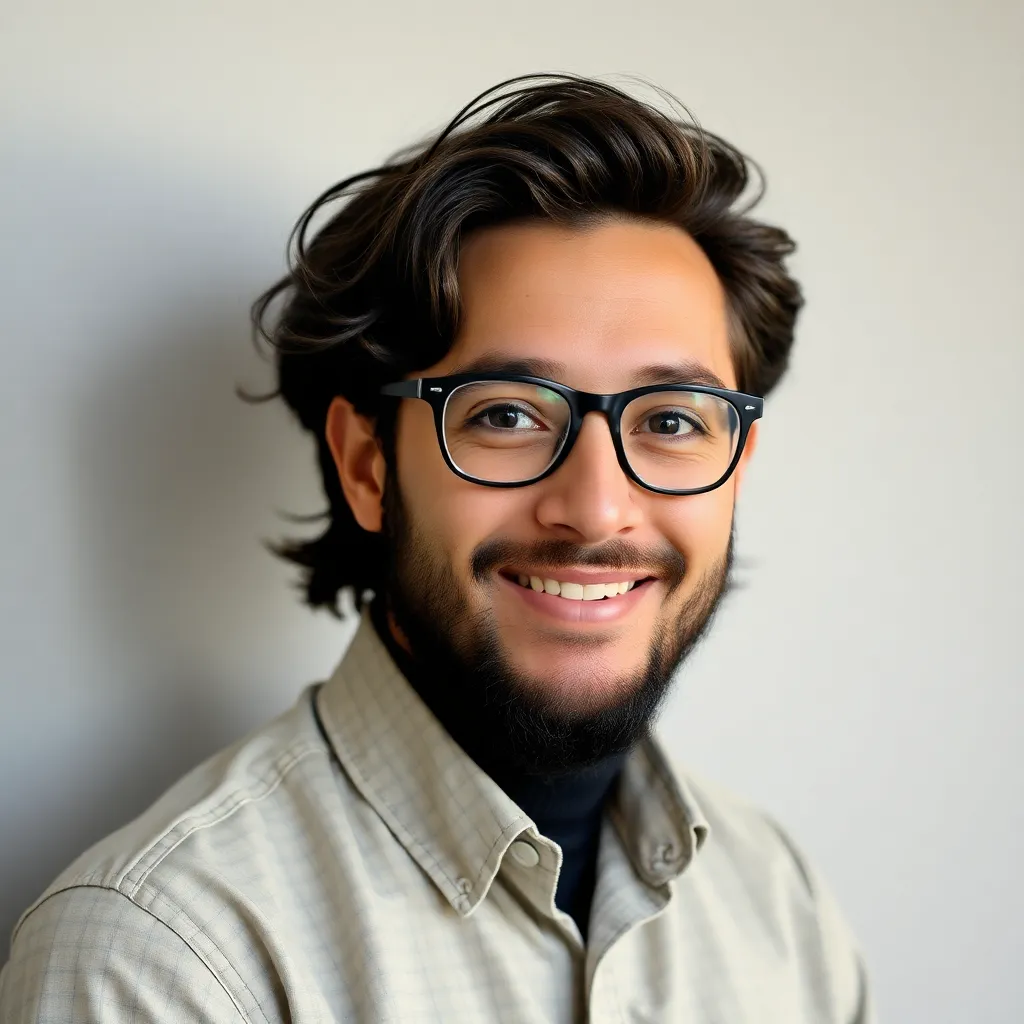
Holbox
Apr 02, 2025 · 5 min read
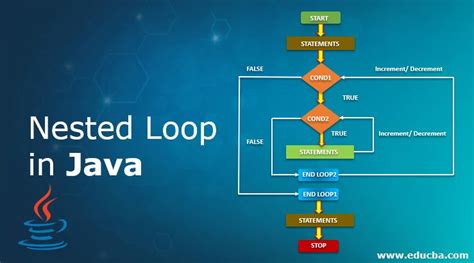
Table of Contents
- Nested Loop Java Ascll Art Pictures
- Table of Contents
- Nested Loops in Java: Crafting ASCII Art Pictures
- Understanding Nested Loops
- Building Simple ASCII Art
- A Simple Square
- A Right-Angled Triangle
- An Isosceles Triangle
- Enhancing the Art: Beyond Basic Shapes
- Adding Patterns
- Using Different Characters
- Latest Posts
- Latest Posts
- Related Post
- Nested Loop Java Ascll Art Pictures
- Table of Contents
- Nested Loops in Java: Crafting ASCII Art Pictures
- Understanding Nested Loops
- Building Simple ASCII Art
- A Simple Square
- A Right-Angled Triangle
- An Isosceles Triangle
- Enhancing the Art: Beyond Basic Shapes
- Adding Patterns
- Using Different Characters
- Latest Posts
- Latest Posts
- Related Post
Nested Loops in Java: Crafting ASCII Art Pictures
Java, renowned for its versatility and power, offers numerous avenues for creative coding. One particularly fascinating application is generating ASCII art using nested loops. This technique, while seemingly simple, allows for the creation of complex and visually appealing patterns, offering a unique blend of programming logic and artistic expression. This comprehensive guide will delve deep into the world of nested loops in Java and demonstrate how to leverage them to design a wide array of ASCII art pictures. We'll cover fundamental concepts, explore various examples, and touch upon advanced techniques to enhance your artistic coding capabilities.
