A String Is A Sequence Of ____.
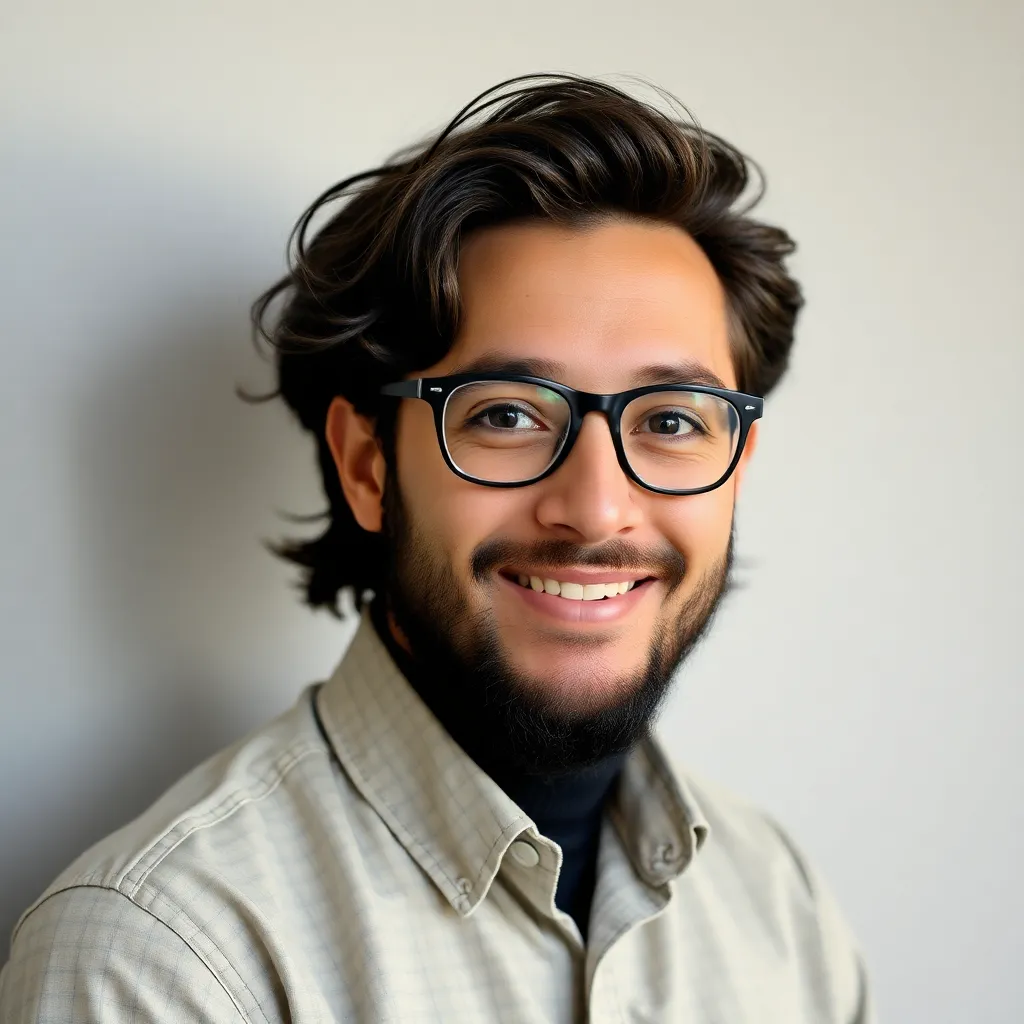
Holbox
May 12, 2025 · 7 min read
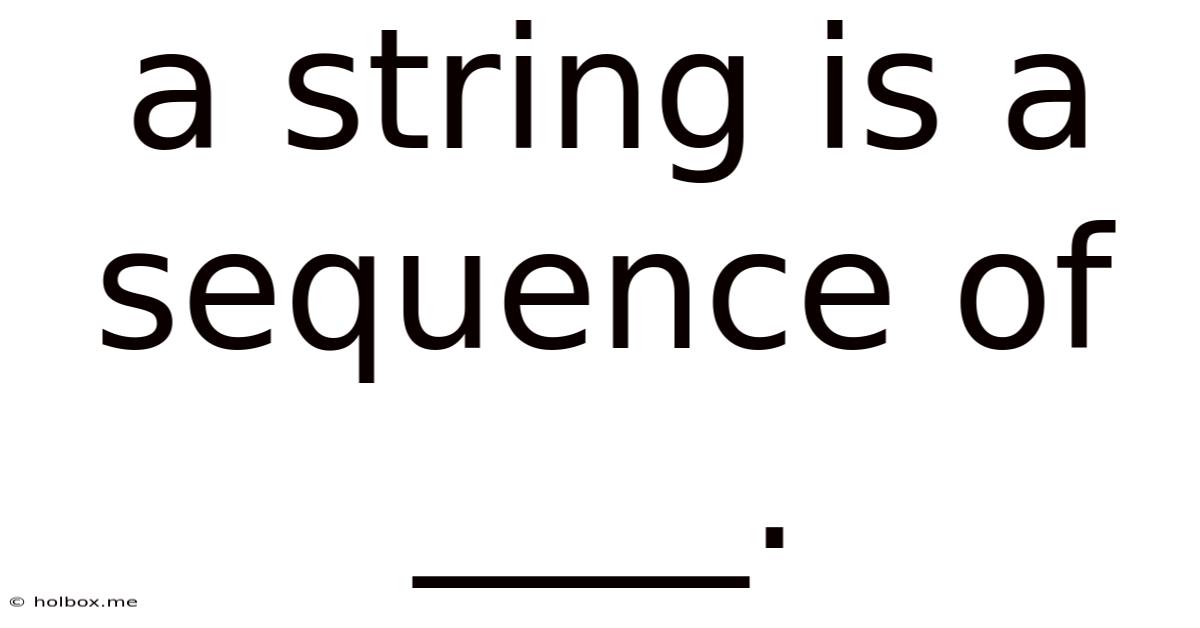
Table of Contents
- A String Is A Sequence Of ____.
