Many Pathways From One Node To Another
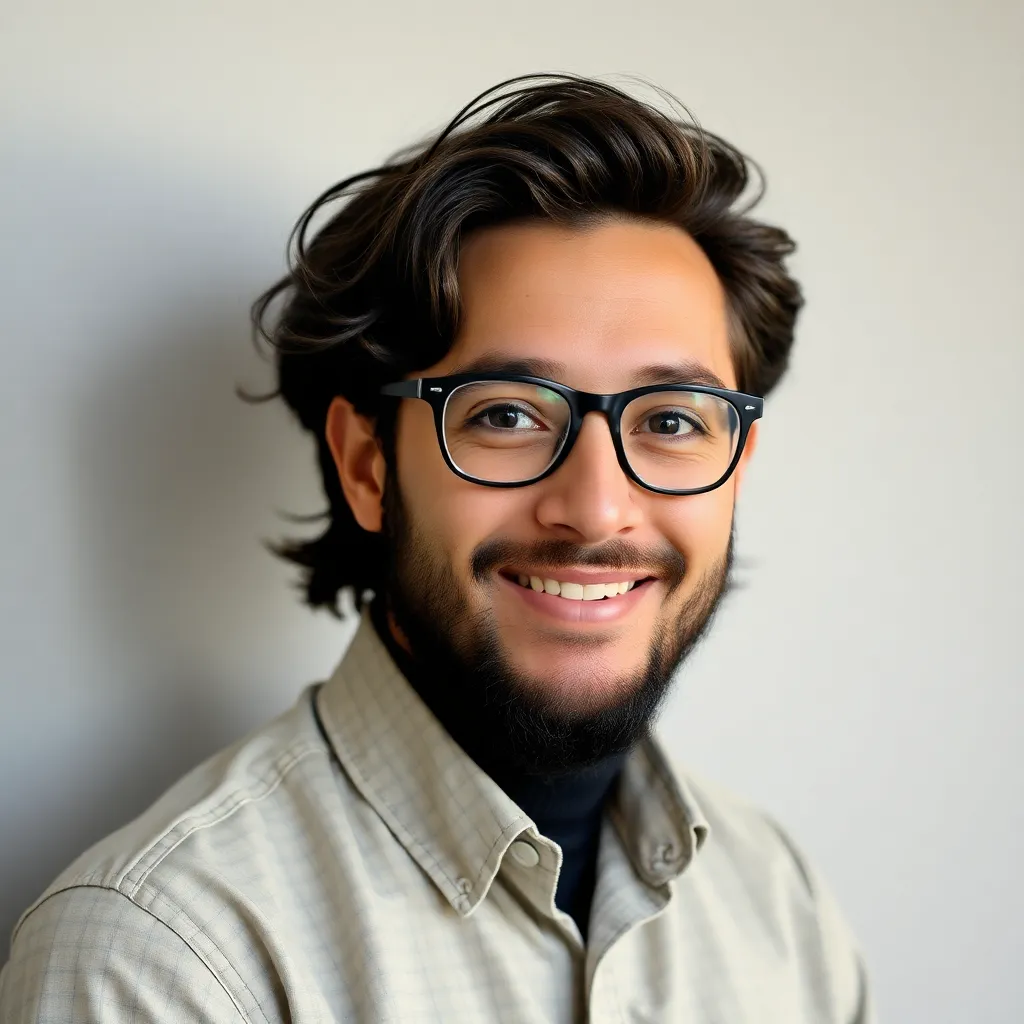
Holbox
May 10, 2025 · 7 min read
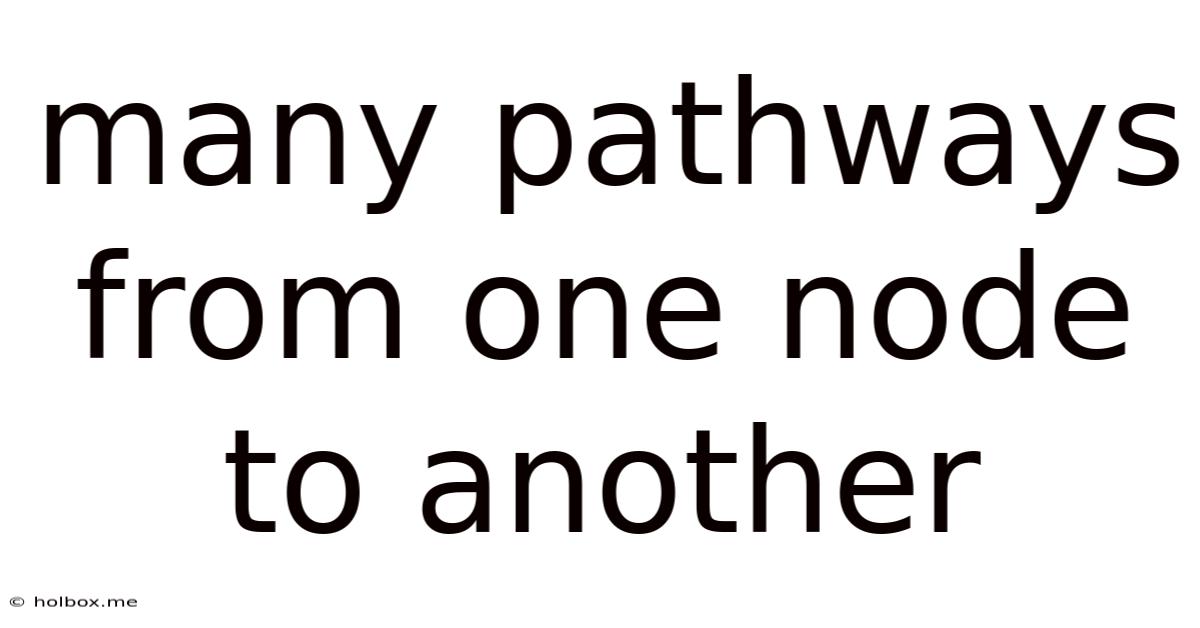
Table of Contents
- Many Pathways From One Node To Another
- Table of Contents
- Many Pathways From One Node to Another: Exploring Graph Traversal Algorithms
- Understanding Graph Terminology
- Exploring Key Graph Traversal Algorithms
- 1. Breadth-First Search (BFS)
- 2. Depth-First Search (DFS)
- 3. Dijkstra's Algorithm
- 4. A* Search Algorithm
- Choosing the Right Algorithm
- Advanced Considerations and Optimizations
- Real-World Applications
- Conclusion
- Latest Posts
- Related Post
Many Pathways From One Node to Another: Exploring Graph Traversal Algorithms
The concept of navigating from one point to another is fundamental across numerous fields. Whether it's finding the shortest route on a map, tracing connections in a social network, or determining dependencies in a complex system, the underlying structure often resembles a graph: a collection of nodes (vertices) connected by edges. This article delves into the fascinating world of graph traversal, focusing on the various algorithms that allow us to explore the multitude of pathways between any two nodes within a graph. We'll examine their strengths, weaknesses, and suitability for different applications.
Understanding Graph Terminology
Before diving into the algorithms, let's establish a common understanding of key graph concepts.
-
Node (Vertex): A fundamental unit representing an entity in the graph. Think of cities on a map, users on a social network, or files in a file system.
-
Edge: A connection between two nodes. Edges can be directed (one-way) or undirected (two-way). In a map, an edge represents a road; in a social network, it represents a friendship.
-
Path: A sequence of nodes connected by edges, starting from a source node and ending at a destination node.
-
Weighted Graph: A graph where edges have associated weights, representing distance, cost, or any other relevant metric. In a map, the weight might be the distance between cities.
-
