Import The Text File Paty Matchups Txt As A Table
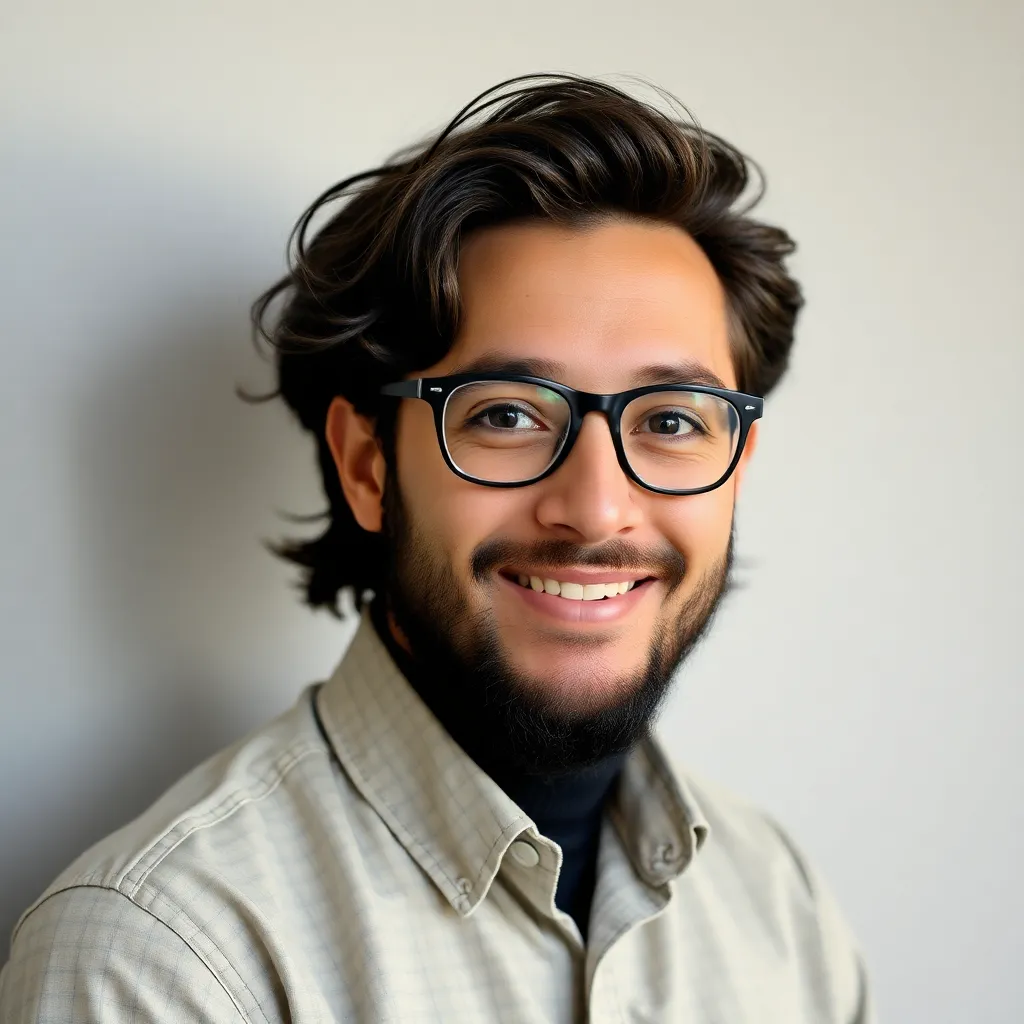
Holbox
Mar 13, 2025 · 6 min read

Table of Contents
- Import The Text File Paty Matchups Txt As A Table
- Table of Contents
- Importing the "party_matchups.txt" File as a Table: A Comprehensive Guide
- Understanding the Data: Structure and Challenges
- Importing the Data: Different Approaches
- Method 1: Python with the csv module (for CSV and TSV)
- Method 2: Python with pandas (for various formats)
- Method 3: SQL (for structured data)
- Method 4: Handling Irregular Formats (Python with Regular Expressions)
- Data Cleaning and Validation
- Choosing the Right Approach
- Latest Posts
- Latest Posts
- Related Post
Importing the "party_matchups.txt" File as a Table: A Comprehensive Guide
This article provides a detailed walkthrough of importing a text file named "party_matchups.txt" into a tabular format suitable for analysis and manipulation. We'll cover various approaches, from simple scripting using Python to leveraging the capabilities of specialized data analysis tools like pandas and SQL. We will assume the file contains data representing political party matchups, but the techniques discussed are applicable to any similarly structured text file. The focus is on efficient and robust methods suitable for datasets of varying sizes and complexities.
Understanding the Data: Structure and Challenges
Before we begin, it's crucial to understand the structure of your "party_matchups.txt" file. This will dictate the best approach for importing the data. Let's assume a few potential structures:
Scenario 1: Comma-Separated Values (CSV)
This is the simplest scenario. Each line represents a matchup, with values separated by commas. Example:
Republican,Democrat,2020
Republican,Independent,2016
Democrat,Green,2022
Scenario 2: Tab-Separated Values (TSV)
Similar to CSV, but values are separated by tabs. This is often used to handle commas within data fields. Example:
Republican Democrat 2020
Republican Independent 2016
Democrat Green 2022
Scenario 3: Space-Separated Values (SSV)
Values are separated by spaces. More prone to errors if data fields contain spaces. Example:
Republican Democrat 2020
Republican Independent 2016
Democrat Green 2022
Scenario 4: Fixed-Width Format
Each field occupies a specific number of characters. Requires precise knowledge of the field widths. Example: (Assuming 15 characters per field)
Republican Democrat 2020
Republican Independent 2016
Democrat Green 2022
Scenario 5: Irregular Format
The data might have inconsistent separators or no clear delimiter. This requires more advanced techniques, potentially involving regular expressions. Example:
Republican vs Democrat (2020)
Republican vs. Independent (2016)
Democrat and Green in 2022
Importing the Data: Different Approaches
The method you choose depends heavily on the structure of your "party_matchups.txt" file and your preferred tools.