- Table of Contents
- A String is a Sequence of Characters: A Deep Dive into String Manipulation and Applications
- Understanding the Essence of Strings
- Character Encoding: The Unspoken Language of Strings
- String Manipulation: The Power of Transformation
- 1. Concatenation: Joining Strings Together
- 2. Slicing and Substrings: Extracting Portions of Strings
- 3. Searching and Finding: Locating Substrings
- 4. Replacing: Substituting Substrings
- 5. Case Conversion: Changing the Case of Characters
- 6. Splitting and Joining: Breaking and Combining Strings
- 7. Trimming: Removing Leading and Trailing Whitespace
- Advanced String Operations and Techniques
- Regular Expressions: Powerful Pattern Matching
- String Formatting: Creating Readable Output
- Applications of Strings: A Wide-Ranging Impact
- 1. Web Development: The Foundation of Websites
- 2. Data Science and Analysis: Working with Textual Data
- 3. Natural Language Processing (NLP): Understanding Human Language
- 4. Software Development: User Interaction and Data Storage
- Optimization Strategies for Efficient String Processing
- 1. String Immutability: Understanding Its Implications
- 2. Algorithmic Efficiency: Choosing the Right Approach
- 3. Memory Management: Avoiding Unnecessary Copies
- 4. Data Structures: Selecting Appropriate Data Structures
- Conclusion: The Enduring Significance of Strings
- Latest Posts
- Related Post
A String is a Sequence of Characters: A Deep Dive into String Manipulation and Applications
A string is a sequence of characters. This seemingly simple definition belies the incredible power and versatility of strings in computer science and programming. Strings are fundamental data structures, forming the backbone of much of the text-based interaction we experience daily, from websites and software applications to data analysis and machine learning. This comprehensive guide will explore the intricacies of strings, delving into their structure, manipulation techniques, common applications, and the optimization strategies for efficient string processing.
Understanding the Essence of Strings
At its core, a string is an ordered collection of characters. These characters can include letters (both uppercase and lowercase), numbers, symbols, and whitespace characters (spaces, tabs, newlines). The crucial aspect is the order; changing the order of characters within a string fundamentally alters its meaning. For instance, "hello" is distinct from "olleh."
Different programming languages handle strings in slightly different ways, but the underlying principle remains consistent: a string is a sequence of characters treated as a single unit. This allows us to perform operations on the entire string or individual characters within it.
Character Encoding: The Unspoken Language of Strings
Before diving into string manipulation, it's essential to understand character encoding. Character encoding is the method used to represent characters as numerical values. Common encodings include ASCII (American Standard Code for Information Interchange), UTF-8 (Unicode Transformation Format-8-bit), and UTF-16. These encodings determine how much memory a character occupies and how different characters are mapped to numerical values. Understanding character encoding is crucial for ensuring that your strings are correctly interpreted across different systems and languages. Incorrect encoding can lead to garbled text or unexpected behavior.
String Manipulation: The Power of Transformation
Strings are not static entities; they can be manipulated and transformed in numerous ways. This manipulation forms the core of many programming tasks, from simple text processing to sophisticated natural language processing.
1. Concatenation: Joining Strings Together
Concatenation is the process of combining two or more strings into a single string. Most programming languages provide operators or functions to perform concatenation. For example, in Python, the +
operator concatenates strings:
string1 = "Hello"
string2 = " World"
combined_string = string1 + string2 # combined_string will be "Hello World"
2. Slicing and Substrings: Extracting Portions of Strings
Slicing allows you to extract a portion of a string, creating a substring. This is achieved by specifying the starting and ending indices of the desired portion. Many languages use zero-based indexing, where the first character has an index of 0.
my_string = "This is a sample string"
substring = my_string[5:8] # substring will be "is a"
3. Searching and Finding: Locating Substrings
Finding specific substrings within a larger string is a common task. Programming languages offer functions like find()
or index()
to locate the position of a substring within a string.
