Applied Numerical Methods With Matlab For Engineers And Scientists
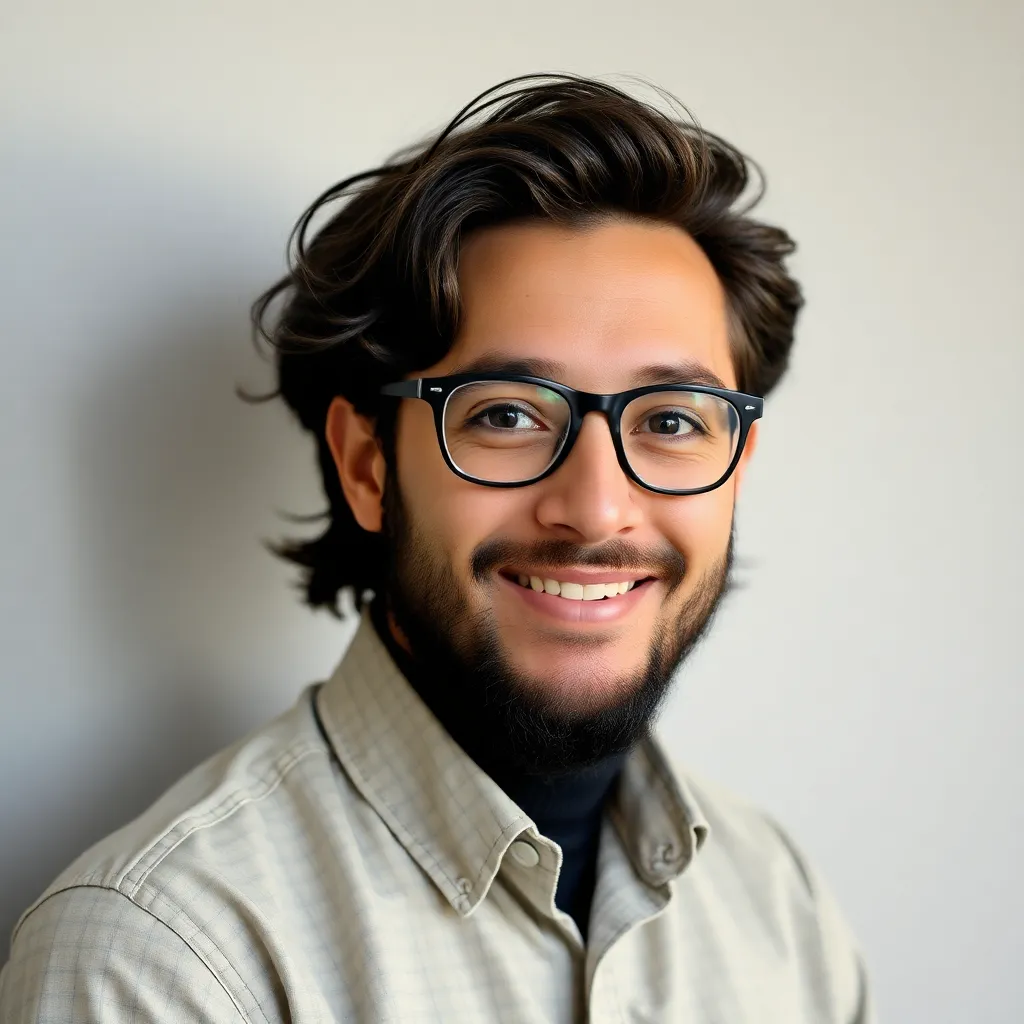
Holbox
May 11, 2025 · 7 min read
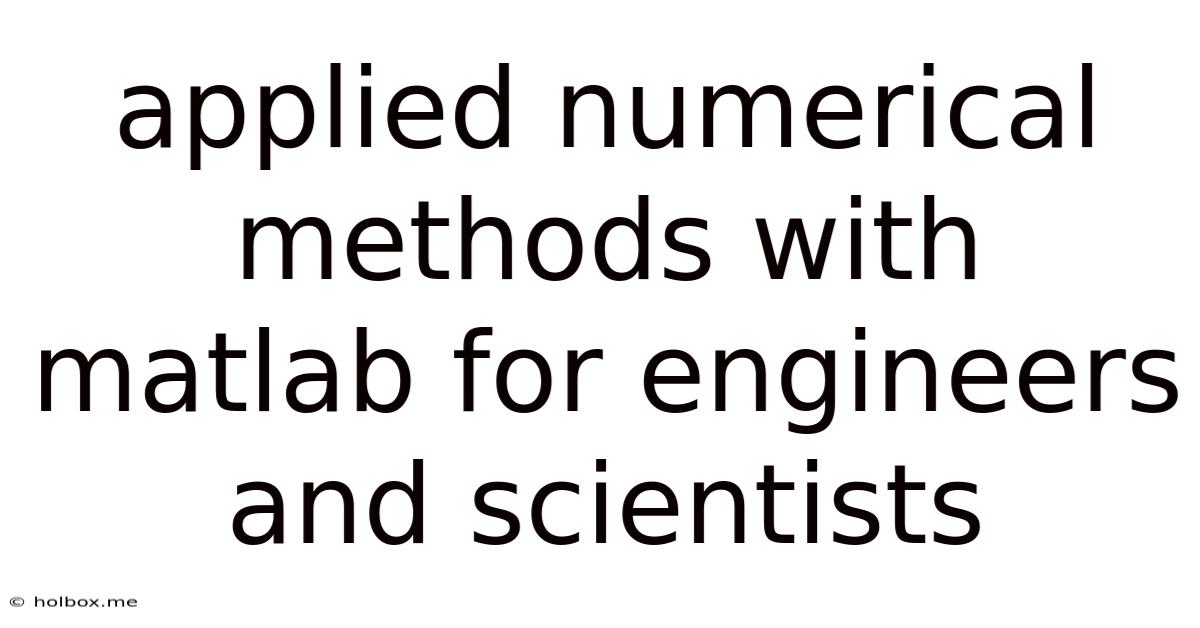
Table of Contents
- Applied Numerical Methods With Matlab For Engineers And Scientists
- Table of Contents
- Applied Numerical Methods with MATLAB for Engineers and Scientists
- I. Introduction to Numerical Methods and MATLAB
- Why Use MATLAB for Numerical Methods?
- II. Root Finding Methods
- A. Bisection Method
- B. Newton-Raphson Method
- C. Secant Method
- III. Solving Systems of Linear Equations
- A. Gaussian Elimination
- B. LU Decomposition
- C. Iterative Methods (Jacobi, Gauss-Seidel)
- IV. Numerical Integration and Differentiation
- A. Numerical Integration (Trapezoidal Rule, Simpson's Rule)
- B. Numerical Differentiation
- V. Ordinary Differential Equations (ODEs)
- A. Euler's Method
- B. Runge-Kutta Methods
- VI. Partial Differential Equations (PDEs)
- A. Finite Difference Method
- VII. Interpolation and Approximation
- A. Polynomial Interpolation (Lagrange, Newton)
- B. Spline Interpolation
- VIII. Conclusion
- Latest Posts
- Related Post
Applied Numerical Methods with MATLAB for Engineers and Scientists
Numerical methods are essential tools for engineers and scientists tackling complex problems that lack analytical solutions. MATLAB, with its powerful built-in functions and intuitive interface, provides a robust platform for implementing these methods. This article delves into various applied numerical methods, showcasing their implementation in MATLAB and highlighting their applications in engineering and scientific fields.
I. Introduction to Numerical Methods and MATLAB
Numerical methods are algorithms that provide approximate solutions to mathematical problems. They are crucial when analytical solutions are either impossible or computationally expensive to obtain. These methods often involve iterative processes, approximating the solution through successive refinements.
MATLAB (MATrix LABoratory) is a high-level programming language and interactive environment specifically designed for numerical computation and visualization. Its extensive libraries, including the Symbolic Math Toolbox and the Partial Differential Equation Toolbox, provide a vast array of functions tailored to numerical analysis. MATLAB's ease of use and powerful visualization capabilities make it an ideal platform for implementing and analyzing numerical methods.
