Complete The Function Definition To Return The Hours Given Minutes.
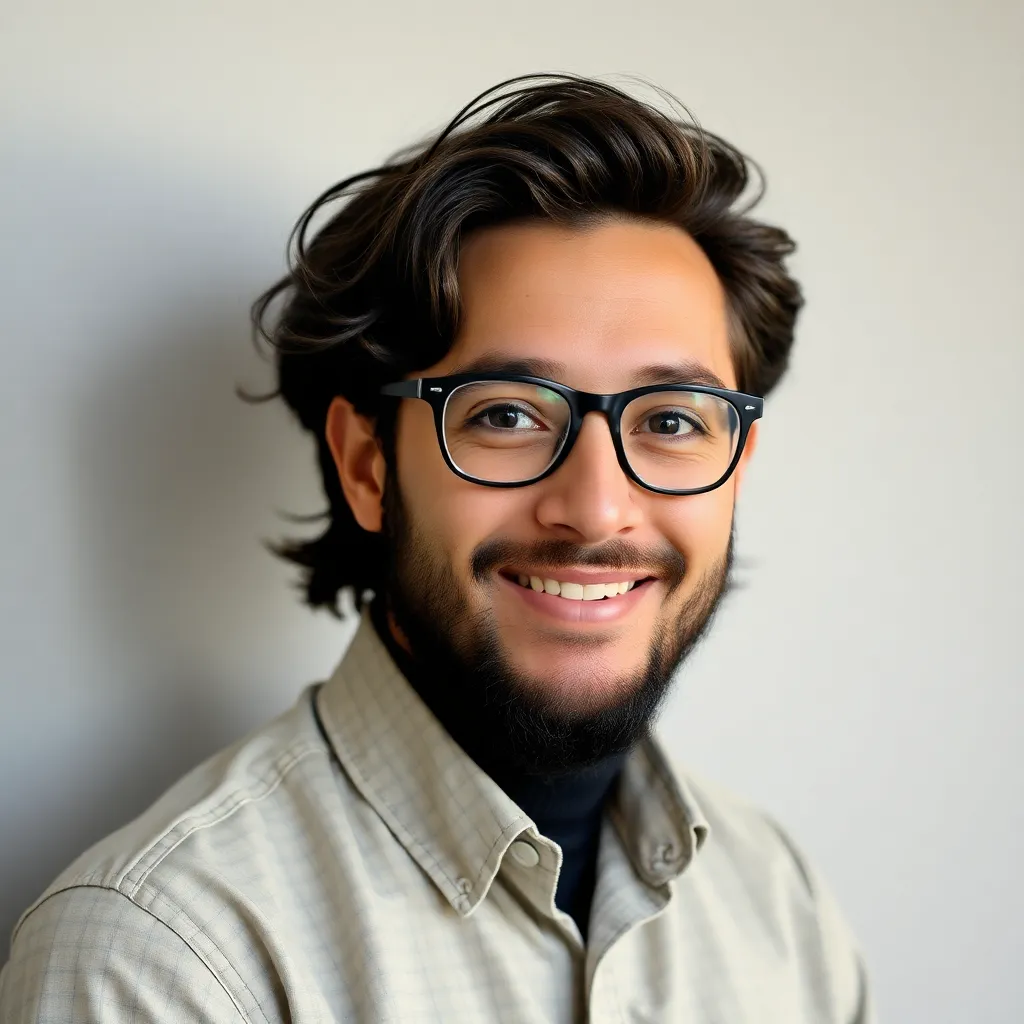
Holbox
May 11, 2025 · 5 min read
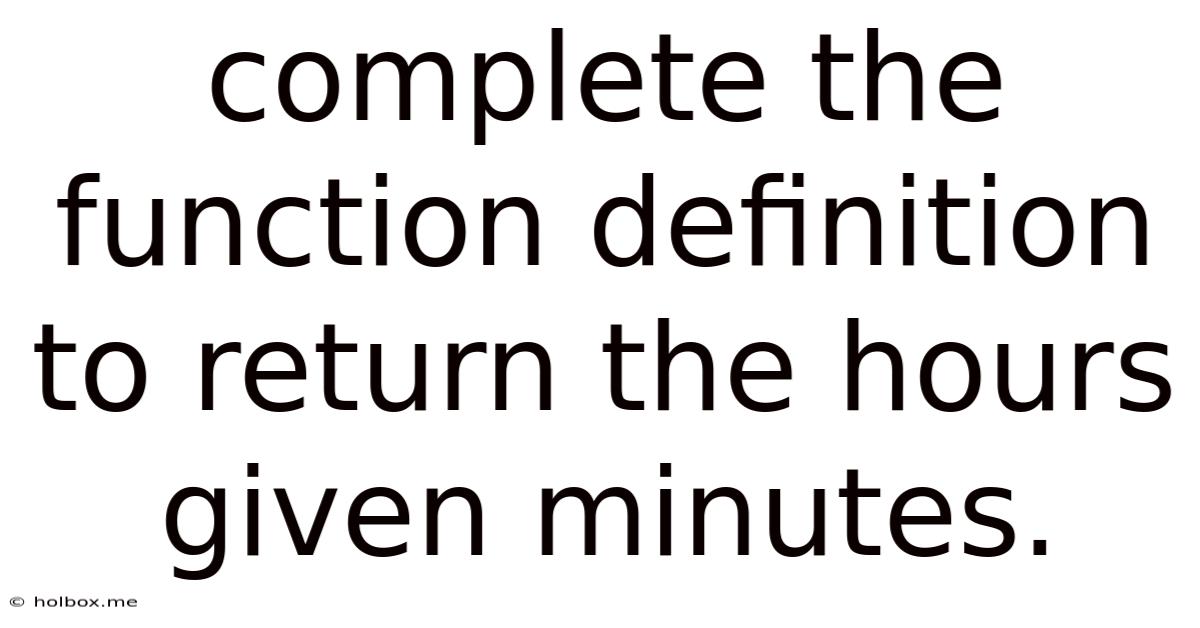
Table of Contents
- Complete The Function Definition To Return The Hours Given Minutes.
- Table of Contents
- Complete the Function Definition to Return the Hours Given Minutes
- Understanding the Core Conversion Logic
- Implementing the Function in Different Programming Languages
- Python
- JavaScript
- Java
- C#
- C++
- Handling Edge Cases and Advanced Scenarios
- Handling Non-Integer Inputs
- Rounding and Precision
- Error Handling and Input Validation
- Extending Functionality
- Best Practices for Function Design
- Conclusion
- Latest Posts
- Related Post
Complete the Function Definition to Return the Hours Given Minutes
This comprehensive guide delves into the intricacies of converting minutes into hours, focusing on crafting robust and efficient function definitions in various programming languages. We'll explore different approaches, address potential challenges, and emphasize best practices for creating reusable and scalable code. The core problem revolves around understanding the fundamental relationship between minutes and hours – there are 60 minutes in one hour. This seemingly simple conversion, however, offers a fertile ground for exploring different programming paradigms and refining coding skills.
Understanding the Core Conversion Logic
At the heart of this problem lies a simple mathematical formula:
Hours = Minutes / 60
This formula provides the basic framework for our function. However, depending on the desired level of precision and handling of edge cases (like non-integer minute values), the implementation can become more complex.
