In Order To Move The Ovals
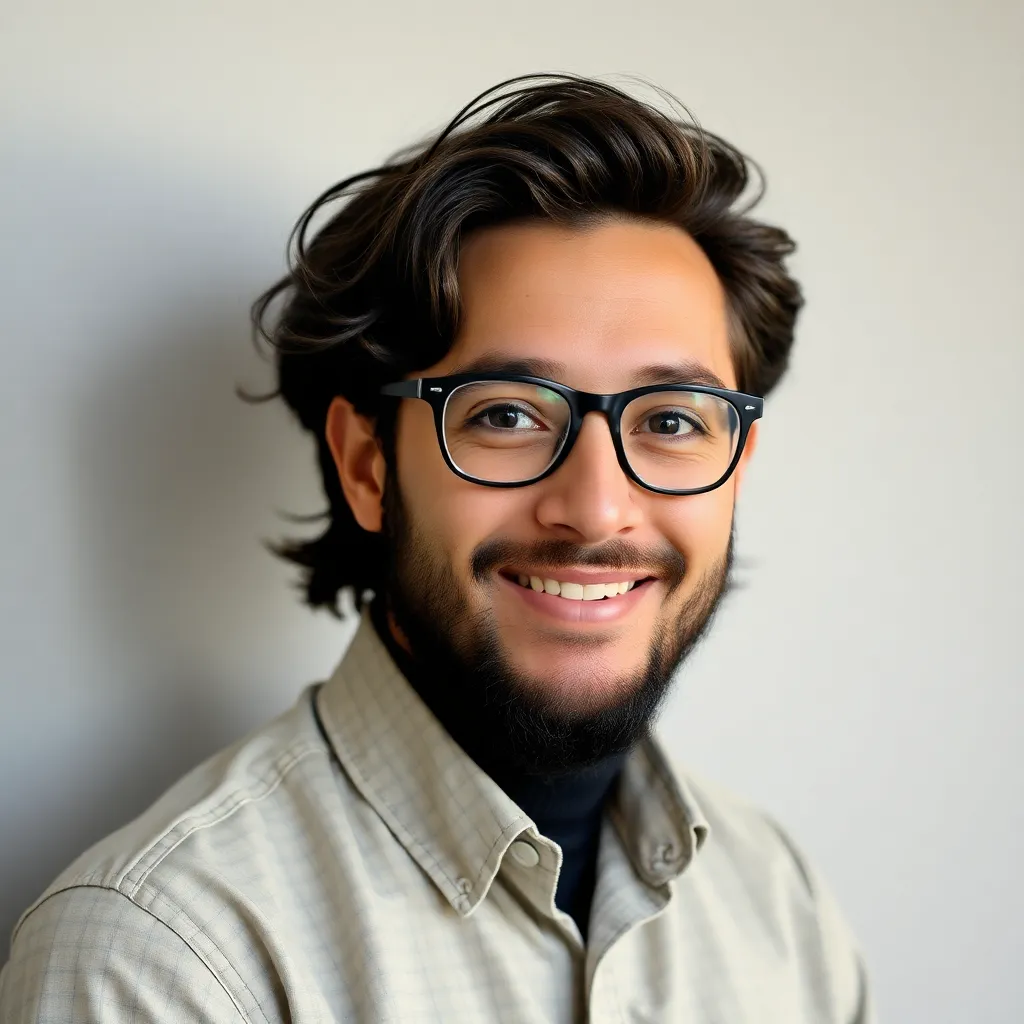
Holbox
Apr 03, 2025 · 6 min read
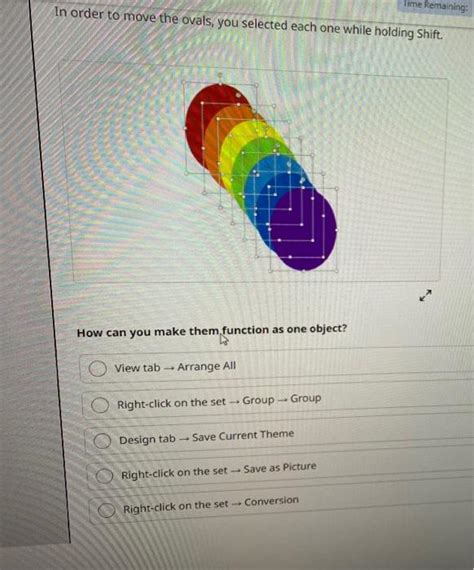
Table of Contents
- In Order To Move The Ovals
- Table of Contents
- In Order to Move the Ovals: A Deep Dive into Object Manipulation and Animation
- Understanding the Fundamentals: Position and Transformation
- Coordinate Systems: The Foundation of Positioning
- Transformation Matrices: The Engine of Movement
- Animation Techniques: Bringing the Oval to Life
- Keyframe Animation: Defining Milestones
- Procedural Animation: Algorithmic Control
- Physics-Based Animation: Real-World Simulation
- Implementing Movement: Code Examples (Conceptual)
- Optimization Strategies: Efficiency in Movement
- Beyond Simple Movement: Advanced Techniques
- Conclusion: The Power of Oval Manipulation
- Latest Posts
- Latest Posts
- Related Post
In Order to Move the Ovals: A Deep Dive into Object Manipulation and Animation
Moving ovals, seemingly a simple task, reveals a surprisingly rich landscape of concepts relevant to computer graphics, animation, game development, and even robotics. This seemingly simple action involves understanding fundamental principles of object manipulation, coordinate systems, transformation matrices, animation techniques, and optimization strategies. This in-depth exploration will delve into each of these areas, examining both the theoretical foundations and practical implementations.
Understanding the Fundamentals: Position and Transformation
Before we can move an oval, we need to understand its representation within a digital environment. Typically, an oval (or ellipse) is defined by its center point (x, y coordinates) and its major and minor radii (a and b). These parameters define the shape and position of the oval in a two-dimensional space.
Coordinate Systems: The Foundation of Positioning
Every object within a digital scene exists within a specific coordinate system. The most common is the Cartesian coordinate system, where objects are located based on their x and y (and sometimes z for 3D) coordinates relative to an origin (0,0). Understanding the coordinate system is crucial because moving the oval involves changing its coordinates within this system.
Transformation Matrices: The Engine of Movement