Why Use MATLAB for Numerical Methods?
- Extensive Libraries: MATLAB boasts a comprehensive set of built-in functions for various numerical methods, including linear algebra, optimization, differential equations, and more. This significantly reduces development time and effort.
- Ease of Use: MATLAB's syntax is relatively straightforward, making it easier to learn and use compared to other programming languages like C++ or Fortran.
- Powerful Visualization: MATLAB's plotting and visualization capabilities allow for easy interpretation of results, making it easier to identify patterns and trends in data.
- Interactive Environment: MATLAB's interactive environment allows for quick testing and modification of code, accelerating the development process.
- Widely Used: MATLAB is a standard tool in many engineering and scientific disciplines, making it a valuable skill to possess.
II. Root Finding Methods
Root finding, or finding the zeros of a function, is a fundamental problem in many engineering and scientific applications. Several numerical methods exist for this purpose.
A. Bisection Method
The bisection method is a simple yet robust iterative method that relies on repeatedly halving an interval containing a root. The method requires an initial interval [a, b] such that f(a) and f(b) have opposite signs, guaranteeing a root within the interval. The midpoint of the interval is then evaluated, and the interval is halved based on the sign of the function at the midpoint.
MATLAB Implementation:
function root = bisection(f, a, b, tol)
% Bisection method for finding a root of f(x) in [a, b]
% with tolerance tol
while (b - a) / 2 > tol
c = (a + b) / 2;
if f(c) == 0
root = c;
return;
elseif f(a) * f(c) < 0
b = c;
else
a = c;
end
end
root = (a + b) / 2;
end
B. Newton-Raphson Method
The Newton-Raphson method is a faster iterative method that uses the derivative of the function to refine the root estimate. It converges quadratically (i.e., the number of correct digits roughly doubles with each iteration) near the root, making it very efficient.
MATLAB Implementation:
function root = newtonraphson(f, df, x0, tol)
% Newton-Raphson method for finding a root of f(x)
% starting at x0 with tolerance tol
x = x0;
while abs(f(x)) > tol
x = x - f(x) / df(x);
end
root = x;
end
C. Secant Method
The secant method is a variation of the Newton-Raphson method that avoids the need to explicitly compute the derivative. Instead, it approximates the derivative using a finite difference approximation.
MATLAB Implementation:
function root = secant(f, x0, x1, tol)
% Secant method for finding a root of f(x)
% starting at x0 and x1 with tolerance tol
x2 = x1 - f(x1) * (x1 - x0) / (f(x1) - f(x0));
while abs(x2 - x1) > tol
x0 = x1;
x1 = x2;
x2 = x1 - f(x1) * (x1 - x0) / (f(x1) - f(x0));
end
root = x2;
end
III. Solving Systems of Linear Equations
Solving systems of linear equations is a ubiquitous task in engineering and science. Direct and iterative methods exist for this purpose.
A. Gaussian Elimination
Gaussian elimination is a direct method that systematically transforms the augmented matrix of the system into an upper triangular form. Back substitution is then used to solve for the unknowns.
MATLAB Implementation: MATLAB's built-in \
operator efficiently solves linear systems using optimized algorithms including Gaussian elimination.
A = [1 2 3; 4 5 6; 7 8 9];
b = [10; 11; 12];
x = A \ b; % Solves Ax = b
B. LU Decomposition
LU decomposition factors a matrix into a lower triangular matrix (L) and an upper triangular matrix (U). This factorization can be efficiently used to solve multiple systems with the same coefficient matrix.
MATLAB Implementation: MATLAB's lu
function performs LU decomposition.
A = [1 2 3; 4 5 6; 7 8 9];
[L, U] = lu(A);
b = [10; 11; 12];
y = L \ b;
x = U \ y; % Solves Ax = b using LU decomposition
C. Iterative Methods (Jacobi, Gauss-Seidel)
Iterative methods, such as Jacobi and Gauss-Seidel, are suitable for large sparse systems. They generate a sequence of approximate solutions that converge to the true solution.
