Convert The Following Expression To The Indicated Base.
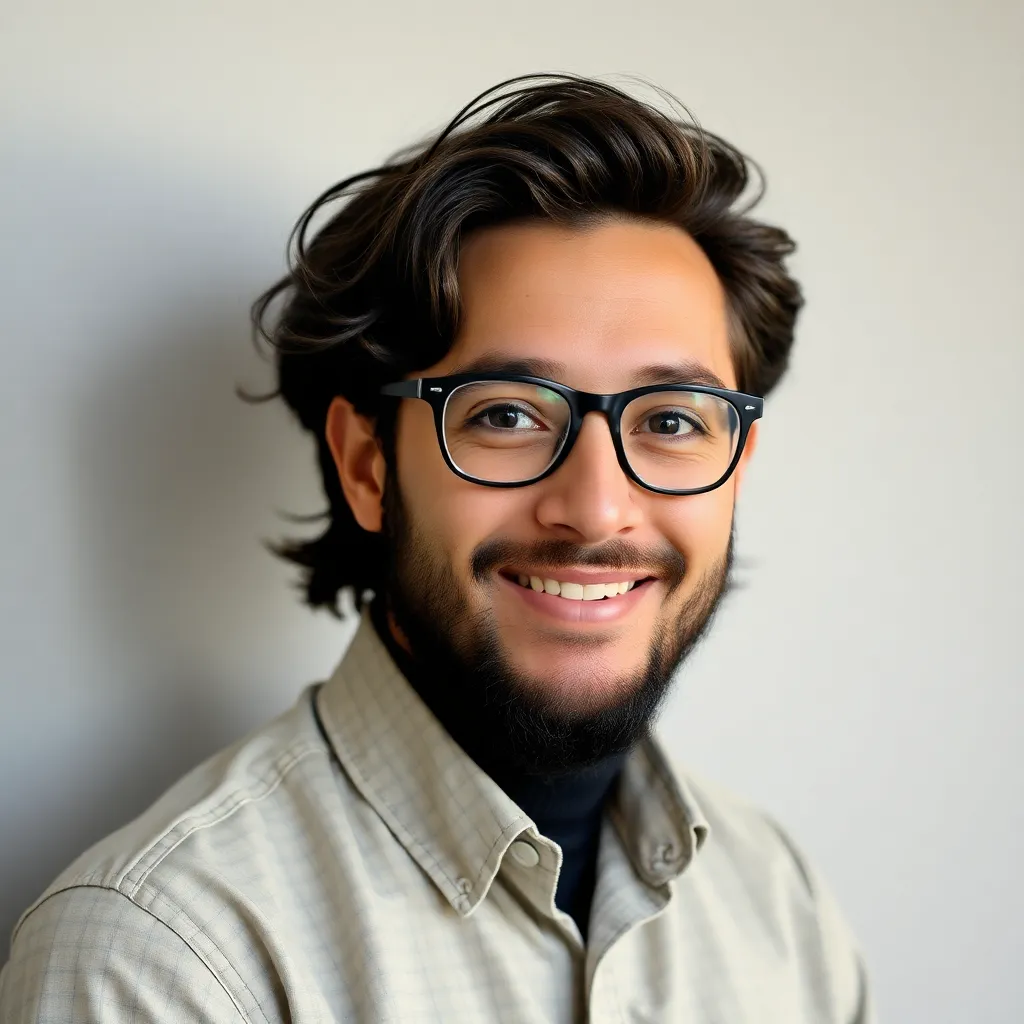
Holbox
May 07, 2025 · 6 min read
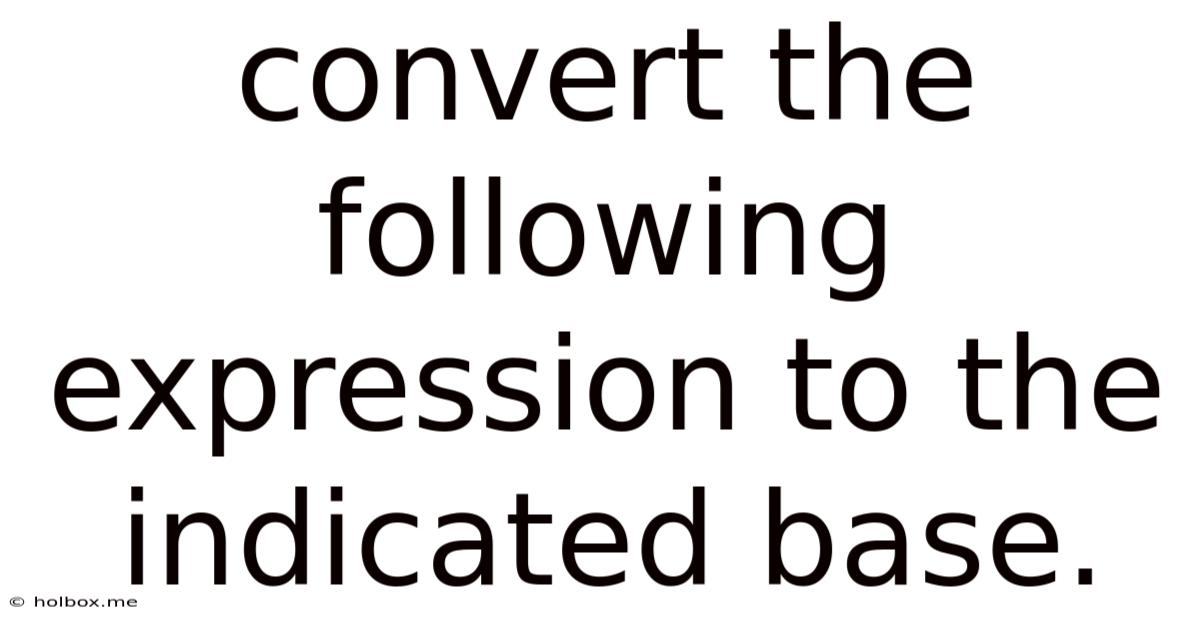
Table of Contents
- Convert The Following Expression To The Indicated Base.
- Table of Contents
- Converting Expressions to Different Bases: A Comprehensive Guide
- Understanding Number Bases
- Converting from Decimal to Other Bases
- Algorithm for Decimal to Base-b Conversion:
- Converting from Other Bases to Decimal
- Algorithm for Base-b to Decimal Conversion:
- Converting Between Non-Decimal Bases
- Efficient Conversion Techniques and Tools
- Applications of Base Conversion
- Handling Expressions with Multiple Bases
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
Converting Expressions to Different Bases: A Comprehensive Guide
Converting numerical expressions from one base to another is a fundamental concept in computer science, mathematics, and various other fields. Understanding this process is crucial for tasks ranging from representing data in different formats to performing arithmetic operations in non-decimal systems. This comprehensive guide will explore the techniques and principles involved in converting expressions to different bases, focusing on clarity and practical application. We'll delve into both manual conversion methods and how to leverage tools for efficient conversion.
Understanding Number Bases
Before we dive into the conversion process, let's review the concept of number bases. The base, or radix, of a number system specifies the number of unique digits used to represent numbers.
- Base 10 (Decimal): The most common number system we use daily. It uses ten digits (0-9).
