6.2.9 Find Index Of A String
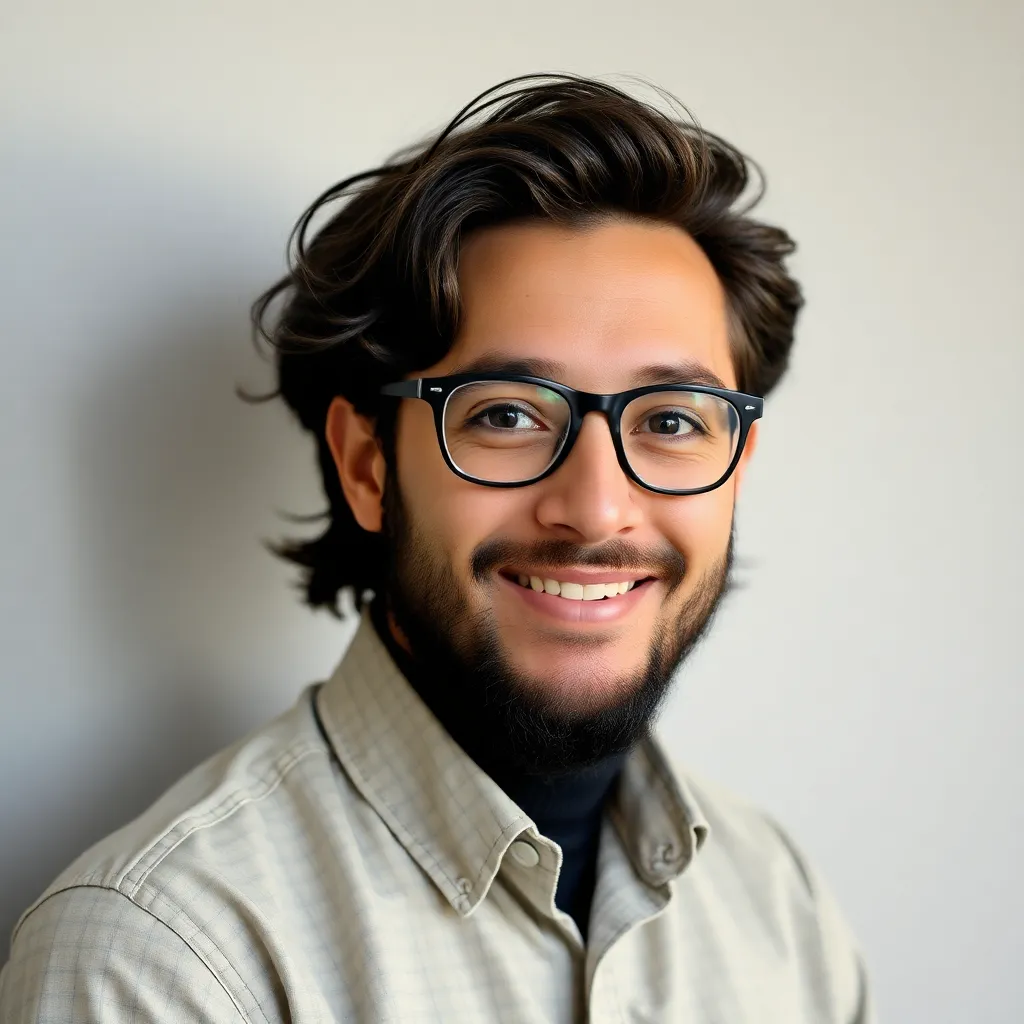
Holbox
Apr 01, 2025 · 5 min read
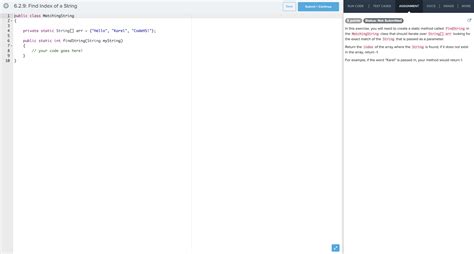
Table of Contents
- 6.2.9 Find Index Of A String
- Table of Contents
- 6.2.9 Finding the Index of a String: A Comprehensive Guide
- Understanding the Problem: String Indexing
- Method 1: Using Built-in String Functions (Python, JavaScript, and More)
- Python's find() and index() Methods
- JavaScript's indexOf() and lastIndexOf() Methods
- Other Languages
- Method 2: Manual Search using Loops (for educational purposes)
- Method 3: Regular Expressions
- Method 4: Handling Overlapping Substrings
- Method 5: Case-Insensitive Search
- Choosing the Right Method
- Error Handling and Robustness
- Performance Considerations
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
6.2.9 Finding the Index of a String: A Comprehensive Guide
Finding the index of a substring within a larger string is a fundamental task in many programming applications. Whether you're parsing log files, analyzing text data, or manipulating strings in a web application, understanding how to efficiently locate a substring's position is crucial. This comprehensive guide will delve into various methods for finding the index of a string, exploring their efficiency, limitations, and practical applications. We'll cover different programming languages and approaches, offering a robust understanding of this essential string manipulation technique.
Understanding the Problem: String Indexing
Before diving into specific methods, let's clarify the problem. We're looking to determine the starting position (index) of a target substring within a larger string. Indexes typically start at 0, meaning the first character of the string is at index 0, the second at index 1, and so on. If the substring isn't found, we often return a value indicating this, such as -1 or null.
Method 1: Using Built-in String Functions (Python, JavaScript, and More)
Most programming languages offer built-in functions specifically designed for finding substrings. These are usually the most straightforward and efficient approaches for common use cases.
