Final Assignment Part 2 Create Dashboard With Plotly And Dash
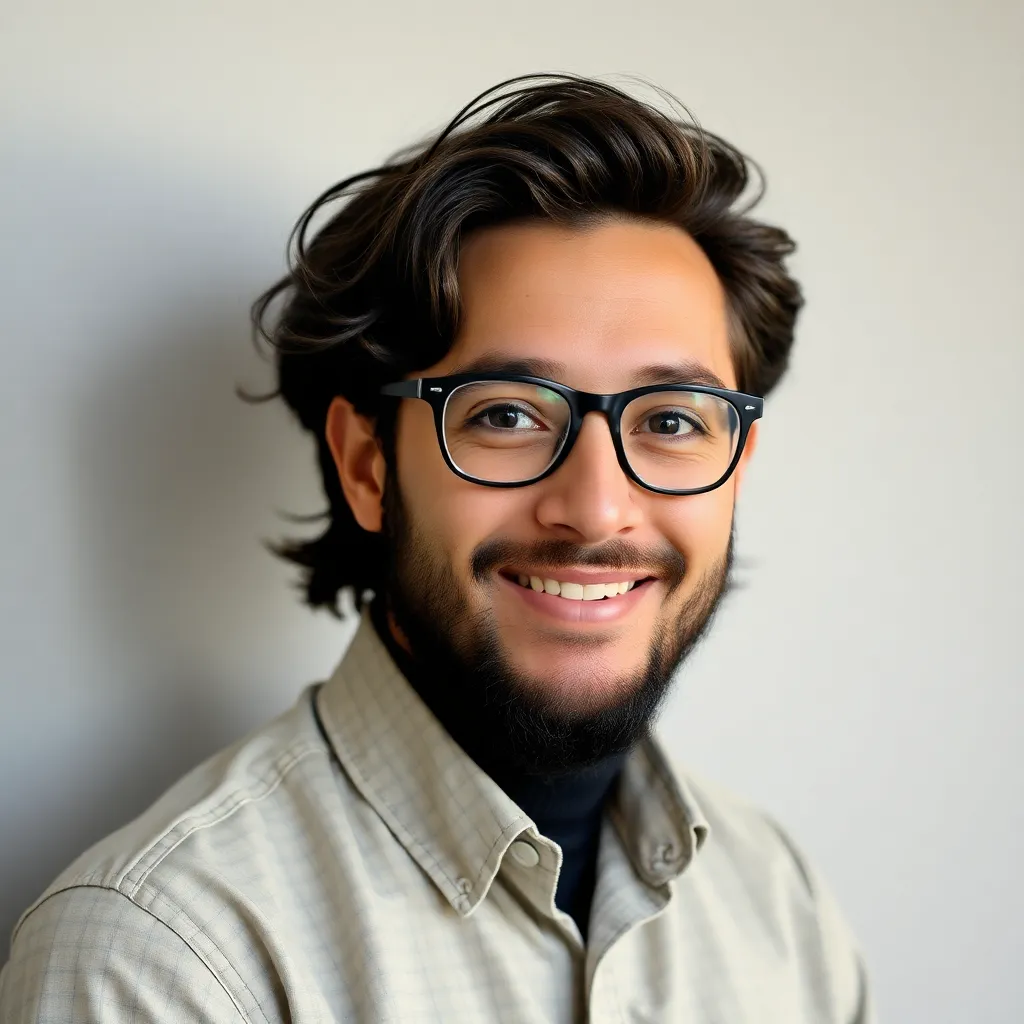
Holbox
May 09, 2025 · 6 min read
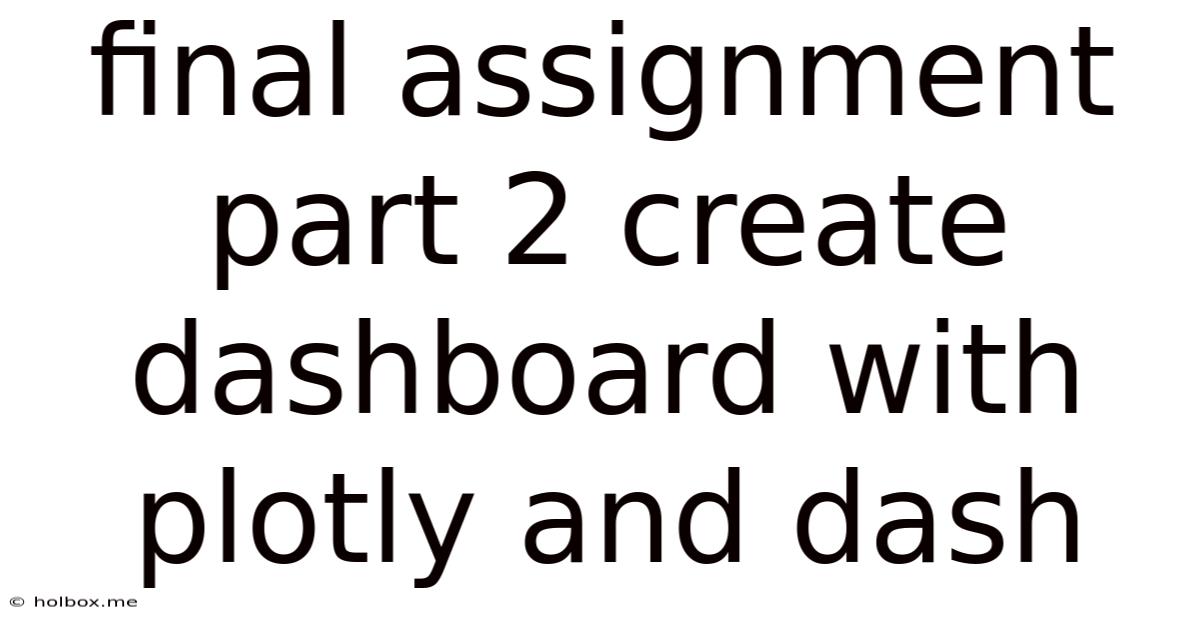
Table of Contents
- Final Assignment Part 2 Create Dashboard With Plotly And Dash
- Table of Contents
- Final Assignment Part 2: Creating a Dashboard with Plotly and Dash
- Setting Up Your Environment
- Data Acquisition and Preparation
- Building the Dashboard with Dash
- Defining the App Layout
- Creating Interactive Components
- Enhancing the Dashboard with Advanced Features
- Multiple Charts and Layouts
- Interactive Controls: Sliders and Range Sliders
- Custom Styling with CSS
- Data Tables
- Deployment and Sharing
- SEO Optimization for Your Dashboard Blog Post
- Latest Posts
- Related Post
Final Assignment Part 2: Creating a Dashboard with Plotly and Dash
This comprehensive guide will walk you through the process of creating a compelling data dashboard using Plotly and Dash for your final assignment. We'll cover everything from setting up your environment to deploying a functional and visually appealing dashboard, focusing on best practices for both functionality and aesthetics. This detailed walkthrough assumes a basic understanding of Python and data manipulation libraries like Pandas.
Setting Up Your Environment
Before diving into the dashboard creation, ensure you have the necessary libraries installed. Use pip, the package installer for Python, to install the required packages:
pip install plotly dash pandas numpy
This command installs Plotly for interactive visualizations, Dash for building the dashboard framework, Pandas for data manipulation, and NumPy for numerical computing. Confirm installations by importing them in a Python script:
import plotly
import dash
import pandas as pd
import numpy as np
print(plotly.__version__)
print(dash.__version__)
print(pd.__version__)
print(np.__version__)