Moving an oval isn't just about changing its x and y coordinates directly. It's about applying transformations to its position. Transformation matrices are powerful tools for representing these transformations concisely and efficiently. A common transformation is translation, which involves shifting the oval's position by a certain amount along the x and y axes. This is achieved using a translation matrix that adds the desired offsets to the oval's coordinates.
Example (using matrix notation):
Let's say we want to move the oval from (x, y) to (x + dx, y + dy). The translation matrix would be:
[ 1 0 dx ]
[ 0 1 dy ]
[ 0 0 1 ]
Multiplying this matrix with the oval's coordinate vector will yield the new position.
Beyond translation, other transformations include:
- Rotation: Changing the oval's orientation around a point. Rotation matrices involve trigonometric functions (sine and cosine).
- Scaling: Changing the size of the oval by altering its radii (a and b). Scaling matrices involve scaling factors along the x and y axes.
- Shearing: Distorting the oval by skewing it along one or both axes.
Combining these transformations allows for complex movements and manipulations. The order of applying these transformations matters; matrix multiplication is not commutative.
Animation Techniques: Bringing the Oval to Life
Moving the oval statically is one thing, but animating its movement adds a dynamic element. Several techniques can be used to create animated movements:
Keyframe Animation: Defining Milestones
Keyframe animation involves defining the oval's position and other properties (like size, rotation) at specific points in time (keyframes). Interpolation algorithms then determine the oval's position and properties between these keyframes, creating a smooth animation. Linear interpolation is simple, but more sophisticated algorithms like Bezier curves or splines can produce smoother, more natural-looking movements.
Procedural Animation: Algorithmic Control
Procedural animation uses algorithms to define the oval's movement. This allows for more control and flexibility, as the oval's path can be defined mathematically, making it ideal for generating complex or repetitive movements. Examples include:
- Circular Motion: The oval's position can be calculated using trigonometric functions to create circular or elliptical paths.
