4.17 Lab Mad Lib - Loops
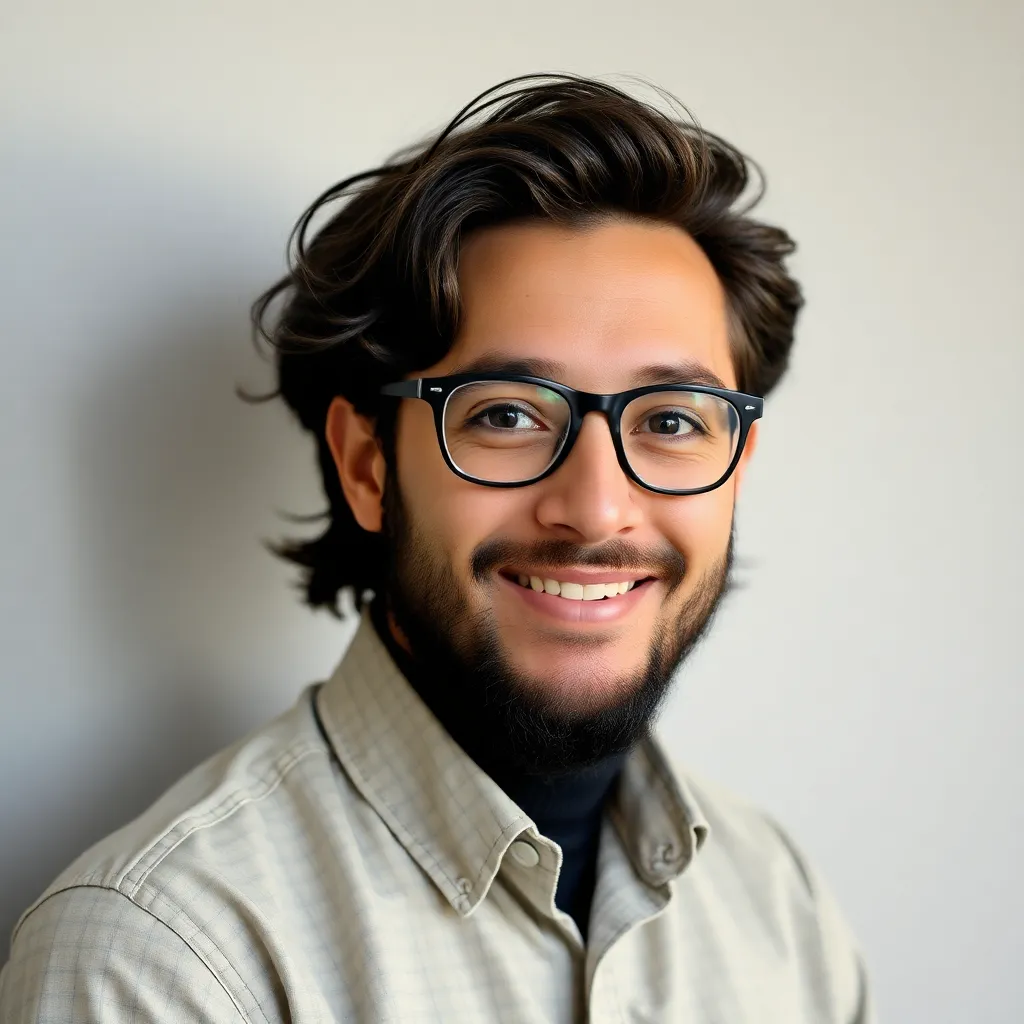
Holbox
May 10, 2025 · 5 min read
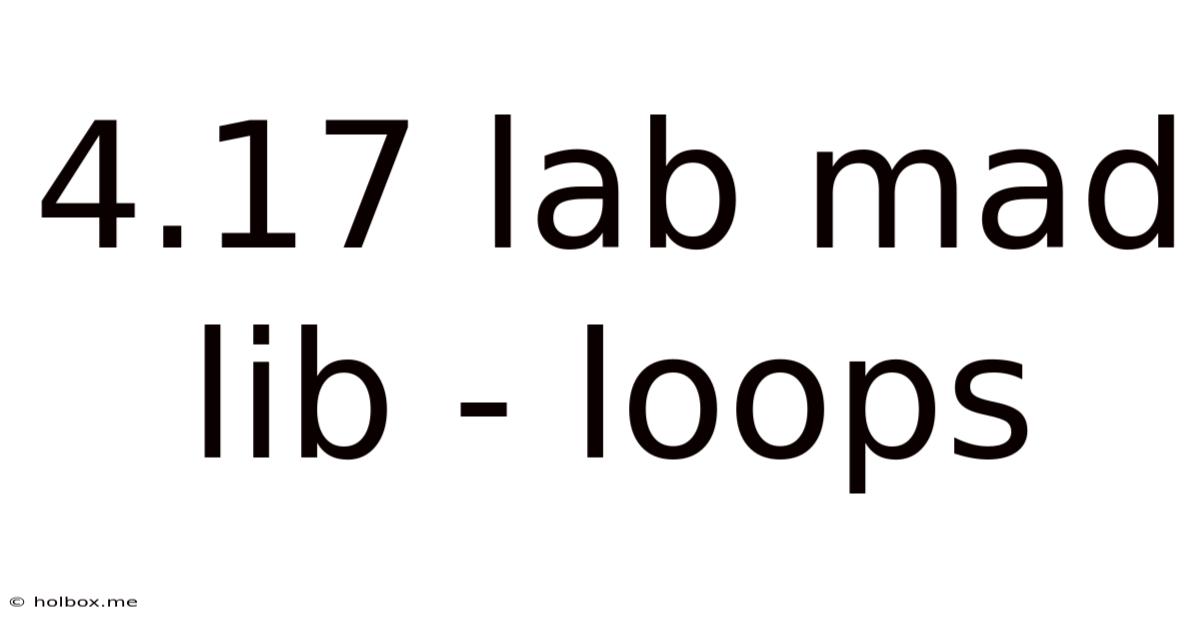
Table of Contents
- 4.17 Lab Mad Lib - Loops
- Table of Contents
- 4.17 Lab: Mad Libs with Loops – A Deep Dive into Python Programming
- Understanding the Mad Libs Game Concept
- Core Components: Lists and Loops in Python
- Implementing a for loop for Word Collection
- Employing a while loop for Enhanced User Interaction
- Building the Mad Libs Story Template
- Integrating Loops and String Formatting for Dynamic Story Generation
- Enhancing the Game with Advanced Features
- Handling Different Word Types with Nested Loops
- Implementing File I/O for Story Templates
- Adding a Score Tracking System with Loops
- Incorporating User Difficulty Levels
- Conclusion: Expanding Your Python Mad Libs Game
- Latest Posts
- Latest Posts
- Related Post
4.17 Lab: Mad Libs with Loops – A Deep Dive into Python Programming
This article delves into the creation of a Mad Libs game using Python, focusing specifically on the implementation of loops for enhanced user interaction and game functionality. We'll explore different loop types, error handling, and techniques for creating a more engaging and robust game experience. This comprehensive guide is perfect for beginners and intermediate Python programmers alike, offering practical examples and explanations to improve your coding skills. Let's get started!
Understanding the Mad Libs Game Concept
Mad Libs is a simple yet fun word game where one player prompts others for words of particular types (nouns, verbs, adjectives, etc.) without revealing the context. These words are then inserted into a pre-written story template, creating a hilarious and often nonsensical result. Our Python program will automate this process, leveraging the power of loops to streamline user input and story generation.
Core Components: Lists and Loops in Python
The heart of our Mad Libs program lies in the efficient use of lists and loops. Lists will store the user's input, while loops will manage the input process and story generation. We'll utilize both for
and while
loops, demonstrating their versatility in this application.
Implementing a for
loop for Word Collection
A for
loop is ideal for iterating through a predetermined number of inputs. In our Mad Libs game, this means looping a specific number of times to collect the required words from the user. Consider the following code snippet:
word_types = ["adjective", "noun", "verb", "adverb"]
words = []
for word_type in word_types:
word = input(f"Enter a {word_type}: ")
words.append(word)
print(words)
This code iterates through the word_types
list, prompting the user for each word type and storing the input in the words
list. The f-string
allows for dynamic string formatting, making the prompts clear and user-friendly.