This verifies the successful installation and displays the versions of each package.
Data Acquisition and Preparation
The foundation of any effective dashboard is clean and well-structured data. For this tutorial, we'll assume you're working with a CSV file containing your data. Replace "your_data.csv"
with the actual path to your file.
import pandas as pd
data = pd.read_csv("your_data.csv")
# Data Cleaning and Preprocessing
# ... Add your data cleaning and preprocessing steps here ...
# This might include handling missing values, data type conversions, etc.
print(data.head()) # Inspect the first few rows of your cleaned data
Remember to adapt the data cleaning and preprocessing steps (indicated by # ...
) to suit your specific dataset. This crucial step ensures your dashboard displays accurate and meaningful information. Common preprocessing steps include:
- Handling Missing Values: Imputation (filling missing values) or removal of rows/columns with excessive missing data.
- Data Type Conversion: Ensuring your data is in the correct format (e.g., converting strings to dates or numbers).
- Data Transformation: Applying transformations like logarithmic scaling or standardization to improve visualization.
- Feature Engineering: Creating new features from existing ones to enhance insights.
Building the Dashboard with Dash
Dash provides a framework for creating interactive web applications. We'll build our dashboard using a series of callbacks and layouts.
Defining the App Layout
The app layout defines the structure of your dashboard. We use dash.html
components to arrange different elements, and dcc
(dash core components) for interactive elements like graphs and dropdowns.
from dash import Dash, html, dcc
import plotly.express as px
app = Dash(__name__)
app.layout = html.Div(children=[
html.H1(children='My Data Dashboard'),
html.Div(children='''
Dash: A web application framework for your data.
