6.10.6: Handling Multiple Exceptions: Vending Machine Example.
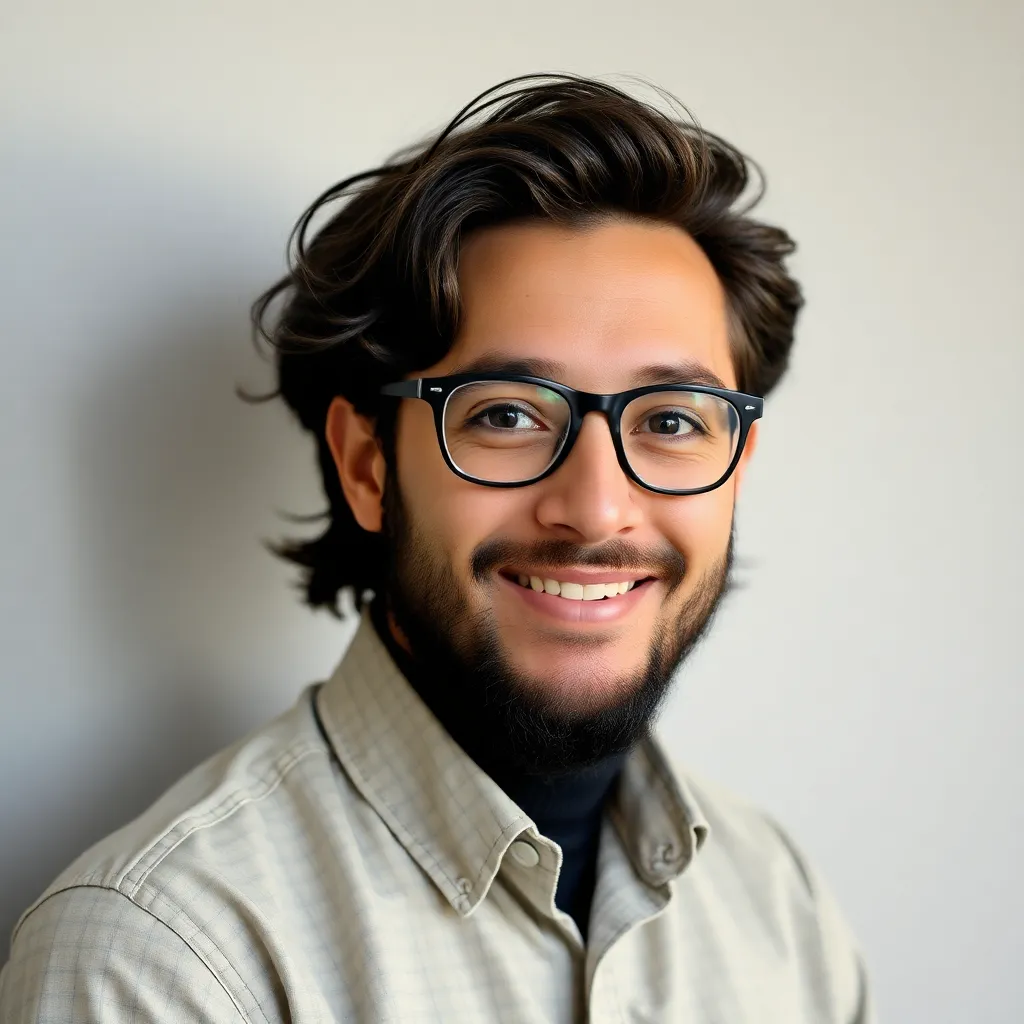
Holbox
Apr 04, 2025 · 5 min read

Table of Contents
- 6.10.6: Handling Multiple Exceptions: Vending Machine Example.
- Table of Contents
- 6.10.6: Handling Multiple Exceptions: A Vending Machine Example
- The Vending Machine Scenario
- Handling Exceptions with try-except Blocks
- Prioritizing Exception Handling
- Exception Chaining
- Using else and finally Blocks
- Logging Exceptions
- User-Friendly Error Messages
- Testing Exception Handling
- Advanced Techniques
- Conclusion
- Latest Posts
- Latest Posts
- Related Post
6.10.6: Handling Multiple Exceptions: A Vending Machine Example
Exception handling is a crucial aspect of robust software development. It allows your program to gracefully manage unexpected situations, preventing crashes and providing informative feedback to the user. While handling single exceptions is straightforward, real-world applications often require managing multiple, potentially interconnected exceptions. This article delves into the intricacies of handling multiple exceptions using a practical example: a vending machine program. We'll explore different strategies, best practices, and the importance of clear error messaging in building a reliable and user-friendly application.
The Vending Machine Scenario
Imagine a software system controlling a vending machine. Several things can go wrong:
- Insufficient Funds: The user might not insert enough money for the selected item.
- Item Out of Stock: The desired item might be sold out.
- Invalid Selection: The user might enter an invalid item code.
- Mechanical Failure: The vending machine's internal mechanisms (e.g., the dispensing mechanism) might malfunction.
- Network Issues: If the vending machine connects to a network for inventory updates or payment processing, network connectivity problems could arise.
Each of these scenarios represents a different type of exception. A well-designed vending machine program needs to handle each exception individually, providing appropriate feedback to the user and preventing the program from crashing.