Unweighted Graph: A graph where edges have no associated weights. Each edge is treated equally.
-
Directed Acyclic Graph (DAG): A directed graph with no cycles (paths that start and end at the same node). This type of graph is often used to represent dependencies.
Exploring Key Graph Traversal Algorithms
Several algorithms efficiently explore the numerous pathways between nodes in a graph. Let's examine some of the most prominent ones:
1. Breadth-First Search (BFS)
BFS systematically explores a graph level by level. It starts at the source node and visits all its neighbors before moving to their neighbors, ensuring that nodes closer to the source are visited first. This algorithm is particularly useful for finding the shortest path in unweighted graphs.
Algorithm Steps:
- Initialization: Create a queue, add the source node to the queue, and mark it as visited.
- Iteration: While the queue is not empty:
- Dequeue a node.
- Process the node (e.g., add it to a path).
- Enqueue all unvisited neighbors of the node and mark them as visited.
- Termination: The algorithm terminates when the queue is empty, or when the destination node is found (depending on the application).
Strengths:
- Finds the shortest path in unweighted graphs.
- Relatively simple to implement.
Weaknesses:
- Can be memory-intensive for large graphs, as it needs to store all nodes at a given level.
- Not optimal for weighted graphs.
2. Depth-First Search (DFS)
DFS explores a graph by going as deep as possible along each branch before backtracking. It uses a stack (implicitly or explicitly) to keep track of the nodes to visit. This algorithm is often used for topological sorting and detecting cycles in graphs.
Algorithm Steps:
- Initialization: Create a stack (or use recursion), add the source node to the stack, and mark it as visited.
- Iteration: While the stack is not empty:
- Pop a node from the stack.
- Process the node.
- Push all unvisited neighbors of the node onto the stack and mark them as visited.
- Termination: The algorithm terminates when the stack is empty, or when the destination node is found.
Strengths:
- Requires less memory than BFS in some cases.
