6.9.5: Handling Input Exceptions: Restaurant Max Occupancy Tracker.
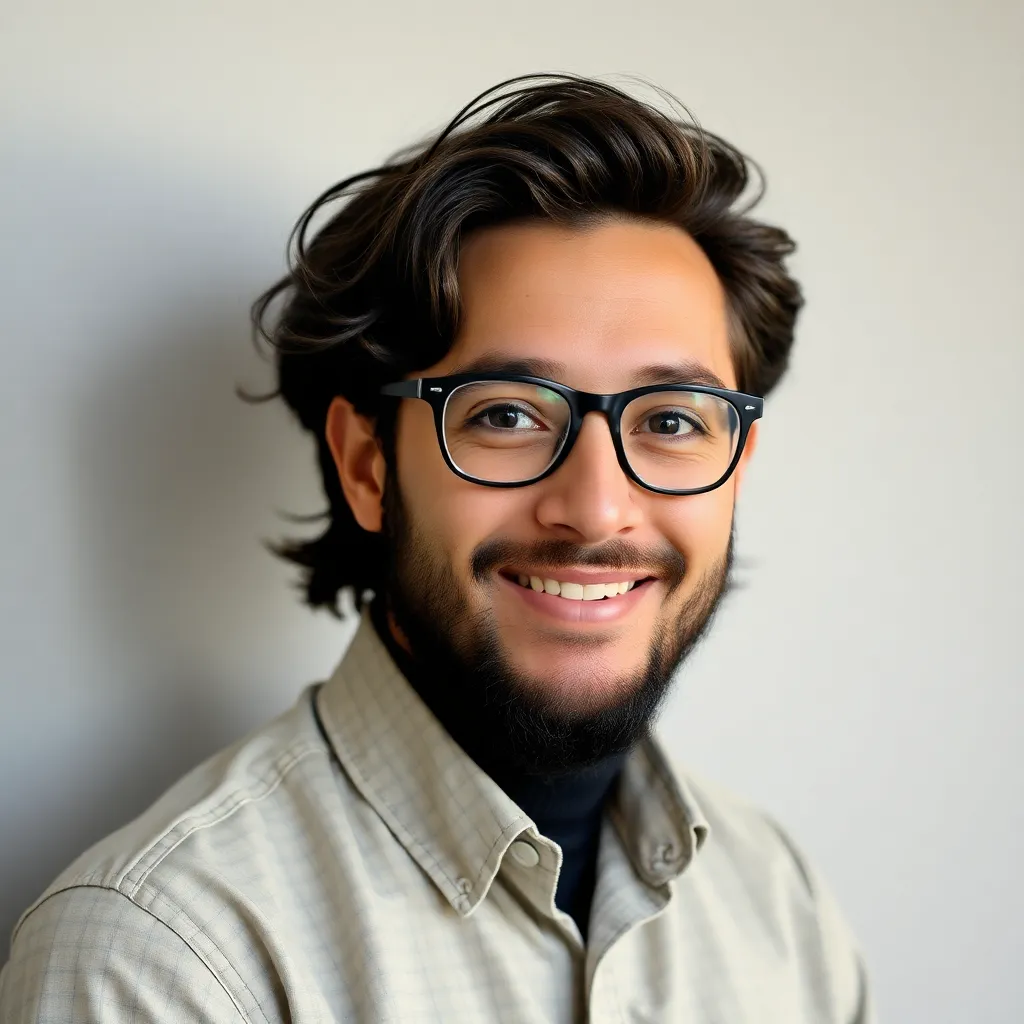
Holbox
May 08, 2025 · 5 min read
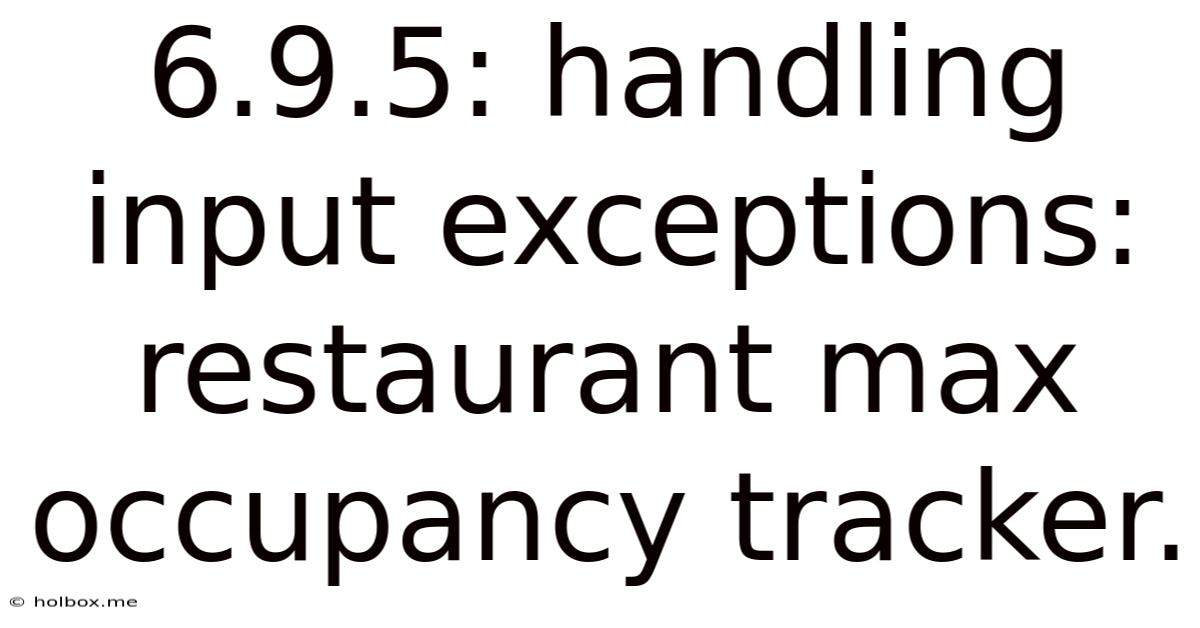
Table of Contents
- 6.9.5: Handling Input Exceptions: Restaurant Max Occupancy Tracker.
- Table of Contents
- 6.9.5: Handling Input Exceptions: Restaurant Max Occupancy Tracker
- Understanding the Problem: Invalid Input in a Restaurant Occupancy Tracker
- Implementing Robust Exception Handling: A Python Example
- Beyond Basic Exception Handling: Advanced Techniques
- 1. Logging Exceptions:
- 2. Custom Exceptions:
- 3. Input Sanitization:
- 4. Context Managers (with statement):
- 5. Testing and Unit Tests:
- Conclusion: Building a Reliable Restaurant Occupancy Tracker
- Latest Posts
- Latest Posts
- Related Post
6.9.5: Handling Input Exceptions: Restaurant Max Occupancy Tracker
This article delves into the crucial aspect of exception handling within the context of a restaurant maximum occupancy tracker, specifically focusing on error management related to user input. We'll explore how robust error handling enhances the user experience and prevents application crashes, building a reliable and user-friendly system. The example will use Python, but the principles are applicable to other programming languages.
Understanding the Problem: Invalid Input in a Restaurant Occupancy Tracker
A restaurant occupancy tracker needs to manage the number of patrons entering and leaving to ensure it stays within legal limits and maintains safety standards. This application frequently interacts with users, either through direct input or through integrated systems like a door counter. However, user input is notoriously unpredictable. Imagine these scenarios:
- Non-numeric input: A user accidentally types "ten" instead of 10, or enters a letter.
- Negative input: A user mistakenly inputs a negative number representing people leaving.
- Input exceeding capacity: A user tries to register more people than the restaurant's maximum capacity.
- No input: A user fails to enter any data when prompted.
These situations can cause the application to crash or produce incorrect results, leading to operational issues and a frustrating user experience. Effective exception handling is vital to prevent this.