Python's find()
and index()
Methods
Python provides two key methods: find()
and index()
. Both locate the first occurrence of a substring. The crucial difference lies in their handling of non-existent substrings:
find()
: Returns -1 if the substring is not found.index()
: Raises aValueError
exception if the substring is not found.
string = "This is a sample string."
substring = "sample"
index = string.find(substring) # index will be 10
print(f"Index using find(): {index}")
try:
index = string.index(substring) # index will be 10
print(f"Index using index(): {index}")
except ValueError:
print("Substring not found using index()")
substring2 = "missing"
index = string.find(substring2) # index will be -1
print(f"Index using find(): {index}")
try:
index = string.index(substring2)
print(f"Index using index(): {index}")
except ValueError:
print("Substring not found using index()")
JavaScript's indexOf()
and lastIndexOf()
Methods
JavaScript offers indexOf()
and lastIndexOf()
. indexOf()
finds the first occurrence, while lastIndexOf()
finds the last occurrence. Both return -1 if the substring is not found.
let string = "This is a sample string.";
let substring = "sample";
let index = string.indexOf(substring); // index will be 10
console.log(`Index using indexOf(): ${index}`);
let lastIndex = string.lastIndexOf(substring); // index will be 10 (only one occurrence)
console.log(`Last Index using lastIndexOf(): ${lastIndex}`);
let substring2 = "missing";
index = string.indexOf(substring2); // index will be -1
console.log(`Index using indexOf(): ${index}`);
Other Languages
Similar built-in functions exist in most other popular languages like Java (indexOf()
), C# (IndexOf()
), PHP (strpos()
), and many more. Consult your language's documentation for specifics.
Method 2: Manual Search using Loops (for educational purposes)
While built-in functions are generally preferred for efficiency, understanding the underlying logic can be valuable. A manual search involves iterating through the string and comparing substrings.
def find_substring_index(string, substring):
for i in range(len(string) - len(substring) + 1):
if string[i:i + len(substring)] == substring:
return i
return -1
string = "This is a sample string."
substring = "sample"
index = find_substring_index(string, substring) # index will be 10
print(f"Index using manual search: {index}")
This manual approach is less efficient than built-in functions, especially for large strings, but it demonstrates the fundamental steps involved.
Method 3: Regular Expressions
Regular expressions (regex) provide a powerful and flexible way to search for patterns within strings. While potentially more complex to learn, they can handle more sophisticated search criteria beyond simple substring matching.
import re
string = "This is a sample string with multiple samples."
substring = "sample"
match = re.search(substring, string)
if match:
index = match.start()
print(f"Index using regex: {index}") # index will be 10
else:
print("Substring not found using regex")
#Finding all occurrences
matches = re.finditer(substring, string)
for match in matches:
print(f"Index of 'sample': {match.start()}") #Prints all indices
Regex offers features like case-insensitive searches, finding multiple occurrences, and matching more complex patterns. However, for simple substring searches, built-in functions are generally more efficient.
Method 4: Handling Overlapping Substrings
Some scenarios require finding overlapping occurrences of a substring. Built-in functions typically only find non-overlapping occurrences. A custom function is needed to handle overlapping cases.
def find_overlapping_substring_indices(string, substring):
indices = []
for i in range(len(string) - len(substring) + 1):
if string[i:i + len(substring)] == substring:
indices.append(i)
return indices
string = "abababa"
substring = "aba"
indices = find_overlapping_substring_indices(string,substring)
print(f"Indices of overlapping substrings: {indices}") # Output: [0, 2, 4]
This function iterates and identifies all occurrences, even if they overlap.
Method 5: Case-Insensitive Search
Case-insensitive searches are often required. While some built-in functions offer options, you can also achieve this using string manipulation or regex.
string = "This Is A Sample String."
substring = "sample"
# Using lower() for case-insensitive search
index = string.lower().find(substring.lower())
print(f"Case-insensitive index: {index}") # index will be 10
#Using regex for case insensitive search
import re
match = re.search(substring, string, re.IGNORECASE)
if match:
index = match.start()
print(f"Case-insensitive index using regex: {index}")
Choosing the Right Method
The optimal method depends on your specific needs:
- For simple, single-occurrence searches, built-in functions are the most efficient and straightforward.
- For overlapping substring searches or more complex pattern matching, regular expressions are powerful but may require more learning.
- The manual looping method is mainly useful for educational purposes to understand the underlying algorithm.
Error Handling and Robustness
Always consider error handling. Check for potential issues like null or empty strings, and handle exceptions appropriately (e.g., using try-except
blocks in Python). Validate your input data to prevent unexpected behavior.
Performance Considerations
For extremely large strings, consider optimizing your approach. Advanced algorithms like the Boyer-Moore algorithm offer significantly faster substring search in some cases. However, for most everyday applications, built-in functions will suffice.
Conclusion
Finding the index of a string is a common task with several effective solutions. Understanding the strengths and weaknesses of each method—built-in functions, manual searches, and regular expressions—allows you to choose the most appropriate approach for your specific situation. Remember to prioritize clear, concise code, and always incorporate robust error handling for a reliable and maintainable solution. This thorough understanding of string indexing is crucial for any programmer working with text processing or data manipulation.
Latest Posts
Latest Posts
-
When Working Well The Issue Management Process
Apr 04, 2025
-
Managerial Accounting Is Different From Financial Accounting In That
Apr 04, 2025
-
How Many Sig Figs In 10000
Apr 04, 2025
-
What Is The Objects Position At T 2s
Apr 04, 2025
-
A Shift To Corporate Ownership Of Ranches Led To
Apr 04, 2025
Related Post
Thank you for visiting our website which covers about 6.2.9 Find Index Of A String . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.