text = "The quick brown fox jumps over the lazy dog"
position = text.find("fox") # position will be 16 (the index of the first occurrence of "fox")
4. Replacing: Substituting Substrings
Replacing parts of a string is another essential manipulation. Functions like replace()
allow you to substitute occurrences of a substring with another string.
original_string = "This is a test string"
new_string = original_string.replace("test", "sample") # new_string will be "This is a sample string"
5. Case Conversion: Changing the Case of Characters
Converting the case of characters (uppercase to lowercase, or vice-versa) is often necessary for text processing tasks. Functions like lower()
and upper()
are readily available in most languages.
mixed_case = "HeLlO wOrLd"
lowercase = mixed_case.lower() # lowercase will be "hello world"
uppercase = mixed_case.upper() # uppercase will be "HELLO WORLD"
6. Splitting and Joining: Breaking and Combining Strings
Splitting a string into a list of substrings based on a delimiter is a common operation. Conversely, joining a list of strings into a single string is also frequently needed. Functions like split()
and join()
facilitate these tasks.
sentence = "This,is,a,sentence"
words = sentence.split(",") # words will be ['This', 'is', 'a', 'sentence']
joined_sentence = " ".join(words) # joined_sentence will be "This is a sentence"
7. Trimming: Removing Leading and Trailing Whitespace
Whitespace characters (spaces, tabs, newlines) at the beginning or end of a string can be problematic. Functions like strip()
, lstrip()
, and rstrip()
remove these characters, ensuring clean data.
string_with_whitespace = " Hello World "
trimmed_string = string_with_whitespace.strip() # trimmed_string will be "Hello World"
Advanced String Operations and Techniques
Beyond the basic manipulations, more advanced techniques exist to deal with complex string processing tasks:
Regular Expressions: Powerful Pattern Matching
Regular expressions (regex or regexp) are powerful tools for pattern matching within strings. They provide a concise and flexible way to search for and manipulate strings based on complex patterns. Languages offer libraries to work with regular expressions, enabling tasks like data extraction, validation, and text transformation.
String Formatting: Creating Readable Output
Formatting strings is crucial for generating readable output, especially when incorporating variables or data into strings. Many languages provide sophisticated formatting options using placeholders or f-strings (formatted string literals in Python).
name = "Alice"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
Applications of Strings: A Wide-Ranging Impact
The applications of strings span numerous domains:
1. Web Development: The Foundation of Websites
Strings are fundamental to web development. HTML, CSS, and JavaScript heavily rely on strings to represent content, styles, and behavior on websites. Web servers process strings to handle requests and generate responses.
2. Data Science and Analysis: Working with Textual Data
In data science, much data is textual. Strings are crucial for cleaning, processing, and analyzing this data, enabling tasks like sentiment analysis, topic modeling, and text classification.
3. Natural Language Processing (NLP): Understanding Human Language
NLP aims to enable computers to understand and interact with human language. Strings are the core data structure in NLP, enabling tasks like machine translation, chatbot development, and text summarization.
4. Software Development: User Interaction and Data Storage
Strings form the basis of much of the user interaction in software applications. Error messages, user input, and configuration settings are all represented as strings. Databases also store significant amounts of data as strings.
Optimization Strategies for Efficient String Processing
Efficient string processing is crucial, particularly when dealing with large datasets or performance-critical applications.
1. String Immutability: Understanding Its Implications
In many languages (like Python and Java), strings are immutable, meaning they cannot be changed after creation. Operations that appear to modify strings actually create new strings. This has implications for memory management and performance. Consider using mutable data structures like lists of characters when significant modifications are expected.
2. Algorithmic Efficiency: Choosing the Right Approach
The efficiency of string operations depends on the algorithms used. For example, searching for a substring can be done using naive string searching or more sophisticated algorithms like the Knuth-Morris-Pratt (KMP) algorithm, which offers improved performance for large strings.
3. Memory Management: Avoiding Unnecessary Copies
Creating unnecessary string copies can consume significant memory and reduce performance. Techniques like string concatenation using StringBuilder (in Java) or join()
(in Python) can improve efficiency by minimizing the number of string copies.
4. Data Structures: Selecting Appropriate Data Structures
The choice of data structure can impact string processing efficiency. Hash tables or tries can offer improved performance for certain operations, like searching or prefix matching.
Conclusion: The Enduring Significance of Strings
In conclusion, a string is a fundamental data structure representing a sequence of characters. Its importance in computing is undeniable, serving as the backbone for countless applications, from basic text manipulation to sophisticated natural language processing. Understanding string manipulation techniques, optimization strategies, and their wide-ranging applications is essential for any programmer or data scientist aiming to build efficient and robust software. The seemingly simple concept of a sequence of characters unlocks a world of possibilities in the digital realm. Mastering the art of string manipulation is a key skill for success in the ever-evolving world of computer science.
Latest Posts
Related Post
Thank you for visiting our website which covers about A String Is A Sequence Of ____. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.