C First Semester Final Project Airline Reservations Project
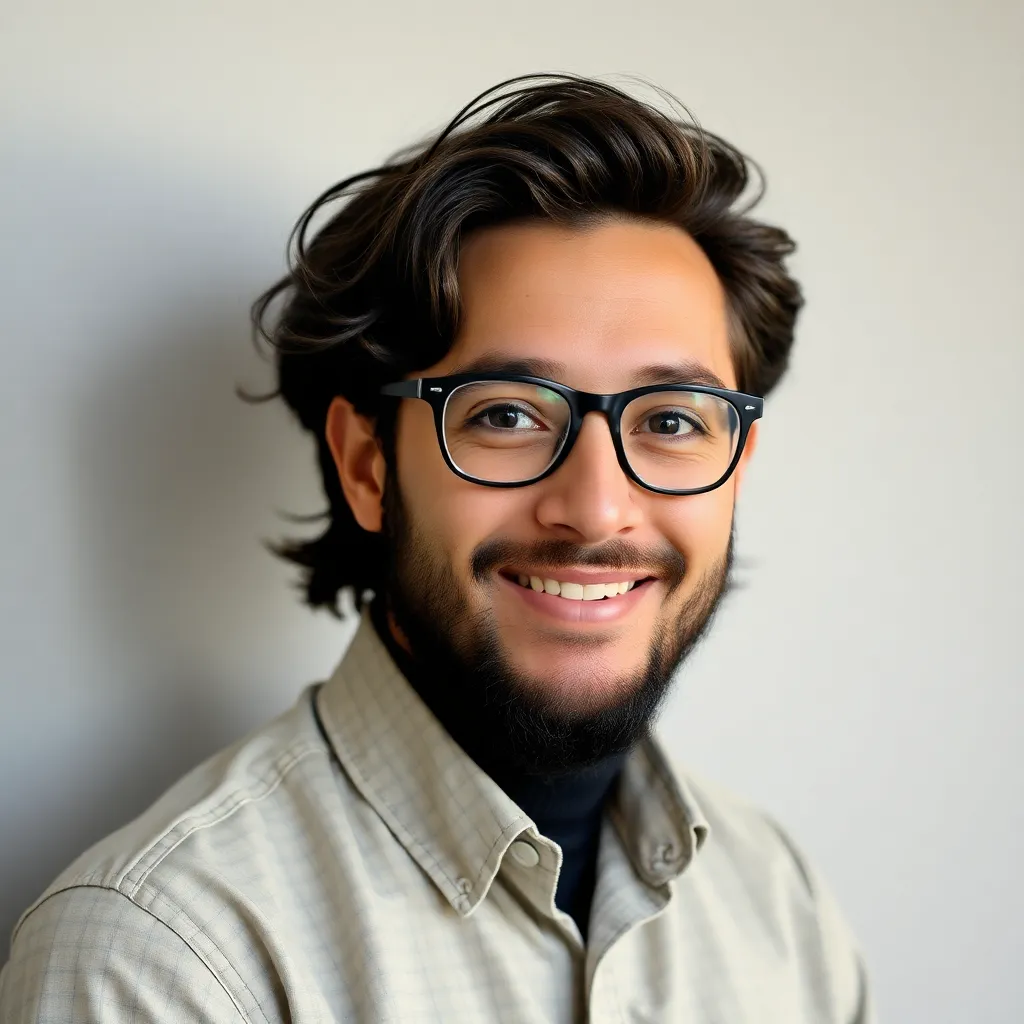
Holbox
Mar 14, 2025 · 6 min read

Table of Contents
- C First Semester Final Project Airline Reservations Project
- Table of Contents
- C First Semester Final Project: Airline Reservation System
- Project Overview: Building an Airline Reservation System in C
- Project Design and Data Structures
- Data Structures:
- Functions:
- Implementation Details: Code Snippets and Explanations
- Function: displayFlights()
- Function: bookFlight()
- Function: generateBookingID()
- File Handling (Saving and Loading Data):
- Testing and Refinement
- Advanced Features (Optional)
- Conclusion: A Successful C Project
- Latest Posts
- Latest Posts
- Related Post
C First Semester Final Project: Airline Reservation System
Congratulations on reaching the final semester of your C programming journey! A common and challenging project for first-semester students is building an Airline Reservation System. This comprehensive guide will walk you through the design, implementation, and testing phases of such a project, equipping you with the knowledge and code snippets to successfully complete your final project.