- Base 2 (Binary): Used extensively in computer science, it employs only two digits (0 and 1).
- Base 8 (Octal): Uses eight digits (0-7).
- Base 16 (Hexadecimal): Uses sixteen digits (0-9 and A-F, where A=10, B=11, C=12, D=13, E=14, F=15).
Each digit in a number represents a power of the base. For example, in the decimal number 123, the 3 represents 3 x 10⁰, the 2 represents 2 x 10¹, and the 1 represents 1 x 10².
Converting from Decimal to Other Bases
Converting a decimal number to another base involves repeatedly dividing the decimal number by the target base and recording the remainders. The remainders, read in reverse order, form the representation in the new base.
Algorithm for Decimal to Base-b Conversion:
- Divide: Divide the decimal number by the target base (b).
- Record Remainder: Note the remainder.
- Repeat: Repeat steps 1 and 2 with the quotient obtained in step 1, until the quotient becomes 0.
- Reverse: The remainders, read from bottom to top (last remainder to first remainder), represent the number in the target base.
Let's illustrate this with examples:
Example 1: Converting 25 (decimal) to binary (base 2)
Division | Quotient | Remainder |
---|---|---|
25 ÷ 2 | 12 | 1 |
12 ÷ 2 | 6 | 0 |
6 ÷ 2 | 3 | 0 |
3 ÷ 2 | 1 | 1 |
1 ÷ 2 | 0 | 1 |
Reading the remainders from bottom to top, we get 11001₂. Therefore, 25₁₀ = 11001₂.
Example 2: Converting 175 (decimal) to hexadecimal (base 16)
Division | Quotient | Remainder |
---|---|---|
175 ÷ 16 | 10 | 15 (F) |
10 ÷ 16 | 0 | 10 (A) |
Reading the remainders from bottom to top, we get AF₁₆. Therefore, 175₁₀ = AF₁₆.
Converting from Other Bases to Decimal
Converting a number from any base to decimal involves multiplying each digit by the corresponding power of the base and summing the results.