Employing a while
loop for Enhanced User Interaction
A while
loop offers more control, allowing the program to continue prompting the user until a specific condition is met. This is particularly useful for error handling and providing a more robust user experience. For example, we can ensure the user enters valid input:
valid_input = False
while not valid_input:
try:
number_of_words = int(input("Enter the number of words you want to input: "))
if number_of_words > 0:
valid_input = True
else:
print("Please enter a positive number.")
except ValueError:
print("Invalid input. Please enter a number.")
print(f"You entered {number_of_words} words.")
This code uses a while
loop and a try-except
block to handle potential ValueError
exceptions if the user enters non-numeric input. It also ensures the user enters a positive number of words.
Building the Mad Libs Story Template
The story template is crucial. It's where the user-provided words will be inserted. We can represent this using a string with placeholders for the words. Here's an example:
story_template = """
The {adjective} {noun} {verb} {adverb} down the street.
It was a very {adjective} day.
"""
This template uses curly braces {}
as placeholders for the words the user will provide.
Integrating Loops and String Formatting for Dynamic Story Generation
Once we have the user's input and the story template, we can use string formatting and a loop to dynamically generate the final Mad Libs story. We can achieve this using f-strings
again or the str.format()
method. Here's an example using f-strings
:
words = ["silly", "monkey", "jumped", "quickly"]
story = f"""
The {words[0]} {words[1]} {words[2]} {words[3]} down the street.
It was a very {words[0]} day.
"""
print(story)
This code replaces the placeholders in the story_template
with the words from the words
list, creating the final Mad Libs story. We can adapt this to handle lists of any length, making the game more flexible.
Enhancing the Game with Advanced Features
To make our Mad Libs game more engaging, we can add several advanced features. These features can be incorporated using more sophisticated loop structures and conditional statements.
Handling Different Word Types with Nested Loops
Instead of a simple list of word types, we can use a dictionary to store different word types with multiple entries for each type. This allows the user to pick from options. We would need nested loops to manage the selection process.
word_types = {
"adjective": ["happy", "sad", "angry", "excited"],
"noun": ["cat", "dog", "bird", "tree"],
"verb": ["run", "jump", "fly", "swim"],
}
words = {}
for word_type, options in word_types.items():
print(f"Choose a {word_type}:")
for i, option in enumerate(options):
print(f"{i+1}. {option}")
while True:
try:
choice = int(input("Enter your choice: "))
if 1 <= choice <= len(options):
words[word_type] = options[choice - 1]
break
else:
print("Invalid choice. Please try again.")
except ValueError:
print("Invalid input. Please enter a number.")
# Generate story using words dictionary...
This example uses nested loops to present the user with choices and handles input validation effectively.
Implementing File I/O for Story Templates
Instead of hardcoding the story template, we can read it from a file. This makes the game more flexible, allowing for easy addition of new stories.
def load_story_from_file(filename):
try:
with open(filename, 'r') as f:
story_template = f.read()
return story_template
except FileNotFoundError:
print(f"Error: Story file '{filename}' not found.")
return None
# ... rest of the code ...
story_template = load_story_from_file("story.txt")
if story_template:
# ... process story_template ...
This code handles potential FileNotFoundError
exceptions gracefully.
Adding a Score Tracking System with Loops
We could introduce a scoring system to make the game more competitive. A loop could manage the accumulation of points based on correct word choices or story creativity.
Incorporating User Difficulty Levels
Different difficulty levels can be implemented. Easy levels may require fewer words, while harder levels might use more complex sentence structures and vocabulary. This could use conditional statements within a loop to control game difficulty.
Conclusion: Expanding Your Python Mad Libs Game
This expanded exploration of creating a Mad Libs game in Python demonstrates the power and versatility of loops in building interactive and engaging applications. By mastering loop control, error handling, and advanced features, you can create a robust and captivating game. Remember to always prioritize user experience and clear, well-commented code. The possibilities for expanding this game are numerous, limited only by your imagination and programming skills. Continue experimenting, and you'll significantly improve your Python programming abilities.
Latest Posts
Latest Posts
-
How Many Kilometers Are In 26 Miles
May 20, 2025
-
What Is 190 Cm In Inches
May 20, 2025
-
How Many Miles Is 32 Kilometers
May 20, 2025
-
86 10 Kg In Stone And Pounds
May 20, 2025
-
What Is 78 Kg In Stone
May 20, 2025
Related Post
Thank you for visiting our website which covers about 4.17 Lab Mad Lib - Loops . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.