Implementing Robust Exception Handling: A Python Example
Let's build a Python-based restaurant occupancy tracker with comprehensive exception handling. The code will focus on the core input validation and error handling aspects:
def track_occupancy(max_capacity):
"""Tracks restaurant occupancy, handling potential input errors."""
current_occupancy = 0
while True:
try:
action = input("Enter action (+ for entry, - for exit, or 'q' to quit): ")
if action.lower() == 'q':
break
if action not in ('+', '-'):
raise ValueError("Invalid action. Please enter '+' or '-' or 'q'.")
number_patrons = int(input("Enter number of patrons: "))
if number_patrons <= 0:
raise ValueError("Number of patrons must be a positive integer.")
if action == '+':
current_occupancy += number_patrons
if current_occupancy > max_capacity:
raise OverflowError("Maximum occupancy exceeded!")
elif action == '-':
current_occupancy -= number_patrons
if current_occupancy < 0:
raise ValueError("Occupancy cannot be negative.")
print(f"Current occupancy: {current_occupancy}/{max_capacity}")
except ValueError as e:
print(f"Error: {e}")
except OverflowError as e:
print(f"Error: {e}")
except Exception as e: # Catching other potential exceptions
print(f"An unexpected error occurred: {e}")
# Consider logging the error here for debugging purposes
print("Occupancy tracking ended.")
if __name__ == "__main__":
max_capacity = 0
while True:
try:
max_capacity = int(input("Enter the maximum capacity of the restaurant: "))
if max_capacity <=0:
raise ValueError("Maximum capacity must be a positive integer")
break
except ValueError as e:
print(f"Error: {e}")
track_occupancy(max_capacity)
This code demonstrates several key exception-handling techniques:
try-except
blocks: The core logic is encased intry-except
blocks to catch potential errors gracefully.- Specific exception types: Different exception types (
ValueError
,OverflowError
,Exception
) are caught, allowing for tailored error messages. - User-friendly error messages: Instead of cryptic error messages, informative messages guide the user on how to correct their input.
