An Element In A 2d List Is A ______
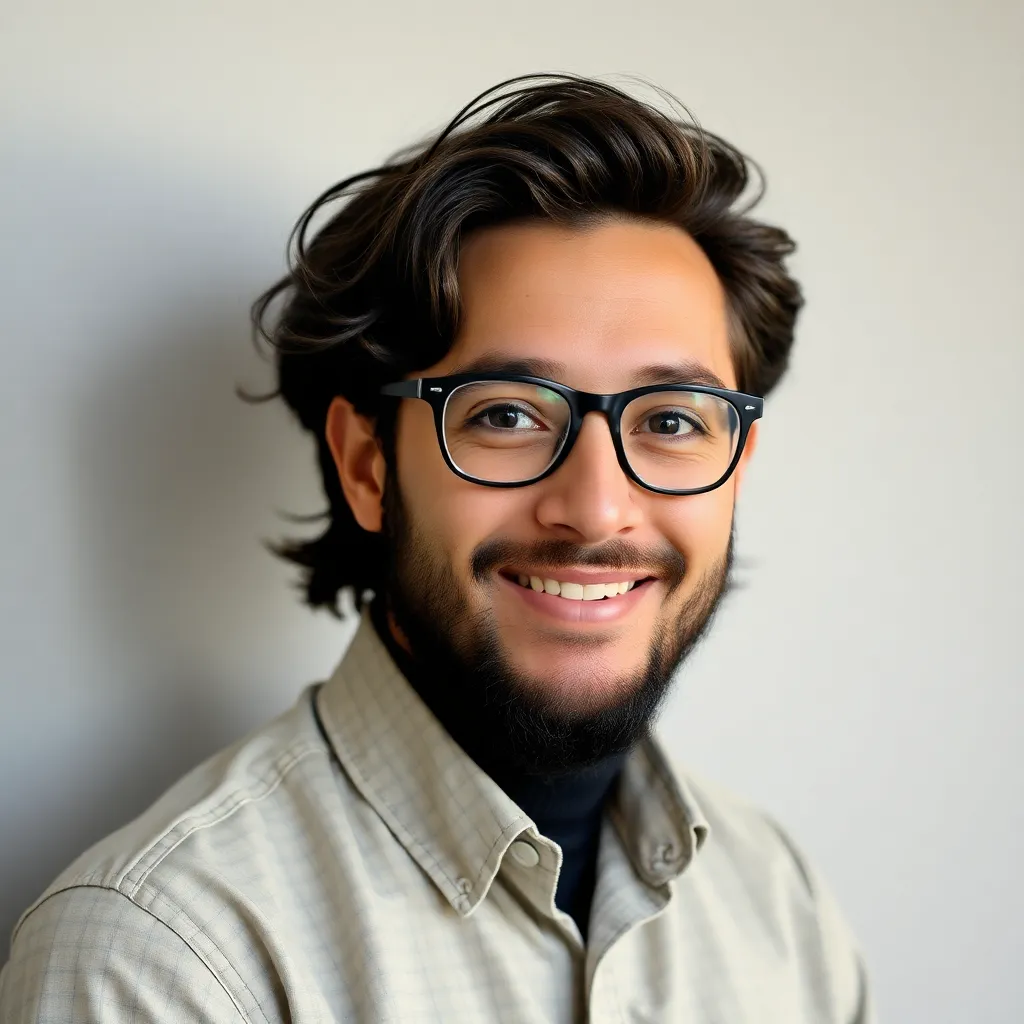
Holbox
Apr 02, 2025 · 5 min read
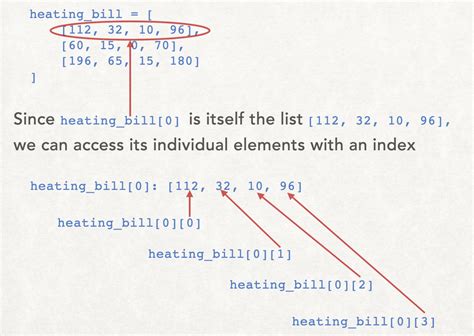
Table of Contents
- An Element In A 2d List Is A ______
- Table of Contents
- An Element in a 2D List is a ______: Understanding Data Structures in Programming
- What is a 2D List?
- Defining an Element in a 2D List
- Accessing Elements: Indexing and Slicing
- Manipulating Elements
- Applications of 2D Lists
- 1. Representing Matrices in Linear Algebra
- 2. Image Processing
- 3. Game Development
- 4. Data Visualization
- 5. Graph Representation (Adjacency Matrix)
- 6. Spreadsheet Data
- Common Mistakes and Best Practices
- Advanced Concepts and Extensions
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
An Element in a 2D List is a ______: Understanding Data Structures in Programming
A 2D list, also known as a nested list or matrix, is a fundamental data structure in programming used to represent tabular data or grids. Understanding what constitutes an "element" within this structure is crucial for effective programming. This article delves deep into the concept, exploring its properties, accessing elements, manipulating them, and its applications across various programming paradigms.
What is a 2D List?
Before we define what an element is, let's solidify our understanding of a 2D list itself. A 2D list is essentially a list of lists. Each inner list represents a row in the structure, and each element within those inner lists represents a single data point at a specific row and column coordinate. Imagine a spreadsheet; the rows are the inner lists, and each cell within a row is an element.