Implementing the Function in Different Programming Languages
Let's explore how to implement this conversion function in several popular programming languages, highlighting best practices and variations:
Python
def minutes_to_hours(minutes):
"""Converts minutes to hours.
Args:
minutes: The number of minutes to convert. Must be a non-negative number.
Returns:
The equivalent number of hours as a float. Returns -1 if input is invalid.
"""
if minutes < 0:
return -1 # Handle negative input gracefully
return minutes / 60
# Example usage
print(minutes_to_hours(120)) # Output: 2.0
print(minutes_to_hours(75)) # Output: 1.25
print(minutes_to_hours(-30)) # Output: -1
This Python function uses a simple division to perform the conversion. Crucially, it includes error handling for negative input, returning -1 to indicate invalid input. This robust approach prevents unexpected behavior and enhances the function's reliability. The docstrings clearly explain the function's purpose, arguments, and return value, crucial for maintainability and readability.
JavaScript
function minutesToHours(minutes) {
// Input validation: Check for non-negative numbers
if (minutes < 0) {
return -1; // Indicate invalid input
}
return minutes / 60;
}
// Example Usage
console.log(minutesToHours(120)); // Output: 2
console.log(minutesToHours(90)); // Output: 1.5
console.log(minutesToHours(-15)); // Output: -1
The JavaScript equivalent follows a similar structure. Input validation ensures that the function handles invalid input gracefully, returning -1 for negative values. The concise code maintains readability while prioritizing robustness.