- Input validation: The code validates input to ensure it's within acceptable bounds (positive integers, valid actions).
- Handling general exceptions: The final
except Exception
block acts as a safety net for unexpected errors. This is crucial for production systems, allowing you to capture unanticipated problems. In a real-world scenario, logging details of this exception would be beneficial for debugging.
Beyond Basic Exception Handling: Advanced Techniques
The previous example provides a solid foundation. Let's explore more advanced techniques to make the occupancy tracker even more robust:
1. Logging Exceptions:
For debugging and maintenance, logging exceptions is essential. Instead of just printing error messages to the console, you should record the error details in a log file. Python's logging
module is ideal for this:
import logging
# Configure logging
logging.basicConfig(filename='occupancy_tracker.log', level=logging.ERROR,
format='%(asctime)s - %(levelname)s - %(message)s')
# ... (rest of the code remains the same, but add logging within the except blocks)
except ValueError as e:
logging.error(f"ValueError: {e}")
print(f"Error: {e}")
except OverflowError as e:
logging.error(f"OverflowError: {e}")
print(f"Error: {e}")
except Exception as e:
logging.exception(f"An unexpected error occurred: {e}") # Log the full traceback
print(f"An unexpected error occurred: {e}")
2. Custom Exceptions:
For improved clarity and maintainability, create custom exception classes. This allows you to define specific exception types that are relevant to the application's domain:
class OccupancyExceededError(Exception):
pass
class InvalidActionError(Exception):
pass
# ... (modified code using custom exceptions)
if action == '+':
current_occupancy += number_patrons
if current_occupancy > max_capacity:
raise OccupancyExceededError("Maximum occupancy exceeded!")
elif action == '-':
#...
except OccupancyExceededError as e:
logging.error(f"OccupancyExceededError: {e}")
print(f"Error: {e}")
except InvalidActionError as e:
logging.error(f"InvalidActionError: {e}")
print(f"Error: {e}")
#...
3. Input Sanitization:
While exception handling catches errors, preventing them in the first place is better. Input sanitization involves cleaning and validating user input before processing. For example, you might use regular expressions to ensure the input is in the expected format:
import re
# ... (inside the try block)
number_patrons_str = input("Enter number of patrons: ")
if not re.match(r"^\d+$", number_patrons_str):
raise ValueError("Invalid input. Please enter a positive integer.")
number_patrons = int(number_patrons_str)
#...
4. Context Managers (with
statement):
For tasks that require resource cleanup (like file handling or database connections), use context managers with the with
statement. This ensures resources are properly released, even if exceptions occur:
5. Testing and Unit Tests:
Thorough testing is vital for robust error handling. Write unit tests to cover various scenarios, including those that should trigger exceptions. Frameworks like pytest
or unittest
can simplify this process. Test cases should include:
- Testing with valid inputs.
- Testing with invalid inputs (non-numeric, negative, exceeding capacity).
- Testing with edge cases (zero input, maximum capacity input).
- Testing the exception handling mechanisms themselves (do the right exceptions get raised and handled appropriately?).
Conclusion: Building a Reliable Restaurant Occupancy Tracker
Building a robust restaurant occupancy tracker requires diligent attention to exception handling. By implementing these techniques, you create a system that's not only functional but also user-friendly and resilient to unexpected inputs. Remember, comprehensive error handling is a critical aspect of software development, contributing significantly to the reliability and maintainability of any application. By combining advanced exception handling with thorough testing, you ensure that your restaurant occupancy tracker operates smoothly and reliably, improving both the customer and staff experience.
Latest Posts
Latest Posts
-
What Is 71 Inches In Feet
May 19, 2025
-
How Many Gallons Is 5 Cups
May 19, 2025
-
How Many Feet Is 75 Meters
May 19, 2025
-
What Is 29 Inches In Cm
May 19, 2025
-
How Many Kilos Is 170 Pounds
May 19, 2025
Related Post
Thank you for visiting our website which covers about 6.9.5: Handling Input Exceptions: Restaurant Max Occupancy Tracker. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.