- Parabolic Motion: Simulating projectile motion using physics equations can create realistic movements.
- Following a Path: Defining a path (a series of points) and using interpolation to make the oval smoothly follow this path.
Physics-Based Animation: Real-World Simulation
Physics-based animation uses physics engines to simulate real-world forces and interactions, making the oval's movement more realistic. This is particularly relevant in games and simulations, where gravity, collisions, and other physical phenomena affect object movement.
Implementing Movement: Code Examples (Conceptual)
While specific implementation details vary based on the programming language and graphics library used, the fundamental concepts remain consistent. Here are conceptual examples to illustrate the core ideas:
Conceptual Python Example (using hypothetical libraries):
# Initialize oval object with position and radii
oval = Oval(x=100, y=100, a=50, b=30)
# Translate the oval
oval.translate(dx=20, dy=30) # Move 20 units right, 30 units down
# Rotate the oval
oval.rotate(angle=45) # Rotate 45 degrees counter-clockwise
# Animate the oval (simple linear interpolation)
for i in range(100):
oval.x += 1 # Move 1 unit right in each frame
oval.draw() # Redraw the oval in its new position
time.sleep(0.05) # Pause for smoothness
Conceptual JavaScript Example (using hypothetical libraries):
// Initialize oval object with position and radii
let oval = new Oval(100, 100, 50, 30);
// Translate the oval
oval.translate(20, 30);
// Animate the oval (using requestAnimationFrame for smooth animation)
function animate() {
oval.x += 1;
oval.draw();
requestAnimationFrame(animate);
}
animate();
These are highly simplified representations. Real-world implementations will involve more complex structures, data management, and potentially 3D graphics libraries.
Optimization Strategies: Efficiency in Movement
Moving numerous ovals, or animating complex movements, can be computationally expensive. Optimization strategies are crucial for maintaining smooth performance:
- Spatial Partitioning: Divide the scene into smaller regions, making it easier to identify objects that interact with each other, reducing unnecessary calculations.
- Culling: Hide objects that are not visible to the camera, reducing the number of objects that need to be rendered.
- Level of Detail (LOD): Use simpler representations of objects when they are far away from the camera, improving performance without noticeably affecting visual quality.
- Efficient Data Structures: Use data structures that allow for fast access and manipulation of object properties.
Beyond Simple Movement: Advanced Techniques
The ability to move ovals forms a basis for many advanced techniques:
- Simulation: Moving ovals can be part of a larger simulation, such as simulating particles, fluid dynamics, or complex physical systems.
- Game Development: Moving ovals is fundamental to game development, enabling the creation of interactive characters, objects, and game elements.
- Robotics: The principles of object manipulation and animation are directly applicable in robotics, controlling the movement of robots and robotic arms.
- Virtual Reality (VR) and Augmented Reality (AR): The ability to create realistic and interactive movements of objects is vital in VR and AR applications, immersing users in virtual environments.
Conclusion: The Power of Oval Manipulation
The seemingly simple act of moving ovals unlocks a wealth of computational power and creative possibilities. From fundamental coordinate systems and transformation matrices to sophisticated animation techniques and optimization strategies, understanding these concepts lays a solid foundation for advanced applications in computer graphics, animation, and related fields. The ability to manipulate and animate objects effectively is crucial for creating engaging and realistic experiences, regardless of the application. This exploration has only scratched the surface; continuous learning and experimentation are key to mastering these powerful techniques.
Latest Posts
Latest Posts
-
A Change In What Component Of Microscopy Influences
Apr 06, 2025
-
Which Of The Following Is An Example Of A Reflex
Apr 06, 2025
-
A Workstation Is Out Of Compliance
Apr 06, 2025
-
Gold Forms A Substitutional Solid Solution With Silver
Apr 06, 2025
-
Over Longer Periods Of Time Demand Tends To Become
Apr 06, 2025
Related Post
Thank you for visiting our website which covers about In Order To Move The Ovals . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.