Example (Python):
my_2d_list = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
In this example, my_2d_list
is a 2D list containing three inner lists (rows). Each number (1, 2, 3, etc.) is an individual element.
Defining an Element in a 2D List
Now, to answer the core question: an element in a 2D list is a single data item residing at a specific location within the nested list structure. It's the fundamental building block of the entire 2D list. This data item can be of any data type supported by your programming language:
- Integers:
10
,-5
,0
- Floating-point numbers:
3.14
,-2.71
,0.0
- Strings:
"hello"
,"world"
,"python"
- Booleans:
True
,False
- Other data structures: Even other lists, or custom objects, can be elements within a 2D list, creating more complex data structures.
Accessing Elements: Indexing and Slicing
Accessing specific elements within a 2D list involves using indexing, a method of specifying the element's location using its row and column coordinates. Remember that indexing usually starts at 0.
Example (Python):
To access the element '5' in my_2d_list
above, we would use:
element = my_2d_list[1][1] # Row 1 (index 1), Column 1 (index 1)
print(element) # Output: 5
This illustrates that accessing an element requires specifying two indices: one for the outer list (row) and one for the inner list (column).
Slicing: Slicing allows you to extract portions of the 2D list. For instance, you could extract a sub-matrix, a row, or a column.
# Extract the first row
first_row = my_2d_list[0] # Output: [1, 2, 3]
# Extract the second column
second_column = [row[1] for row in my_2d_list] # Output: [2, 5, 8]
# Extract a sub-matrix (top-left 2x2)
sub_matrix = [row[:2] for row in my_2d_list[:2]] # Output: [[1, 2], [4, 5]]
Manipulating Elements
Once you've accessed an element, you can modify its value.
my_2d_list[1][2] = 10 #Change the element at row 1, column 2 from 6 to 10.
print(my_2d_list) # Output: [[1, 2, 3], [4, 5, 10], [7, 8, 9]]
You can also add new elements (rows or individual elements) or remove existing ones using methods like append()
, insert()
, pop()
, del
, and list comprehensions.
Applications of 2D Lists
The versatility of 2D lists makes them applicable in a wide range of scenarios:
1. Representing Matrices in Linear Algebra
2D lists are ideal for representing matrices in numerical computations, allowing for operations like matrix addition, multiplication, and transposition.
2. Image Processing
Images are often represented as 2D arrays, where each element represents the color value of a pixel. Image manipulation tasks like filtering, resizing, and edge detection can be performed using 2D list operations.
3. Game Development
Game maps or boards can be efficiently modeled using 2D lists, with each element representing a game object (player, obstacle, item).
4. Data Visualization
Data visualization libraries often use 2D lists to represent the data to be plotted on graphs or charts.
5. Graph Representation (Adjacency Matrix)
A graph, a fundamental data structure in computer science, can be represented using an adjacency matrix, which is a 2D list where each element indicates the existence of an edge between two nodes.
6. Spreadsheet Data
The data in a spreadsheet program can be naturally represented as a 2D list. This allows for easy manipulation and analysis of tabular data.
Common Mistakes and Best Practices
1. Out-of-Bounds Errors: Attempting to access an element using an index that's outside the list's boundaries will result in an IndexError
. Always check the dimensions of your 2D list before accessing elements to prevent this error.
2. Modifying List Size During Iteration: Modifying the size of a list (adding or removing elements) while iterating through it can lead to unexpected behavior and errors. It's generally safer to create a new list or modify the list after the loop completes.
3. Inefficient Element Access: For very large 2D lists, repeatedly accessing elements using nested loops can be slow. Consider using optimized algorithms and data structures if performance is critical.
4. Data Type Consistency: While a 2D list can hold various data types, maintaining consistent data types within each inner list can simplify operations and improve readability.
5. Using Appropriate Data Structures: For specific tasks, other data structures might be more efficient than 2D lists. For example, NumPy arrays are highly optimized for numerical computations.
Advanced Concepts and Extensions
-
Sparse Matrices: For matrices with mostly zero values, specialized sparse matrix data structures can significantly reduce memory usage and improve performance.
-
Multi-dimensional Lists: The concept extends beyond 2D; you can create 3D lists (lists of lists of lists) and higher-dimensional lists to represent more complex data structures.
-
Object-Oriented Programming and 2D Lists: In object-oriented programming, you can encapsulate a 2D list within a custom class to create more structured and manageable representations of data, allowing for easier manipulation and maintenance of complex datasets.
Conclusion
An element in a 2D list is a single data item, the fundamental building block of this versatile data structure. Understanding how to access, manipulate, and utilize these elements is essential for effective programming across diverse domains. Mastering 2D lists empowers developers to tackle a wide array of problems, from simple data representation to complex computational tasks. Remember to follow best practices and choose the most efficient data structures for your specific application to write robust and performant code. The more you understand the intricacies of 2D lists, the more powerful and versatile your programming capabilities become.
Latest Posts
Latest Posts
-
What Is The Objects Position At T 2s
Apr 04, 2025
-
A Shift To Corporate Ownership Of Ranches Led To
Apr 04, 2025
-
The Capitalized Cost Of Equipment Excludes
Apr 04, 2025
-
A Projectile Is Launched From Ground Level
Apr 04, 2025
-
The Generic Types Of Competitive Strategies Include
Apr 04, 2025
Related Post
Thank you for visiting our website which covers about An Element In A 2d List Is A ______ . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.