Algorithm for Base-b to Decimal Conversion:
- Identify Digits: Identify the digits and their positions (from right to left, starting with position 0).
- Multiply and Sum: Multiply each digit by the corresponding power of the base (b). For example, the rightmost digit is multiplied by b⁰, the next digit by b¹, the next by b², and so on.
- Add: Sum the results of the multiplications.
Example 1: Converting 110101₂ (binary) to decimal
1 x 2⁵ + 1 x 2⁴ + 0 x 2³ + 1 x 2² + 0 x 2¹ + 1 x 2⁰ = 32 + 16 + 0 + 4 + 0 + 1 = 53₁₀
Therefore, 110101₂ = 53₁₀.
Example 2: Converting 2B5₁₆ (hexadecimal) to decimal
2 x 16² + 11 (B) x 16¹ + 5 x 16⁰ = 512 + 176 + 5 = 693₁₀
Therefore, 2B5₁₆ = 693₁₀.
Converting Between Non-Decimal Bases
Converting between non-decimal bases (e.g., binary to hexadecimal, octal to binary) can be done in two steps:
- Convert to Decimal: First, convert the number from the source base to decimal using the method described above.
- Convert from Decimal: Then, convert the decimal equivalent to the target base using the method described earlier.
Example: Converting 111010₂ (binary) to hexadecimal (base 16)
- Binary to Decimal: 1 x 2⁵ + 1 x 2⁴ + 1 x 2³ + 0 x 2² + 1 x 2¹ + 0 x 2⁰ = 32 + 16 + 8 + 0 + 2 + 0 = 58₁₀
- Decimal to Hexadecimal:
- 58 ÷ 16 = 3 remainder 10 (A)
- 3 ÷ 16 = 0 remainder 3
Therefore, 111010₂ = 3A₁₆.
Efficient Conversion Techniques and Tools
While manual conversion is valuable for understanding the underlying principles, using tools can significantly speed up the process, especially for larger numbers. Many programming languages and online calculators offer built-in functions or tools for base conversion.
Programming Languages:
Most programming languages (Python, Java, C++, JavaScript, etc.) provide functions for base conversion. For example, in Python:
# Convert decimal to binary
decimal_num = 25
binary_num = bin(decimal_num) # Output: 0b11001
print(binary_num[2:]) # Remove the "0b" prefix
# Convert binary to decimal
binary_str = "11001"
decimal_num = int(binary_str, 2) # Output: 25
print(decimal_num)
# Convert to hexadecimal
hex_num = hex(decimal_num) # Output: 0x19
print(hex_num[2:]) # Remove the "0x" prefix
These functions significantly simplify the conversion process, especially when dealing with complex expressions or large numbers.
Applications of Base Conversion
The ability to convert between different number bases has wide-ranging applications:
- Computer Science: Binary, octal, and hexadecimal are fundamental in computer science for representing data in memory, processing instructions, and debugging code.
- Digital Signal Processing: Base conversion is essential in representing and manipulating digital signals.
- Cryptography: Certain cryptographic algorithms rely on operations in different number bases.
- Error Detection and Correction: Base conversion aids in developing error detection and correction codes.
- Mathematics: Base conversion provides a different perspective on number systems and their properties.
Handling Expressions with Multiple Bases
When dealing with expressions that involve numbers in different bases, always ensure you convert all components to a common base (usually decimal) before performing any arithmetic operations. After calculations, you can convert the result back to your desired base.
Example: Add 1101₂ and 2A₁₆
-
Convert to Decimal:
- 1101₂ = 13₁₀
- 2A₁₆ = 42₁₀
-
Perform Addition: 13₁₀ + 42₁₀ = 55₁₀
-
Convert back to desired base (e.g., hexadecimal):
- 55₁₀ = 37₁₆
Conclusion
Converting expressions between different number bases is a vital skill in various domains. Understanding the underlying algorithms and utilizing available tools can make this process efficient and straightforward. This comprehensive guide has covered the key techniques for performing these conversions, providing both manual methods and programming examples for practical application. Remember to always consider the context and choose the most appropriate base for your specific needs. Mastering base conversion opens up a deeper understanding of numerical systems and their practical applications in computing and beyond.
Latest Posts
Latest Posts
-
How Tall Is 186 Cm In Feet
May 19, 2025
-
How Many Seconds In 2 Minutes
May 19, 2025
-
How Many Oz Are In 600 Ml
May 19, 2025
-
How Many Days In 13 Weeks
May 19, 2025
-
195 Cm In Feet And Inches
May 19, 2025
Related Post
Thank you for visiting our website which covers about Convert The Following Expression To The Indicated Base. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.