Method 1: Python with the csv
module (for CSV and TSV)
Python's built-in csv
module is ideal for importing CSV and TSV files. This approach is simple and efficient.
import csv
def import_csv(filepath):
"""Imports a CSV or TSV file into a list of lists."""
data = []
with open(filepath, 'r', newline='') as file:
reader = csv.reader(file, delimiter=',') # Change delimiter to '\t' for TSV
for row in reader:
data.append(row)
return data
# Example usage (for CSV):
filepath = "party_matchups.txt"
party_matchups = import_csv(filepath)
print(party_matchups)
# Example usage (for TSV):
filepath = "party_matchups.txt"
party_matchups = import_csv(filepath) #Change delimiter to '\t' within the function
print(party_matchups)
This code reads the file line by line, creating a list of lists, where each inner list represents a row from the file. Remember to change the delimiter
argument in csv.reader()
to '\t'
for tab-separated files.
Method 2: Python with pandas (for various formats)
Pandas is a powerful Python library for data manipulation and analysis. It can handle various file formats and offers many data processing features.
import pandas as pd
def import_with_pandas(filepath, delimiter=',', header=None):
"""Imports a file into a pandas DataFrame."""
try:
df = pd.read_csv(filepath, delimiter=delimiter, header=header)
return df
except pd.errors.EmptyDataError:
print("Error: File is empty.")
return None
except pd.errors.ParserError:
print("Error: Could not parse the file. Check the delimiter and file format.")
return None
# Example usage (for CSV):
filepath = "party_matchups.txt"
df = import_with_pandas(filepath)
print(df)
# Example usage (for TSV):
filepath = "party_matchups.txt"
df = import_with_pandas(filepath, delimiter='\t')
print(df)
#Example usage for space separated (adjust based on your needs)
filepath = "party_matchups.txt"
df = import_with_pandas(filepath, delimiter=' ')
print(df)
Pandas automatically infers the delimiter in many cases, but you can specify it using the delimiter
argument. The header
argument specifies the row number (starting from 0) to be used as the header. Set it to None
if there's no header row. Error handling is included to gracefully manage empty or improperly formatted files.
Method 3: SQL (for structured data)
If your data is well-structured, you can import it into a SQL database using the COPY
command (PostgreSQL) or equivalent commands in other database systems. This approach is best for large datasets and allows for efficient querying and analysis using SQL.
(PostgreSQL Example)
First, create a table to store the data:
CREATE TABLE party_matchups (
party1 TEXT,
party2 TEXT,
year INTEGER
);
Then, import the data using the COPY
command:
COPY party_matchups FROM '/path/to/party_matchups.txt' DELIMITER ',' CSV HEADER;
Replace /path/to/party_matchups.txt
with the actual path to your file. Adjust the DELIMITER
and HEADER
options as needed based on your file's format.
Method 4: Handling Irregular Formats (Python with Regular Expressions)
For irregularly formatted files, you might need to use regular expressions to extract the relevant information. This is a more complex approach, requiring careful crafting of regular expressions to match the patterns in your data.
import re
def import_irregular(filepath, pattern):
"""Imports data from an irregularly formatted file using regular expressions."""
data = []
with open(filepath, 'r') as file:
for line in file:
match = re.search(pattern, line)
if match:
data.append(match.groups())
return data
#Example - needs adjustment based on your specific irregular format.
filepath = "party_matchups.txt"
pattern = r"(\w+) vs (\w+) \((\d+)\)" #Example pattern: Party1 vs Party2 (Year)
party_matchups = import_irregular(filepath, pattern)
print(party_matchups)
This example uses a simple regular expression. You'll need to adjust the pattern
variable to precisely match the structure of your irregular data. This often involves experimentation and iterative refinement.
Data Cleaning and Validation
Once you've imported the data, it's crucial to clean and validate it. This involves:
- Handling missing values: Decide how to handle rows with missing data (e.g., imputation, removal).
- Data type conversion: Convert columns to appropriate data types (e.g., string to integer for the year).
- Data validation: Check for inconsistencies, errors, or outliers in the data.
- Duplicate removal: Identify and remove duplicate rows.
Choosing the Right Approach
The best approach depends on your specific needs and the characteristics of your data:
- Simple CSV/TSV: Python's
csv
module or pandas are sufficient. - Complex or Large Datasets: pandas or SQL are more suitable for their efficiency and features.
- Irregular Formats: Requires regular expressions and potentially more sophisticated parsing techniques.
Remember to always back up your original data before performing any transformations. This allows you to revert to the original data if needed. By following these steps and selecting the appropriate tools, you can effectively import your "party_matchups.txt" file and prepare it for analysis and further processing. The ability to handle various data formats and complexities is essential for successful data analysis. Remember to always consider error handling and data validation steps to ensure data quality and robustness in your analyses.
Latest Posts
Latest Posts
-
How Long Is 90 Minutes In Hours
May 20, 2025
-
How Many Hours In 28 Days
May 20, 2025
-
What Is 13 5 Stone In Pounds
May 20, 2025
-
What Is 8 5 Stone In Kg
May 20, 2025
-
What Is 77 Kg In Stones And Pounds
May 20, 2025
Related Post
Thank you for visiting our website which covers about Import The Text File Paty Matchups Txt As A Table . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.