MATLAB Implementation: While MATLAB doesn't have built-in functions for basic Jacobi and Gauss-Seidel, they can be easily implemented. (Implementation omitted for brevity due to space constraints, but readily available online).
IV. Numerical Integration and Differentiation
Numerical integration and differentiation are crucial for problems where analytical solutions are unavailable or difficult to obtain.
A. Numerical Integration (Trapezoidal Rule, Simpson's Rule)
Numerical integration approximates the definite integral of a function. The trapezoidal rule approximates the area under the curve using trapezoids, while Simpson's rule uses parabolas for a more accurate approximation.
MATLAB Implementation: MATLAB's trapz
and quad
functions perform numerical integration using various methods.
% Trapezoidal rule
x = linspace(0, 1, 100);
y = x.^2;
integral_trapz = trapz(x, y);
% Quadrature (more accurate)
integral_quad = quad(@(x) x.^2, 0, 1);
B. Numerical Differentiation
Numerical differentiation approximates the derivative of a function using finite difference approximations. Forward, backward, and central difference formulas are commonly used.
MATLAB Implementation: While MATLAB doesn't have a single function for all difference methods, they are easily implemented. (Implementation omitted for brevity but readily available online).
V. Ordinary Differential Equations (ODEs)
Many engineering and scientific problems are modeled using ordinary differential equations. Numerical methods are necessary for solving these equations when analytical solutions are unavailable.
A. Euler's Method
Euler's method is a simple first-order method that approximates the solution by following the tangent line at each point.
MATLAB Implementation: (Implementation omitted for brevity, but easily implemented).
B. Runge-Kutta Methods
Runge-Kutta methods are higher-order methods that offer improved accuracy compared to Euler's method. The most common is the fourth-order Runge-Kutta method.
MATLAB Implementation: MATLAB's ode45
function is a highly optimized implementation of a Runge-Kutta method.
% Define the ODE function
f = @(t, y) -y; % Example: dy/dt = -y
% Solve the ODE
[t, y] = ode45(f, [0 1], 1); % Solve from t=0 to t=1 with initial condition y(0)=1
% Plot the solution
plot(t, y);
VI. Partial Differential Equations (PDEs)
Partial differential equations (PDEs) model many complex physical phenomena. Numerical methods are crucial for solving PDEs, often involving discretization techniques such as finite difference, finite element, or finite volume methods.
A. Finite Difference Method
The finite difference method approximates the derivatives in a PDE using finite difference approximations. This transforms the PDE into a system of algebraic equations that can be solved numerically.
MATLAB Implementation: MATLAB's Partial Differential Equation Toolbox provides tools for solving PDEs using the finite element method.
VII. Interpolation and Approximation
Interpolation involves finding a function that passes through a given set of data points, while approximation finds a function that closely fits the data without necessarily passing through all points.
A. Polynomial Interpolation (Lagrange, Newton)
Polynomial interpolation constructs a polynomial that passes through all given data points. Lagrange and Newton interpolation methods are commonly used.
MATLAB Implementation: MATLAB's interp1
function performs various types of interpolation.
x = [1 2 3 4];
y = [2 4 1 3];
xi = 2.5;
yi = interp1(x, y, xi, 'linear'); % Linear interpolation
B. Spline Interpolation
Spline interpolation uses piecewise polynomial functions to interpolate data, resulting in smoother curves than polynomial interpolation.
MATLAB Implementation: MATLAB's spline
function performs spline interpolation.
VIII. Conclusion
This article provided an overview of various applied numerical methods and their implementation in MATLAB. MATLAB's powerful capabilities make it an invaluable tool for engineers and scientists working with numerical methods. Mastering these techniques is crucial for solving a wide range of complex problems in various scientific and engineering disciplines. Remember to always consider the limitations and potential errors associated with numerical methods and choose the most appropriate method based on the specific problem and desired accuracy. Further exploration into specific methods and advanced techniques within each category is highly encouraged.
Latest Posts
Related Post
Thank you for visiting our website which covers about Applied Numerical Methods With Matlab For Engineers And Scientists . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.