Project Overview: Building an Airline Reservation System in C
This project aims to create a command-line based Airline Reservation System using the C programming language. The system will allow users to perform various tasks, including:
- Viewing available flights: Displaying flight details like flight number, origin, destination, departure time, arrival time, and available seats.
- Booking a flight: Reserving seats on a chosen flight. The system should handle seat availability and update the number of available seats accordingly.
- Cancelling a flight booking: Removing a reservation and updating seat availability.
- Viewing booking details: Allowing users to view their existing bookings.
- Searching for flights: Filtering flights based on origin, destination, or date.
- Data persistence: Storing and retrieving flight and booking information. This could involve using files to store data or a simple in-memory database.
Project Design and Data Structures
Before diving into the code, it's crucial to design the data structures and overall architecture. This will significantly simplify the development process and prevent future complications.
Data Structures:
We'll need several data structures to effectively manage flight and booking information. Here's a suggested approach:
Flight
structure: This structure will hold details for each flight:
typedef struct {
int flightNumber;
char origin[50];
char destination[50];
char departureTime[50]; //Consider using a time structure for better date/time handling.
char arrivalTime[50]; //Consider using a time structure for better date/time handling.
int totalSeats;
int availableSeats;
} Flight;
Booking
structure: This will store booking details:
typedef struct {
int bookingID;
int flightNumber;
char passengerName[100];
int numberOfSeats;
} Booking;
- Arrays or Linked Lists: You can use arrays or linked lists to store multiple
Flight
andBooking
structures. Linked lists offer more dynamic memory management, but arrays are simpler to implement for beginners. For this project, arrays are sufficient if the number of flights and bookings is relatively small.
Functions:
Breaking down the system into smaller, modular functions is crucial for maintainability and readability. Some key functions you'll need include:
loadFlights()
: Loads flight data from a file (or initializes it if a file doesn't exist).displayFlights()
: Displays a list of available flights.searchFlights()
: Searches for flights based on user-specified criteria (origin, destination, date).bookFlight()
: Handles flight booking, updating seat availability.cancelBooking()
: Cancels a booking and updates seat availability.viewBooking()
: Allows users to view their booking details.saveFlights()
: Saves updated flight data to a file.generateBookingID()
: Generates unique booking IDs.
Implementation Details: Code Snippets and Explanations
Let's delve into code snippets for some crucial functions. Remember, error handling (like checking for invalid user input) is crucial and should be implemented throughout the code.
Function: displayFlights()
void displayFlights(Flight flights[], int numFlights) {
if (numFlights == 0) {
printf("No flights available.\n");
return;
}
printf("-------------------------------------------------------------------------\n");
printf("| Flight No | Origin | Destination | Departure Time | Arrival Time |\n");
printf("-------------------------------------------------------------------------\n");
for (int i = 0; i < numFlights; i++) {
if (flights[i].availableSeats > 0) { //Only show flights with available seats.
printf("| %-9d | %-11s | %-13s | %-14s | %-12s |\n",
flights[i].flightNumber, flights[i].origin, flights[i].destination,
flights[i].departureTime, flights[i].arrivalTime);
}
}
printf("-------------------------------------------------------------------------\n");
}
Function: bookFlight()
void bookFlight(Flight flights[], Booking bookings[], int numFlights, int *numBookings) {
int flightNumber, numSeats;
char passengerName[100];
// Get flight number from user (input validation needed!)
printf("Enter flight number: ");
scanf("%d", &flightNumber);
// Find flight (input validation needed to check if flight number exists)
int flightIndex = -1;
for (int i = 0; i < numFlights; i++) {
if (flights[i].flightNumber == flightNumber) {
flightIndex = i;
break;
}
}
if(flightIndex == -1){
printf("Invalid flight number.\n");
return;
}
// Get passenger name and number of seats (input validation needed!)