Java
public class MinutesToHoursConverter {
public static double minutesToHours(double minutes) {
if (minutes < 0) {
return -1; // Handle negative input
}
return minutes / 60;
}
public static void main(String[] args) {
System.out.println(minutesToHours(180)); // Output: 3.0
System.out.println(minutesToHours(60)); // Output: 1.0
System.out.println(minutesToHours(-45)); // Output: -1.0
}
}
The Java implementation demonstrates the use of a double
data type to accommodate decimal values for hours. The main
method provides example usage, showcasing the function's capabilities. The use of a separate class encapsulates the function, promoting better code organization.
C#
public class MinutesToHoursConverter
{
public static double MinutesToHours(double minutes)
{
if (minutes < 0)
{
return -1; // Handle negative input
}
return minutes / 60;
}
public static void Main(string[] args)
{
Console.WriteLine(MinutesToHours(240)); // Output: 4
Console.WriteLine(MinutesToHours(30)); // Output: 0.5
Console.WriteLine(MinutesToHours(-90)); // Output: -1
}
}
The C# code mirrors the Java structure, using a double
for precision and a Main
method for demonstration. The emphasis on clear structure and error handling remains consistent across languages.
C++
#include
double minutesToHours(double minutes) {
if (minutes < 0) {
return -1; // Handle negative input
}
return minutes / 60;
}
int main() {
std::cout << minutesToHours(300) << std::endl; // Output: 5
std::cout << minutesToHours(15) << std::endl; // Output: 0.25
std::cout << minutesToHours(-60) << std::endl; // Output: -1
return 0;
}
The C++ version utilizes double
for precision and includes error handling for negative inputs. The main
function serves as a testing ground for the minutesToHours
function.
Handling Edge Cases and Advanced Scenarios
While the basic conversion is straightforward, let's consider more complex scenarios:
Handling Non-Integer Inputs
All the examples above gracefully handle non-integer (floating-point) minute values. The division operation naturally produces a floating-point result, representing the fractional part of an hour.
Rounding and Precision
For applications requiring specific levels of precision, you might need to incorporate rounding functions. For instance, you could use round()
in Python, Math.round()
in JavaScript, or Math.round()
in Java to round the result to the nearest whole number.
Error Handling and Input Validation
Thorough error handling is crucial. Consider adding checks for:
- Negative minutes: As demonstrated in the examples, negative minute values are invalid and should be handled appropriately (e.g., returning an error code or throwing an exception).
- Non-numeric input: If your function might receive non-numeric input, include type checking to prevent errors.
- Extremely large inputs: Very large minute values might lead to overflow errors. Consider adding checks to prevent this.
Extending Functionality
The function can be extended to include additional features:
- Displaying the result in a specific format: Instead of just returning the numerical value, format the output as "HH:MM" or similar.
- Converting to other units: Expand the function to convert minutes to seconds, days, or other time units.
- Adding unit labels to the output: The output could be enhanced to include units (e.g., "2.5 hours").
Best Practices for Function Design
- Clear and concise naming: Use descriptive names that clearly communicate the function's purpose (e.g.,
minutesToHours
). - Meaningful comments and docstrings: Provide clear explanations of the function's behavior, arguments, and return values.
- Modular design: Keep the function focused on a single task (converting minutes to hours). Avoid adding unnecessary complexity.
- Error handling: Implement robust error handling to gracefully manage invalid inputs and potential exceptions.
- Testability: Design the function to be easily testable with unit tests.
Conclusion
Converting minutes to hours is a fundamental task with numerous applications in programming. By mastering the implementation of this conversion function in different programming languages, and by adhering to best practices in function design and error handling, you can create robust, efficient, and maintainable code that is ready to handle a variety of scenarios. Remember that the seemingly simple task of converting units presents excellent opportunities to practice coding principles such as modularity, error handling, and the efficient use of data types. Continuously refining your skills in these areas will make you a more proficient and effective programmer.
Latest Posts
Related Post
Thank you for visiting our website which covers about Complete The Function Definition To Return The Hours Given Minutes. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.