Handling Exceptions with try-except
Blocks
Python's try-except
block is the fundamental mechanism for exception handling. To handle multiple exceptions, we can chain multiple except
blocks after a single try
block. Each except
block specifies the type of exception it handles.
class InsufficientFundsError(Exception):
pass
class ItemOutOfStockError(Exception):
pass
class InvalidSelectionError(Exception):
pass
class MechanicalFailureError(Exception):
pass
class NetworkError(Exception):
pass
def buy_item(item_code, amount_inserted):
# Simulate vending machine operations
items = {
"A1": {"price": 1.50, "stock": 3},
"B2": {"price": 2.00, "stock": 0},
"C3": {"price": 1.00, "stock": 5},
}
try:
item = items[item_code]
if amount_inserted < item["price"]:
raise InsufficientFundsError("Insufficient funds. Please insert more money.")
if item["stock"] == 0:
raise ItemOutOfStockError(f"Item {item_code} is out of stock.")
# Simulate dispensing and deduct stock
item["stock"] -= 1
print(f"Dispensing item {item_code}. Your change is: ${amount_inserted - item['price']:.2f}")
except InsufficientFundsError as e:
print(f"Error: {e}")
except ItemOutOfStockError as e:
print(f"Error: {e}")
except KeyError:
raise InvalidSelectionError("Invalid item code. Please try again.")
except MechanicalFailureError as e:
print(f"Error: Mechanical failure. Please contact support. Details: {e}")
except NetworkError as e:
print(f"Error: Network error. Please try again later. Details: {e}")
except Exception as e: #A general except block to catch unexpected errors.
print(f"An unexpected error occurred: {e}")
#Example Usage
buy_item("A1", 2.00) #Successful purchase
buy_item("A1", 0.50) #Insufficient Funds
buy_item("B2", 3.00) # Item out of stock
buy_item("D4", 1.00) # Invalid Selection
This improved code demonstrates the handling of multiple exceptions using separate except
blocks. Each exception type has its corresponding handler, providing specific error messages. The addition of custom exception classes improves readability and maintainability.
Prioritizing Exception Handling
The order of except
blocks matters. Python checks the exceptions in the order they are listed. If an exception matches multiple except
blocks, only the first matching block will be executed. It's generally recommended to put more specific exception handlers before more general ones (like the base Exception
class).
Exception Chaining
In some cases, one exception might cause another. For example, a network error could prevent the vending machine from updating its inventory, leading to an ItemOutOfStockError
even if the item is actually in stock. Python allows you to chain exceptions using the raise ... from ...
syntax:
try:
# Simulate network failure during inventory update
raise NetworkError("Network connection failed during inventory update.")
except NetworkError as e:
raise ItemOutOfStockError("Item temporarily out of stock due to network error.") from e
This explicitly shows the causal relationship between the NetworkError
and the ItemOutOfStockError
, making debugging easier.
Using else
and finally
Blocks
The try-except
block can include an optional else
block and a finally
block.
-
else
block: The code in theelse
block executes only if no exception occurs in thetry
block. This is useful for code that should run only when the operation is successful. -
finally
block: The code in thefinally
block always executes, regardless of whether an exception occurs or not. This is ideal for cleanup actions (like closing files or releasing resources).
try:
# some code that might raise an exception
pass
except Exception as e:
#handle the exception
pass
else:
# Code to execute if no exception occurred
pass
finally:
# Code to execute regardless of exceptions
pass
Logging Exceptions
For larger applications, logging exceptions is crucial for debugging and monitoring. Python's logging
module provides a powerful mechanism for recording exceptions and other events.
import logging
logging.basicConfig(filename='vending_machine.log', level=logging.ERROR,
format='%(asctime)s - %(levelname)s - %(message)s')
try:
# Your vending machine code here
pass
except Exception as e:
logging.exception("An error occurred:") # Logs the exception and traceback
This logs error messages to a file, making it easier to track issues over time.
User-Friendly Error Messages
Avoid cryptic error messages. Instead, provide informative and user-friendly messages that guide the user on how to resolve the problem. Consider different scenarios:
- Insufficient Funds: "Insufficient funds. Please insert $X more."
- Item Out of Stock: "Item [Item Code] is currently out of stock."
- Invalid Selection: "Invalid item code. Please enter a valid code from the list."
- Mechanical Failure: "We're experiencing a technical issue. Please contact support."
Testing Exception Handling
Thorough testing is essential to ensure your exception handling is robust. Use unit tests to simulate various scenarios (including error conditions) and verify that your program handles them correctly.
Advanced Techniques
Custom Exception Classes: Defining custom exception classes, as shown in the examples, provides a more structured and maintainable approach to handling exceptions, especially for large projects.
Context Managers (with
statement): The with
statement simplifies resource management, ensuring that resources are properly released even if exceptions occur. For example, when working with files or network connections, use with
statements to handle resource cleanup reliably.
Conclusion
Handling multiple exceptions is a critical part of building robust and reliable applications. By using the techniques described in this article — chained except
blocks, custom exception classes, informative error messages, logging, and rigorous testing — you can create software that gracefully handles unexpected situations and provides a positive user experience. The vending machine example serves as a practical illustration of how to apply these principles to a real-world scenario. Remember to prioritize clear error handling to build trust and ensure user satisfaction.
Latest Posts
Latest Posts
-
When Using A Shared File Platform It Is Important To
Apr 09, 2025
-
Identify A Characteristic Of Venture Capital Private Equity Supported Start Ups
Apr 09, 2025
-
Which Regression Equation Best Fits These Data
Apr 09, 2025
-
These Employee Assignments Show A Job Design Strategy Of
Apr 09, 2025
-
Acetylene Is Hydrogenated To Form Ethane
Apr 09, 2025
Related Post
Thank you for visiting our website which covers about 6.10.6: Handling Multiple Exceptions: Vending Machine Example. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.