Function Definition Volume Of A Pyramid
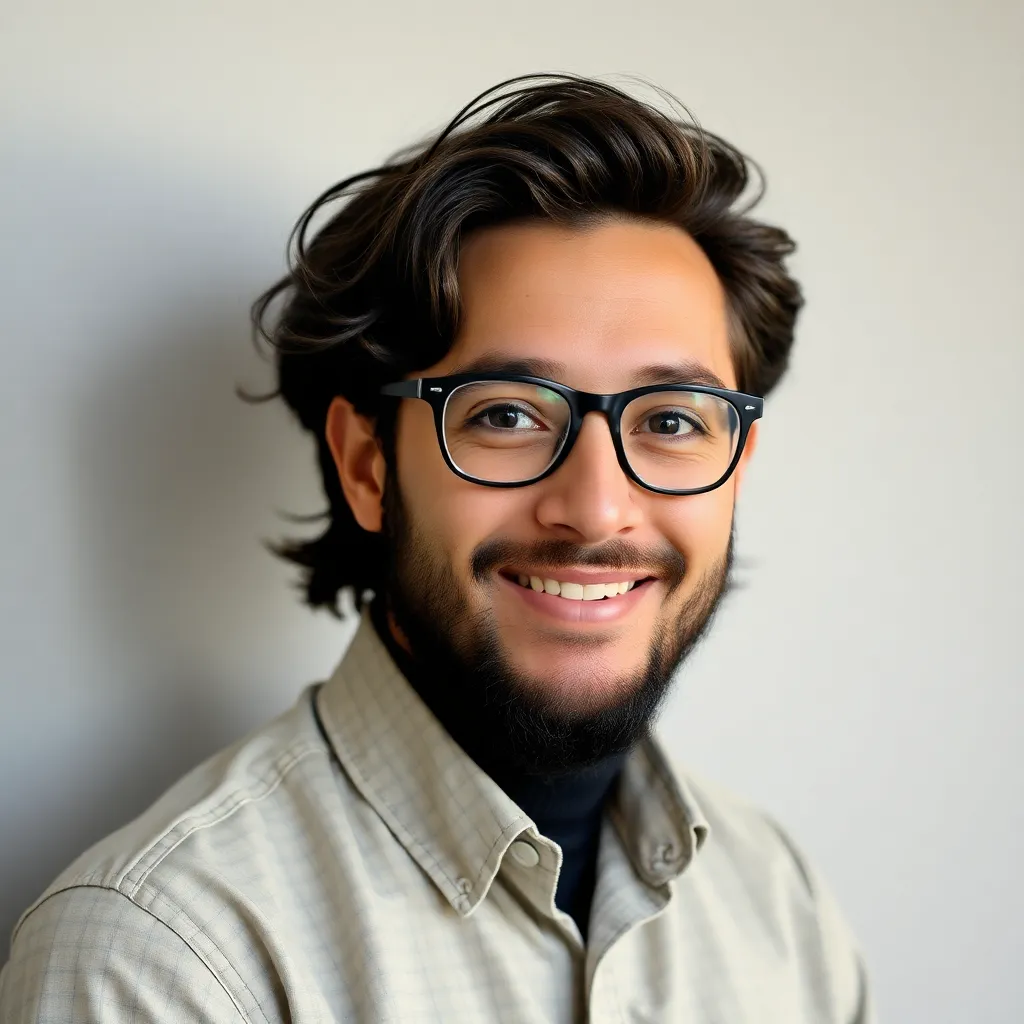
Holbox
Mar 14, 2025 · 6 min read

Table of Contents
- Function Definition Volume Of A Pyramid
- Table of Contents
- Function Definition: Volume of a Pyramid
- Understanding the Basics: What is a Pyramid?
- The Formula: Defining the Volume Function
- Function Definition in Programming Languages
- Calculating Base Area for Different Pyramid Types
- Applications and Practical Uses
- Handling Complexities and Challenges
- Advanced Considerations: Numerical Methods and Optimization
- Conclusion
- Latest Posts
- Related Post
Function Definition: Volume of a Pyramid
Understanding how to calculate the volume of a pyramid is a fundamental concept in geometry with applications across various fields, from architecture and engineering to computer graphics and surveying. This article delves into the function definition for calculating the volume of a pyramid, exploring different approaches, handling various pyramid types, and considering practical applications and potential challenges.
Understanding the Basics: What is a Pyramid?
A pyramid is a three-dimensional geometric shape with a polygonal base and triangular faces that meet at a common point called the apex or vertex. The type of pyramid is determined by the shape of its base. Common types include:
- Triangular Pyramid (Tetrahedron): A pyramid with a triangular base. This is the simplest form.
- Square Pyramid: A pyramid with a square base.
- Rectangular Pyramid: A pyramid with a rectangular base.
- Pentagonal Pyramid: A pyramid with a pentagonal base.
- And so on... The possibilities extend to any polygon as a base.
The height of a pyramid is the perpendicular distance from the apex to the base. This is a crucial measurement for volume calculations. The base area is simply the area of the polygon forming the base.
The Formula: Defining the Volume Function
The formula for the volume (V) of any pyramid is:
V = (1/3) * B * h
Where:
- V represents the volume of the pyramid.
- B represents the area of the base.
- h represents the perpendicular height of the pyramid.