- Suitable for detecting cycles and topological sorting.
Weaknesses:
- Doesn't guarantee finding the shortest path.
- Can get stuck in infinite loops in graphs with cycles.
3. Dijkstra's Algorithm
Dijkstra's algorithm is a powerful algorithm for finding the shortest path in weighted graphs with non-negative edge weights. It uses a priority queue to efficiently select the node with the smallest distance from the source.
Algorithm Steps:
- Initialization: Assign a tentative distance value to every node: set it to zero for the source node and to infinity for all other nodes. Set the source node as current.
- Iteration: For the current node, consider all of its unvisited neighbors and calculate their tentative distances through the current node. Compare the newly calculated tentative distance to the current assigned value and assign the smaller one. When we are done considering all of the unvisited neighbors of the current node, mark the current node as visited. A visited node will never be checked again.
- Selection: Select the unvisited node that is marked with the smallest tentative distance, set it as the new "current node," and go back to step 2.
- Termination: The algorithm terminates when the destination node is visited or when all the nodes have been visited.
Strengths:
- Finds the shortest path in weighted graphs with non-negative edge weights.
- Efficiently handles weighted edges.
Weaknesses:
- Doesn't work with graphs containing negative edge weights.
4. A* Search Algorithm
A* search is an informed search algorithm that combines the best features of BFS and Dijkstra's algorithm. It uses a heuristic function to estimate the distance from a node to the destination, guiding the search towards promising paths. This makes it particularly efficient for large graphs.
Algorithm Steps:
- Initialization: Similar to Dijkstra's algorithm, assign a tentative distance to each node, but A* also incorporates a heuristic estimate (h(n)) of the distance from the node to the goal. The total cost (f(n)) is calculated as g(n) + h(n), where g(n) is the actual cost from the source to node n.
- Iteration: Use a priority queue ordered by f(n) to select the node with the lowest estimated total cost.
- Expansion: Explore the neighbors of the selected node, updating their g(n) and f(n) values.
- Termination: The algorithm terminates when the destination node is reached.
Strengths:
- Very efficient for large graphs due to its heuristic guidance.
- Finds optimal paths in many cases.
Weaknesses:
- The performance heavily depends on the quality of the heuristic function. A poorly chosen heuristic can lead to suboptimal results.
Choosing the Right Algorithm
The choice of algorithm depends heavily on the characteristics of the graph and the specific problem being solved.
- Unweighted graphs, shortest path: BFS is the clear choice.
- Weighted graphs with non-negative weights, shortest path: Dijkstra's algorithm is the most appropriate.
- Weighted graphs, shortest path, large graphs: A* search offers excellent performance with a well-chosen heuristic.
- Finding all paths: While the algorithms above can be modified to find all paths (with potential performance implications for very large graphs), specialized algorithms for enumerating all paths might be more efficient.
- Topological sorting, cycle detection: DFS is highly effective.
Advanced Considerations and Optimizations
The algorithms discussed above can be further optimized and enhanced for specific applications. Some notable enhancements include:
-
Heuristic Functions in A Search:* Developing effective heuristic functions is crucial for A*'s performance. Different heuristics can be tailored to specific problem domains.
-
Bidirectional Search: For finding the shortest path between two nodes, bidirectional search can significantly reduce the search space by simultaneously exploring paths from both the source and the destination.
-
Memory Management: For large graphs, efficient memory management techniques are essential to prevent memory exhaustion.
-
Parallel Processing: Graph traversal algorithms can be parallelized to leverage multi-core processors for improved performance, particularly for large-scale graphs.
Real-World Applications
The ability to efficiently traverse graphs and find paths has numerous applications across diverse domains:
- Navigation Systems: Finding the shortest route between two locations using GPS data.
- Social Network Analysis: Analyzing connections and relationships between users in social networks.
- Recommendation Systems: Identifying items or users that are closely related based on their connections.
- Network Routing: Determining efficient paths for data packets in computer networks.
- Robotics: Planning paths for robots to navigate in complex environments.
- Bioinformatics: Analyzing biological networks and pathways.
- Game AI: Pathfinding for characters in video games.
Conclusion
Graph traversal algorithms are fundamental tools for navigating and analyzing interconnected data. Understanding the strengths and weaknesses of different algorithms, such as Breadth-First Search, Depth-First Search, Dijkstra's algorithm, and A* search, allows us to choose the most appropriate method for a given problem. Furthermore, exploring advanced optimizations and considering the unique aspects of the problem domain can lead to efficient and effective solutions for a wide range of real-world applications. The ongoing development and refinement of these algorithms continue to push the boundaries of what's possible in diverse fields, enabling better navigation, analysis, and decision-making in increasingly complex systems.
Latest Posts
Related Post
Thank you for visiting our website which covers about Many Pathways From One Node To Another . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.