'''),
dcc.Dropdown(
id='dropdown-selector',
options=[{'label': i, 'value': i} for i in data['column_name'].unique()], #Replace 'column_name'
value=data['column_name'].unique()[0] #Replace 'column_name'
),
dcc.Graph(id='interactive-graph'),
])
This code creates a simple layout with a title, description, a dropdown for selecting data subsets, and a graph component to display the visualizations. Remember to replace "column_name"
with the actual name of the column in your DataFrame you want to use for the dropdown.
Creating Interactive Components
Now, we'll build the interactivity using callbacks. Callbacks connect the input component (dropdown) to the output component (graph), updating the graph based on the user's selection.
import plotly.express as px
from dash.dependencies import Input, Output
@app.callback(
Output('interactive-graph', 'figure'),
Input('dropdown-selector', 'value')
)
def update_graph(selected_value):
filtered_data = data[data['column_name'] == selected_value] #Replace 'column_name'
fig = px.scatter(filtered_data, x="x_column", y="y_column", color="color_column") #Replace x_column, y_column, color_column
return fig
if __name__ == '__main__':
app.run_server(debug=True)
This callback function update_graph
takes the selected value from the dropdown as input and filters the data accordingly. It then generates a Plotly scatter plot (px.scatter
) using the filtered data. You need to replace "x_column"
, "y_column"
, and "color_column"
with the appropriate column names from your DataFrame. The app.run_server(debug=True)
starts the development server.
Enhancing the Dashboard with Advanced Features
To make your dashboard even more effective, incorporate the following advanced features:
Multiple Charts and Layouts
Instead of a single graph, include multiple charts to present different aspects of your data. Use html.Div
and html.Br
to organize the charts effectively.
app.layout = html.Div([
# ... (previous code) ...
html.Br(), #Line Break
dcc.Graph(id='graph-2'), #Second Graph
# ... Add more graphs and layouts as needed ...
])
# ... Add corresponding callback functions for the new graphs ...
Interactive Controls: Sliders and Range Sliders
Enhance interactivity with sliders and range sliders to allow users to filter data based on numerical ranges.
dcc.Slider(
id='year-slider',
min=data['year'].min(),
max=data['year'].max(),
value=data['year'].max(),
marks={str(year): str(year) for year in data['year'].unique()},
step=None
),
Remember to integrate this slider into your callbacks to update the charts dynamically.
Custom Styling with CSS
Use CSS to customize the appearance of your dashboard. You can embed CSS directly in your layout or link to an external stylesheet.
.graph-container {
width: 80%;
margin: 0 auto;
}
This example sets the width and margin of elements with class "graph-container."
Data Tables
Include interactive data tables using dash_table
to allow users to explore the raw data. Install dash-table
using pip install dash-table
.
from dash_table import DataTable
DataTable(
data=data.to_dict('records'),
columns=[{'id': c, 'name': c} for c in data.columns],
page_size=10,
style_table={'overflowX': 'auto'}
)
Deployment and Sharing
Once your dashboard is complete, you can deploy it using various platforms like:
- Local Deployment: Run the
app.run_server()
command to host it locally. Share the local URL with others. - Cloud Platforms: Deploy to platforms like Heroku, AWS, or Google Cloud for wider accessibility. These platforms typically require configuration files and may have associated costs.
- Plotly Chart Studio: This is a cloud-based platform designed for sharing and deploying Plotly dashboards.
Remember to thoroughly test your dashboard before sharing to ensure accuracy and functionality.
SEO Optimization for Your Dashboard Blog Post
To maximize the visibility of your blog post about creating dashboards with Plotly and Dash, apply the following SEO strategies:
- Keyword Research: Identify relevant keywords like "Plotly Dash dashboard tutorial," "Python data visualization dashboard," "interactive data dashboard," etc.
- Title Tag and Meta Description: Optimize your title and meta description to include relevant keywords and a compelling description of your blog post.
- Header Tags (H1, H2, H3): Use header tags to structure your content logically and incorporate keywords naturally.
- Image Optimization: Use relevant images and optimize them with alt text containing keywords.
- Internal and External Linking: Link to other relevant blog posts on your website and authoritative sources on data visualization and web development.
- URL Structure: Use a clear and concise URL that includes relevant keywords.
- Schema Markup: Implement schema markup to provide search engines with structured data about your content.
- Social Media Promotion: Share your blog post on social media platforms to increase its visibility.
By meticulously following these steps, you can build a highly functional and visually appealing data dashboard, and effectively communicate your project's value through a well-optimized blog post. Remember, continuous learning and iteration are key to mastering data visualization and dashboard creation.
Latest Posts
Related Post
Thank you for visiting our website which covers about Final Assignment Part 2 Create Dashboard With Plotly And Dash . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.