This formula is a fundamental principle in geometry. Its derivation involves complex calculus but the formula itself is remarkably simple to apply. Let's break down the implications:
- Linear Relationship with Height: The volume is directly proportional to the height. Doubling the height doubles the volume, tripling it triples the volume, and so on.
- Dependence on Base Area: The volume is directly proportional to the area of the base. A larger base results in a larger volume.
Function Definition in Programming Languages
The formula can be easily translated into a function in various programming languages. Here are examples in Python and JavaScript:
Python:
def pyramid_volume(base_area, height):
"""Calculates the volume of a pyramid.
Args:
base_area: The area of the pyramid's base.
height: The perpendicular height of the pyramid.
Returns:
The volume of the pyramid. Returns an error message if input is invalid.
"""
if base_area <= 0 or height <= 0:
return "Error: Base area and height must be positive values."
volume = (1/3) * base_area * height
return volume
# Example usage
base = 16 # Area of a 4x4 square base
h = 10
vol = pyramid_volume(base, h)
print(f"The volume of the pyramid is: {vol}")
#Example with a triangular base
base_triangle = 0.5 * 5 * 4 #Area of a triangle with base 5 and height 4
h_triangle = 6
vol_triangle = pyramid_volume(base_triangle, h_triangle)
print(f"The volume of the triangular pyramid is: {vol_triangle}")
JavaScript:
function pyramidVolume(baseArea, height) {
// Input validation
if (baseArea <= 0 || height <= 0) {
return "Error: Base area and height must be positive values.";
}
const volume = (1/3) * baseArea * height;
return volume;
}
// Example usage
const base = 16; // Area of a 4x4 square base
const h = 10;
const vol = pyramidVolume(base, h);
console.log(`The volume of the pyramid is: ${vol}`);
//Example with a triangular base
const base_triangle = 0.5 * 5 * 4; //Area of a triangle with base 5 and height 4
const h_triangle = 6;
const vol_triangle = pyramidVolume(base_triangle, h_triangle);
console.log(`The volume of the triangular pyramid is: ${vol_triangle}`);
These functions take the base area and height as input and return the calculated volume. Crucially, they include error handling to prevent issues with invalid input (negative or zero values).
Calculating Base Area for Different Pyramid Types
The complexity in calculating the volume often lies in determining the base area (B). Here's a breakdown for common pyramid types:
- Square Pyramid: B = s², where 's' is the side length of the square base.
- Rectangular Pyramid: B = l * w, where 'l' is the length and 'w' is the width of the rectangular base.
- Triangular Pyramid: B = (1/2) * b * h_b, where 'b' is the base of the triangular base and 'h_b' is the height of the triangular base.
- Pentagonal Pyramid (and beyond): For more complex polygons, you'll need to use appropriate formulas or methods to calculate the area. Trigonometry and/or breaking the polygon into smaller triangles might be necessary.
These base area calculations can be incorporated into more sophisticated pyramid volume functions. For example, a function for a square pyramid could look like this (Python):
def square_pyramid_volume(side_length, height):
"""Calculates the volume of a square pyramid.
Args:
side_length: The length of a side of the square base.
height: The perpendicular height of the pyramid.
Returns:
The volume of the square pyramid. Returns an error message if input is invalid.
"""
if side_length <=0 or height <= 0:
return "Error: Side length and height must be positive values."
base_area = side_length**2
volume = (1/3) * base_area * height
return volume
Applications and Practical Uses
The ability to calculate pyramid volume has many practical applications:
- Construction and Architecture: Estimating the amount of material needed for building pyramids (or pyramid-shaped structures).
- Civil Engineering: Calculating the volume of earth to be moved during excavation projects involving pyramid-shaped landforms.
- Mining: Determining the volume of ore in a conical or pyramid-shaped deposit.
- Computer Graphics: Rendering realistic 3D models requires accurate volume calculations for shading and other visual effects.
- Environmental Science: Measuring the volume of sediment deposits in a particular area.
Handling Complexities and Challenges
While the basic formula is straightforward, certain situations might present challenges:
- Irregular Bases: Calculating the area of irregularly shaped bases can be complex and might require numerical integration techniques.
- Oblique Pyramids: In oblique pyramids, the apex is not directly above the center of the base. The height calculation becomes more involved, potentially requiring trigonometry.
- Truncated Pyramids (Frustums): These are pyramids with their tops cut off. A separate formula is needed for calculating their volume.
Advanced Considerations: Numerical Methods and Optimization
For irregularly shaped bases, numerical integration techniques like Simpson's rule or the trapezoidal rule can be used to approximate the base area with sufficient accuracy. This can then be fed into the volume calculation function.
Optimization techniques can be applied to minimize computation time, especially when dealing with large datasets or highly complex shapes.
Conclusion
The function definition for calculating the volume of a pyramid, while seemingly simple at its core, encapsulates a powerful geometric principle with broad applications. Understanding the formula and its implications, combined with the ability to programmatically implement it and handle potential complexities, equips individuals with a valuable tool for various mathematical and practical problem-solving scenarios. Remember to always validate your inputs to ensure accurate and reliable results. The combination of sound mathematical understanding and effective programming provides a robust approach to tackling geometric calculations.
Latest Posts
Related Post
Thank you for visiting our website which covers about Function Definition Volume Of A Pyramid . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.