printf("Enter passenger name: ");
getchar(); // Consume newline character left by scanf
fgets(passengerName, sizeof(passengerName), stdin);
passengerName[strcspn(passengerName, "\n")] = 0; //Remove trailing newline from fgets
printf("Enter number of seats to book: ");
scanf("%d", &numSeats);
if (flights[flightIndex].availableSeats >= numSeats) {
bookings[*numBookings].bookingID = generateBookingID(); //Implement generateBookingID function.
bookings[*numBookings].flightNumber = flightNumber;
strcpy(bookings[*numBookings].passengerName, passengerName);
bookings[*numBookings].numberOfSeats = numSeats;
flights[flightIndex].availableSeats -= numSeats;
(*numBookings)++;
printf("Booking successful!\n");
} else {
printf("Not enough seats available on this flight.\n");
}
}
Function: generateBookingID()
This function needs to generate unique booking IDs. A simple approach is to use a counter that increments with each booking. More robust methods might involve using timestamps or random number generation with collision checking.
int generateBookingID() {
static int bookingIDCounter = 1; // Static variable to retain value between calls.
return bookingIDCounter++;
}
File Handling (Saving and Loading Data):
You'll need functions to save and load flight and booking data to/from files. Consider using binary files for efficient storage or text files for easier debugging. Example (using text files):
//Simplified example; Robust error handling and data validation is needed.
void saveFlights(Flight flights[], int numFlights, const char* filename){
FILE *fp = fopen(filename, "w");
// Write flight data to file (Use fprintf to write each field).
fclose(fp);
}
void loadFlights(Flight flights[], int *numFlights, const char* filename){
FILE *fp = fopen(filename, "r");
// Read flight data from file (Use fscanf to read each field).
fclose(fp);
}
Testing and Refinement
Thorough testing is essential. Test each function individually, then test the entire system. Consider these test cases:
- Booking a flight with available seats: Verify that seat availability updates correctly.
- Booking a flight with insufficient seats: Ensure the system handles this scenario gracefully.
- Cancelling a booking: Verify that seat availability is updated correctly.
- Searching for flights using different criteria: Test with various combinations of origin, destination, and dates.
- Handling invalid user input: Make sure the system doesn't crash or behave unexpectedly with incorrect inputs.
- Data persistence: Verify that data is correctly saved and loaded from the file.
Advanced Features (Optional)
Once you've completed the core functionality, consider adding these advanced features to enhance your project:
- Improved User Interface: Instead of a simple command-line interface, you could explore using a library like ncurses to create a more visually appealing interface.
- More Sophisticated Search: Implement more advanced search filters (e.g., price range, specific airlines).
- User Accounts: Allow users to create accounts and manage their bookings. This will likely require a more sophisticated data storage mechanism (e.g., a database).
- Error Handling and Input Validation: Add more robust error handling and input validation to prevent crashes and unexpected behavior.
- Date/Time Handling: Use a dedicated date/time library for better date and time management.
Conclusion: A Successful C Project
Building an Airline Reservation System in C for your final project is a significant undertaking, but by following this guide and breaking down the problem into smaller, manageable tasks, you can successfully complete it. Remember to focus on good coding practices, thorough testing, and clear documentation. Good luck! Your hard work will pay off! Remember that this is a complex project, and the provided code snippets are meant to be a starting point, not a complete solution. You'll need to fill in many details and add significant error handling and input validation to ensure your program is robust and reliable.
Latest Posts
Latest Posts
-
How Many Grams Is 14 Oz
May 19, 2025
-
How Much Is 53 Kg In Pounds
May 19, 2025
-
How Many Feet Is 35 Inches
May 19, 2025
-
100 Yards Is How Many Feet
May 19, 2025
-
35 Km Is How Many Miles
May 19, 2025
Related Post
Thank you for visiting our website which covers about C First Semester Final Project Airline Reservations Project . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.