Understanding Nested Loops
Before embarking on our artistic journey, let's establish a strong foundation in nested loops. In Java (and most programming languages), a nested loop is a loop placed inside another loop. The inner loop completes all its iterations for each iteration of the outer loop. This creates a powerful mechanism for iterating over multiple dimensions of data, a crucial element in generating intricate patterns. Consider the following example:
for (int i = 0; i < 5; i++) { // Outer loop
for (int j = 0; j < 10; j++) { // Inner loop
System.out.print("*");
}
System.out.println();
}
This code will print a rectangle of asterisks, 5 rows high and 10 columns wide. The outer loop controls the rows, while the inner loop controls the columns. Each time the outer loop iterates, the inner loop runs completely, printing a row of asterisks before moving to the next row.
Building Simple ASCII Art
Now, let's transition from basic loop understanding to creating actual ASCII art. We'll start with straightforward examples, gradually increasing complexity to showcase the versatility of nested loops.
A Simple Square
Building upon the previous example, we can easily modify the code to create a square of any size:
import java.util.Scanner;
public class SquareArt {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the side length of the square: ");
int sideLength = scanner.nextInt();
for (int i = 0; i < sideLength; i++) {
for (int j = 0; j < sideLength; j++) {
System.out.print("*");
}
System.out.println();
}
scanner.close();
}
}
This program takes user input to determine the size of the square, making it more interactive and dynamic.
A Right-Angled Triangle
Let's create a right-angled triangle using nested loops:
public class TriangleArt {
public static void main(String[] args) {
int height = 5;
for (int i = 1; i <= height; i++) {
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
Here, the inner loop's iteration count depends on the outer loop's counter (i
), creating the triangular shape. The number of asterisks printed in each row increases progressively.
An Isosceles Triangle
Creating an isosceles triangle requires a slightly more sophisticated approach:
public class IsoscelesTriangle {
public static void main(String[] args) {
int height = 5;
for (int i = 1; i <= height; i++) {
for (int j = 1; j <= height - i; j++) {
System.out.print(" ");
}
for (int k = 1; k <= 2 * i - 1; k++) {
System.out.print("*");
}
System.out.println();
}
}
}
This example uses three nested loops. The first loop prints leading spaces to center the triangle, the second prints the asterisks for the left half, and an implicit loop within the second completes the right half (mirroring the left). This illustrates how multiple nested loops can be used to create more intricate patterns.
Enhancing the Art: Beyond Basic Shapes
The power of nested loops truly shines when we move beyond simple shapes and incorporate more complex patterns and characters.
Adding Patterns
Let's create a checkered pattern:
public class CheckeredPattern {
public static void main(String[] args) {
int size = 10;
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
if ((i + j) % 2 == 0) {
System.out.print("*");
} else {
System.out.print("#");
}
}
System.out.println();
}
}
}
This code uses a conditional statement inside the inner loop to alternate between two characters, creating a visually interesting checkerboard effect.
Using Different Characters
You're not limited to asterisks. Experiment with different characters to create unique visual effects. For instance:
public class CharacterArt {
public static void main(String[] args) {
int size = 5;
char[] chars = {'@', '#', '
Latest Posts
Latest Posts
-
What Event Occurred During This Cycle Of Meiosis
Apr 04, 2025
-
Arbitration Clauses Are Generally Enforced If
Apr 04, 2025
-
What Is The Lewis Dot Structure For Ci
Apr 04, 2025
-
Government Rather Than Private Business Provides National Defense Because
Apr 04, 2025
-
Select The True Statements About The Citric Acid Cycle
Apr 04, 2025
Related Post
Thank you for visiting our website which covers about
Nested Loop Java Ascll Art Pictures .
We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.